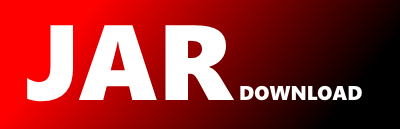
net.leanix.mtm.api.models.Notification Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leanix-mtm-sdk-java Show documentation
Show all versions of leanix-mtm-sdk-java Show documentation
SDK for Java to access leanIX MTM REST API
package net.leanix.mtm.api.models;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModelProperty;
import java.util.Date;
import java.util.Objects;
public class Notification {
private String id = null;
private String subject = null;
private String subjectAggregated = null;
public enum StatusEnum {
READ("READ"),
UNREAD("UNREAD");
private String value;
StatusEnum(String value) {
this.value = value;
}
@Override
@JsonValue
public String toString() {
return value;
}
}
private StatusEnum status = null;
private Date createdAt = null;
private String workspaceId = null;
private User recipient = null;
private String type = null;
private String templates = null;
private String payload = null;
public enum PriorityEnum {
HIGH("HIGH"),
MEDIUM("MEDIUM"),
LOW("LOW");
private String value;
PriorityEnum(String value) {
this.value = value;
}
@Override
@JsonValue
public String toString() {
return value;
}
}
private PriorityEnum priority = null;
public enum TransmissionStatusEnum {
UNSENT("UNSENT"),
SENT("SENT"),
RESENT("RESENT"),
FAILED("FAILED"),
REJECTED("REJECTED");
private String value;
TransmissionStatusEnum(String value) {
this.value = value;
}
@Override
@JsonValue
public String toString() {
return value;
}
}
private TransmissionStatusEnum transmissionStatus = null;
private Integer failCount = null;
private NotificationOrigin origin = null;
/**
**/
public Notification id(String id) {
this.id = id;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("id")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
/**
**/
public Notification subject(String subject) {
this.subject = subject;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("subject")
public String getSubject() {
return subject;
}
public void setSubject(String subject) {
this.subject = subject;
}
/**
**/
public Notification subjectAggregated(String subjectAggregated) {
this.subjectAggregated = subjectAggregated;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("subjectAggregated")
public String getSubjectAggregated() {
return subjectAggregated;
}
public void setSubjectAggregated(String subjectAggregated) {
this.subjectAggregated = subjectAggregated;
}
/**
**/
public Notification status(StatusEnum status) {
this.status = status;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("status")
public StatusEnum getStatus() {
return status;
}
public void setStatus(StatusEnum status) {
this.status = status;
}
/**
**/
public Notification createdAt(Date createdAt) {
this.createdAt = createdAt;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("createdAt")
public Date getCreatedAt() {
return createdAt;
}
public void setCreatedAt(Date createdAt) {
this.createdAt = createdAt;
}
/**
**/
public Notification workspaceId(String workspaceId) {
this.workspaceId = workspaceId;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("workspaceId")
public String getWorkspaceId() {
return workspaceId;
}
public void setWorkspaceId(String workspaceId) {
this.workspaceId = workspaceId;
}
/**
**/
public Notification recipient(User recipient) {
this.recipient = recipient;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("recipient")
public User getRecipient() {
return recipient;
}
public void setRecipient(User recipient) {
this.recipient = recipient;
}
/**
**/
public Notification type(String type) {
this.type = type;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("type")
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
/**
**/
public Notification templates(String templates) {
this.templates = templates;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("templates")
public String getTemplates() {
return templates;
}
public void setTemplates(String templates) {
this.templates = templates;
}
/**
**/
public Notification payload(String payload) {
this.payload = payload;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("payload")
public String getPayload() {
return payload;
}
public void setPayload(String payload) {
this.payload = payload;
}
/**
**/
public Notification priority(PriorityEnum priority) {
this.priority = priority;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("priority")
public PriorityEnum getPriority() {
return priority;
}
public void setPriority(PriorityEnum priority) {
this.priority = priority;
}
/**
**/
public Notification transmissionStatus(TransmissionStatusEnum transmissionStatus) {
this.transmissionStatus = transmissionStatus;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("transmissionStatus")
public TransmissionStatusEnum getTransmissionStatus() {
return transmissionStatus;
}
public void setTransmissionStatus(TransmissionStatusEnum transmissionStatus) {
this.transmissionStatus = transmissionStatus;
}
/**
**/
public Notification failCount(Integer failCount) {
this.failCount = failCount;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("failCount")
public Integer getFailCount() {
return failCount;
}
public void setFailCount(Integer failCount) {
this.failCount = failCount;
}
/**
**/
public Notification origin(NotificationOrigin origin) {
this.origin = origin;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("origin")
public NotificationOrigin getOrigin() {
return origin;
}
public void setOrigin(NotificationOrigin origin) {
this.origin = origin;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Notification notification = (Notification) o;
return Objects.equals(this.id, notification.id) &&
Objects.equals(this.subject, notification.subject) &&
Objects.equals(this.subjectAggregated, notification.subjectAggregated) &&
Objects.equals(this.status, notification.status) &&
Objects.equals(this.createdAt, notification.createdAt) &&
Objects.equals(this.workspaceId, notification.workspaceId) &&
Objects.equals(this.recipient, notification.recipient) &&
Objects.equals(this.type, notification.type) &&
Objects.equals(this.templates, notification.templates) &&
Objects.equals(this.payload, notification.payload) &&
Objects.equals(this.priority, notification.priority) &&
Objects.equals(this.transmissionStatus, notification.transmissionStatus) &&
Objects.equals(this.failCount, notification.failCount) &&
Objects.equals(this.origin, notification.origin);
}
@Override
public int hashCode() {
return Objects.hash(id, subject, subjectAggregated, status, createdAt, workspaceId, recipient, type, templates, payload, priority, transmissionStatus, failCount, origin);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Notification {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" subject: ").append(toIndentedString(subject)).append("\n");
sb.append(" subjectAggregated: ").append(toIndentedString(subjectAggregated)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" workspaceId: ").append(toIndentedString(workspaceId)).append("\n");
sb.append(" recipient: ").append(toIndentedString(recipient)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" templates: ").append(toIndentedString(templates)).append("\n");
sb.append(" payload: ").append(toIndentedString(payload)).append("\n");
sb.append(" priority: ").append(toIndentedString(priority)).append("\n");
sb.append(" transmissionStatus: ").append(toIndentedString(transmissionStatus)).append("\n");
sb.append(" failCount: ").append(toIndentedString(failCount)).append("\n");
sb.append(" origin: ").append(toIndentedString(origin)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy