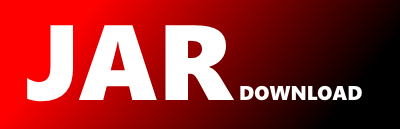
net.leanix.dropkit.util.ManifestUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leanix-dropkit Show documentation
Show all versions of leanix-dropkit Show documentation
Base functionality for leanIX dropwizard-based services
The newest version!
package net.leanix.dropkit.util;
import com.codahale.metrics.Gauge;
import com.codahale.metrics.Metric;
import com.codahale.metrics.MetricSet;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Enumeration;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.jar.Attributes;
import java.util.jar.Manifest;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class ManifestUtil {
private static final Logger LOG = LoggerFactory.getLogger(ManifestUtil.class);
private static final String META_INF_MANIFEST_MF = "META-INF/MANIFEST.MF";
/**
* Scan the complete classpath and tries to find the MANIFEST.MF file within a jar where the given name {@code bootJarName}
* is part of the jar file name. In case a MANIFEST.MF file is found, this is parsed and provided as a {@linkplain Manifest}
* optional.
*/
public Optional findManifestForJar(String bootJarName) {
try {
// find out, which jars contains a manifest
Enumeration resources = getClass().getClassLoader().getResources(META_INF_MANIFEST_MF);
List resouresList = new ArrayList<>();
while (resources.hasMoreElements()) {
URL nextElement = resources.nextElement();
resouresList.add(nextElement);
}
if (resouresList.isEmpty()) {
return Optional.empty();
}
// iterate from last to top
Collections.reverse(resouresList);
URL relevantUrl = resouresList.stream()
.peek(url -> LOG.debug("found: {}", url))
.filter(url -> url.toString().contains(bootJarName))
.findFirst()
.orElse(resouresList.get(0));
// parse metadata file
try (InputStream is = relevantUrl.openStream()) {
LOG.info("parsing manifest from url '{}'", relevantUrl);
return Optional.of(new Manifest(is));
}
} catch (IOException e) {
LOG.warn("failed to load resource '{}' for boot jar '{}'", META_INF_MANIFEST_MF, bootJarName, e);
}
return Optional.empty();
}
/**
* Creates a {@linkplain Gauge} metric used to show the title and version of a given microservice.
* Usage, eg:
*
* @param manifest A {@linkplain Manifest} objects which contains the information used for this metric.
*/
public static ManifestGauge createManifestGauge(Manifest manifest) {
return new ManifestGauge(manifest);
}
public static class ManifestGauge implements MetricSet {
private final Manifest manifest;
ManifestGauge(Manifest manifest) {
this.manifest = manifest;
}
@Override
public Map getMetrics() {
final Map gauges = new HashMap();
gauges.put("version", new Gauge() {
@Override
public String getValue() {
return readFromManifest("Implementation-Version");
}
});
gauges.put("title", new Gauge() {
@Override
public String getValue() {
return readFromManifest("Implementation-Title");
}
});
return Collections.unmodifiableMap(gauges);
}
protected String readFromManifest(String name) {
Attributes attributes = manifest.getMainAttributes();
String value = "---";
if (attributes != null) {
value = attributes.getValue(name);
}
return value;
}
}
}