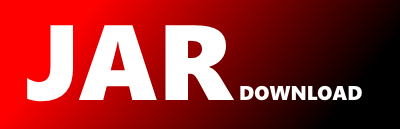
net.leanix.mtm.api.models.FeatureAccessRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leanix-mtm-sdk-java Show documentation
Show all versions of leanix-mtm-sdk-java Show documentation
SDK for Java to access leanIX MTM REST API
package net.leanix.mtm.api.models;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
import net.leanix.mtm.api.models.Feature;
import net.leanix.mtm.api.models.ModulePurchase;
public class FeatureAccessRequest {
private String edition = null;
private List modules = new ArrayList();
private List custom = new ArrayList();
private Integer value = null;
private String role = null;
/**
**/
public FeatureAccessRequest edition(String edition) {
this.edition = edition;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("edition")
public String getEdition() {
return edition;
}
public void setEdition(String edition) {
this.edition = edition;
}
/**
**/
public FeatureAccessRequest modules(List modules) {
this.modules = modules;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("modules")
public List getModules() {
return modules;
}
public void setModules(List modules) {
this.modules = modules;
}
/**
**/
public FeatureAccessRequest custom(List custom) {
this.custom = custom;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("custom")
public List getCustom() {
return custom;
}
public void setCustom(List custom) {
this.custom = custom;
}
/**
**/
public FeatureAccessRequest value(Integer value) {
this.value = value;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("value")
public Integer getValue() {
return value;
}
public void setValue(Integer value) {
this.value = value;
}
/**
**/
public FeatureAccessRequest role(String role) {
this.role = role;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("role")
public String getRole() {
return role;
}
public void setRole(String role) {
this.role = role;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
FeatureAccessRequest featureAccessRequest = (FeatureAccessRequest) o;
return Objects.equals(this.edition, featureAccessRequest.edition) &&
Objects.equals(this.modules, featureAccessRequest.modules) &&
Objects.equals(this.custom, featureAccessRequest.custom) &&
Objects.equals(this.value, featureAccessRequest.value) &&
Objects.equals(this.role, featureAccessRequest.role);
}
@Override
public int hashCode() {
return Objects.hash(edition, modules, custom, value, role);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class FeatureAccessRequest {\n");
sb.append(" edition: ").append(toIndentedString(edition)).append("\n");
sb.append(" modules: ").append(toIndentedString(modules)).append("\n");
sb.append(" custom: ").append(toIndentedString(custom)).append("\n");
sb.append(" value: ").append(toIndentedString(value)).append("\n");
sb.append(" role: ").append(toIndentedString(role)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy