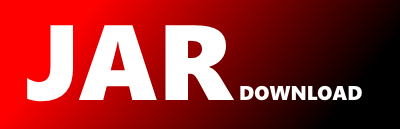
net.leanix.mtm.api.dropwizard.TemplateFactory Maven / Gradle / Ivy
package net.leanix.mtm.api.dropwizard;
import com.google.common.base.Charsets;
import com.google.common.io.Resources;
import com.google.inject.Singleton;
import java.io.IOException;
import java.net.URL;
import java.util.HashMap;
import java.util.Map;
import java.util.logging.Level;
import java.util.logging.Logger;
import net.leanix.mtm.api.models.NotificationTemplate;
/**
* Notification template factory.
*
* Works only in jars and in Unix file systems. Expects a subfolder for each channel.
*
*/
@Singleton
public class TemplateFactory
{
private final Map loadedTemplates = new HashMap();
private String basePath = "views/notifications/";
/**
* Sets the relative base path of the mustache templates.
*
* Defaults to "views/notifications/"
*
* @param basePath
*/
public void setBasePath(String basePath)
{
this.basePath = basePath;
}
/**
* Returns a notification template for an event and recipient.
*
* @param channel
* @param eventType
* @param recipientType
* @return
*/
public NotificationTemplate getTemplate(String channel, String eventType, String recipientType)
{
return getTemplate(channel, eventType.toLowerCase() + "." + recipientType.toLowerCase());
}
/**
* Returns a notification template with a name.
*
* @param channel
* @param name
* @return
*/
public NotificationTemplate getTemplate(String channel, String name)
{
String path = basePath + channel + "/"
+ name.toLowerCase()
+ ".mustache";
return createTemplate(channel, path);
}
private NotificationTemplate createTemplate(String channel, String path)
{
try
{
NotificationTemplate template = new NotificationTemplate();
template.setChannel(channel);
template.setContent(getTemplateString(path));
return template;
} catch (IOException ex)
{
Logger.getLogger(TemplateFactory.class.getName()).log(Level.SEVERE, null, ex);
return null;
}
}
/**
* Loads relative file locate in a jar.
*
* Works only with jars: http://stackoverflow.com/questions/574809/load-a-resource-contained-in-a-jar
*
* @param path
* @return
*/
private String getTemplateString(String path) throws IOException
{
if (loadedTemplates.containsKey(path))
return loadedTemplates.get(path);
URL url = ClassLoader.getSystemResource(path);
if (url == null)
{
throw new IllegalArgumentException("Cannot load template from " + path);
}
String template = Resources.toString(url, Charsets.UTF_8);
loadedTemplates.put(path, template);
return template;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy