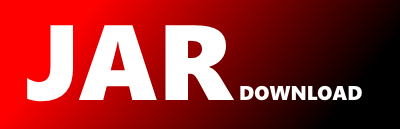
net.leanix.mtm.api.models.Event Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leanix-mtm-sdk-java Show documentation
Show all versions of leanix-mtm-sdk-java Show documentation
SDK for Java to access leanIX MTM REST API
package net.leanix.mtm.api.models;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import net.leanix.mtm.api.models.Account;
import net.leanix.mtm.api.models.Contract;
import net.leanix.mtm.api.models.Instance;
import net.leanix.mtm.api.models.Link;
import net.leanix.mtm.api.models.User;
import net.leanix.mtm.api.models.Workspace;
public class Event {
private String id = null;
public enum TypeEnum {
TEST_EVENT("TEST_EVENT"),
ACCOUNT_CREATE("ACCOUNT_CREATE"),
ACCOUNT_UPDATE("ACCOUNT_UPDATE"),
CONTRACT_CREATE("CONTRACT_CREATE"),
CONTRACT_UPDATE("CONTRACT_UPDATE"),
WORKSPACE_CREATE("WORKSPACE_CREATE"),
WORKSPACE_UPDATE("WORKSPACE_UPDATE"),
WORKSPACE_DELETE("WORKSPACE_DELETE"),
WORKSPACE_INITIALIZE("WORKSPACE_INITIALIZE"),
USER_CREATE("USER_CREATE"),
USER_UPDATE("USER_UPDATE"),
USER_DELETE("USER_DELETE"),
USER_WELCOME("USER_WELCOME"),
USER_LOGIN("USER_LOGIN"),
USER_ACCESS_WORKSPACE("USER_ACCESS_WORKSPACE"),
USER_PERMISSION_CREATE("USER_PERMISSION_CREATE"),
USER_PERMISSION_UPDATE("USER_PERMISSION_UPDATE"),
USER_ACTIVATE("USER_ACTIVATE"),
USER_INVITE("USER_INVITE"),
USER_INVITE_CONFIRM("USER_INVITE_CONFIRM"),
USER_INVITE_REJECT("USER_INVITE_REJECT"),
USER_INVITE_APPROVE("USER_INVITE_APPROVE"),
USER_INVITE_REMIND("USER_INVITE_REMIND"),
USER_PASSWORD_CREATE("USER_PASSWORD_CREATE"),
USER_PASSWORD_UPDATE("USER_PASSWORD_UPDATE"),
USER_PASSWORD_RESET("USER_PASSWORD_RESET");
private String value;
TypeEnum(String value) {
this.value = value;
}
@Override
@JsonValue
public String toString() {
return value;
}
}
private TypeEnum type = null;
private String application = null;
private String version = null;
public enum StatusEnum {
STARTED("STARTED"),
FINISHED("FINISHED");
private String value;
StatusEnum(String value) {
this.value = value;
}
@Override
@JsonValue
public String toString() {
return value;
}
}
private StatusEnum status = null;
private String payload = null;
private Date createdAt = null;
private User actor = null;
private Account account = null;
private User user = null;
private Workspace workspace = null;
private Contract contract = null;
private Instance instance = null;
private Date finishedAt = null;
private List links = new ArrayList();
/**
**/
public Event id(String id) {
this.id = id;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("id")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
/**
**/
public Event type(TypeEnum type) {
this.type = type;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "")
@JsonProperty("type")
public TypeEnum getType() {
return type;
}
public void setType(TypeEnum type) {
this.type = type;
}
/**
**/
public Event application(String application) {
this.application = application;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("application")
public String getApplication() {
return application;
}
public void setApplication(String application) {
this.application = application;
}
/**
**/
public Event version(String version) {
this.version = version;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("version")
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
/**
**/
public Event status(StatusEnum status) {
this.status = status;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("status")
public StatusEnum getStatus() {
return status;
}
public void setStatus(StatusEnum status) {
this.status = status;
}
/**
**/
public Event payload(String payload) {
this.payload = payload;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("payload")
public String getPayload() {
return payload;
}
public void setPayload(String payload) {
this.payload = payload;
}
/**
**/
public Event createdAt(Date createdAt) {
this.createdAt = createdAt;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("createdAt")
public Date getCreatedAt() {
return createdAt;
}
public void setCreatedAt(Date createdAt) {
this.createdAt = createdAt;
}
/**
**/
public Event actor(User actor) {
this.actor = actor;
return this;
}
@ApiModelProperty(example = "null", required = true, value = "")
@JsonProperty("actor")
public User getActor() {
return actor;
}
public void setActor(User actor) {
this.actor = actor;
}
/**
**/
public Event account(Account account) {
this.account = account;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("account")
public Account getAccount() {
return account;
}
public void setAccount(Account account) {
this.account = account;
}
/**
**/
public Event user(User user) {
this.user = user;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("user")
public User getUser() {
return user;
}
public void setUser(User user) {
this.user = user;
}
/**
**/
public Event workspace(Workspace workspace) {
this.workspace = workspace;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("workspace")
public Workspace getWorkspace() {
return workspace;
}
public void setWorkspace(Workspace workspace) {
this.workspace = workspace;
}
/**
**/
public Event contract(Contract contract) {
this.contract = contract;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("contract")
public Contract getContract() {
return contract;
}
public void setContract(Contract contract) {
this.contract = contract;
}
/**
**/
public Event instance(Instance instance) {
this.instance = instance;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("instance")
public Instance getInstance() {
return instance;
}
public void setInstance(Instance instance) {
this.instance = instance;
}
/**
**/
public Event finishedAt(Date finishedAt) {
this.finishedAt = finishedAt;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("finishedAt")
public Date getFinishedAt() {
return finishedAt;
}
public void setFinishedAt(Date finishedAt) {
this.finishedAt = finishedAt;
}
/**
**/
public Event links(List links) {
this.links = links;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("links")
public List getLinks() {
return links;
}
public void setLinks(List links) {
this.links = links;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Event event = (Event) o;
return Objects.equals(this.id, event.id) &&
Objects.equals(this.type, event.type) &&
Objects.equals(this.application, event.application) &&
Objects.equals(this.version, event.version) &&
Objects.equals(this.status, event.status) &&
Objects.equals(this.payload, event.payload) &&
Objects.equals(this.createdAt, event.createdAt) &&
Objects.equals(this.actor, event.actor) &&
Objects.equals(this.account, event.account) &&
Objects.equals(this.user, event.user) &&
Objects.equals(this.workspace, event.workspace) &&
Objects.equals(this.contract, event.contract) &&
Objects.equals(this.instance, event.instance) &&
Objects.equals(this.finishedAt, event.finishedAt) &&
Objects.equals(this.links, event.links);
}
@Override
public int hashCode() {
return Objects.hash(id, type, application, version, status, payload, createdAt, actor, account, user, workspace, contract, instance, finishedAt, links);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Event {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" application: ").append(toIndentedString(application)).append("\n");
sb.append(" version: ").append(toIndentedString(version)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" payload: ").append(toIndentedString(payload)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" actor: ").append(toIndentedString(actor)).append("\n");
sb.append(" account: ").append(toIndentedString(account)).append("\n");
sb.append(" user: ").append(toIndentedString(user)).append("\n");
sb.append(" workspace: ").append(toIndentedString(workspace)).append("\n");
sb.append(" contract: ").append(toIndentedString(contract)).append("\n");
sb.append(" instance: ").append(toIndentedString(instance)).append("\n");
sb.append(" finishedAt: ").append(toIndentedString(finishedAt)).append("\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy