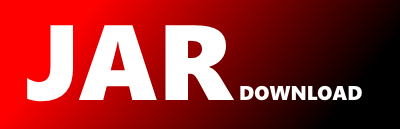
net.leanix.mtm.api.models.NotificationBatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leanix-mtm-sdk-java Show documentation
Show all versions of leanix-mtm-sdk-java Show documentation
SDK for Java to access leanIX MTM REST API
package net.leanix.mtm.api.models;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
import net.leanix.mtm.api.models.NotificationTemplate;
import net.leanix.mtm.api.models.User;
public class NotificationBatch {
private List recipients = new ArrayList();
private String subject = null;
private String subjectAggregated = null;
private String workspaceId = null;
private String type = null;
private List templates = new ArrayList();
private String payload = null;
public enum PriorityEnum {
HIGH("HIGH"),
MEDIUM("MEDIUM"),
LOW("LOW");
private String value;
PriorityEnum(String value) {
this.value = value;
}
@Override
@JsonValue
public String toString() {
return value;
}
}
private PriorityEnum priority = null;
public enum RulesEnum {
CONTACTS("ACCEPT_CONTACTS"),
INVITED("ACCEPT_INVITED");
private String value;
RulesEnum(String value) {
this.value = value;
}
@Override
@JsonValue
public String toString() {
return value;
}
}
private List rules = new ArrayList();
/**
**/
public NotificationBatch recipients(List recipients) {
this.recipients = recipients;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("recipients")
public List getRecipients() {
return recipients;
}
public void setRecipients(List recipients) {
this.recipients = recipients;
}
/**
**/
public NotificationBatch subject(String subject) {
this.subject = subject;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("subject")
public String getSubject() {
return subject;
}
public void setSubject(String subject) {
this.subject = subject;
}
/**
**/
public NotificationBatch subjectAggregated(String subjectAggregated) {
this.subjectAggregated = subjectAggregated;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("subjectAggregated")
public String getSubjectAggregated() {
return subjectAggregated;
}
public void setSubjectAggregated(String subjectAggregated) {
this.subjectAggregated = subjectAggregated;
}
/**
**/
public NotificationBatch workspaceId(String workspaceId) {
this.workspaceId = workspaceId;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("workspaceId")
public String getWorkspaceId() {
return workspaceId;
}
public void setWorkspaceId(String workspaceId) {
this.workspaceId = workspaceId;
}
/**
**/
public NotificationBatch type(String type) {
this.type = type;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("type")
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
/**
**/
public NotificationBatch templates(List templates) {
this.templates = templates;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("templates")
public List getTemplates() {
return templates;
}
public void setTemplates(List templates) {
this.templates = templates;
}
/**
**/
public NotificationBatch payload(String payload) {
this.payload = payload;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("payload")
public String getPayload() {
return payload;
}
public void setPayload(String payload) {
this.payload = payload;
}
/**
**/
public NotificationBatch priority(PriorityEnum priority) {
this.priority = priority;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("priority")
public PriorityEnum getPriority() {
return priority;
}
public void setPriority(PriorityEnum priority) {
this.priority = priority;
}
/**
**/
public NotificationBatch rules(List rules) {
this.rules = rules;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("rules")
public List getRules() {
return rules;
}
public void setRules(List rules) {
this.rules = rules;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
NotificationBatch notificationBatch = (NotificationBatch) o;
return Objects.equals(this.recipients, notificationBatch.recipients) &&
Objects.equals(this.subject, notificationBatch.subject) &&
Objects.equals(this.subjectAggregated, notificationBatch.subjectAggregated) &&
Objects.equals(this.workspaceId, notificationBatch.workspaceId) &&
Objects.equals(this.type, notificationBatch.type) &&
Objects.equals(this.templates, notificationBatch.templates) &&
Objects.equals(this.payload, notificationBatch.payload) &&
Objects.equals(this.priority, notificationBatch.priority) &&
Objects.equals(this.rules, notificationBatch.rules);
}
@Override
public int hashCode() {
return Objects.hash(recipients, subject, subjectAggregated, workspaceId, type, templates, payload, priority, rules);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class NotificationBatch {\n");
sb.append(" recipients: ").append(toIndentedString(recipients)).append("\n");
sb.append(" subject: ").append(toIndentedString(subject)).append("\n");
sb.append(" subjectAggregated: ").append(toIndentedString(subjectAggregated)).append("\n");
sb.append(" workspaceId: ").append(toIndentedString(workspaceId)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" templates: ").append(toIndentedString(templates)).append("\n");
sb.append(" payload: ").append(toIndentedString(payload)).append("\n");
sb.append(" priority: ").append(toIndentedString(priority)).append("\n");
sb.append(" rules: ").append(toIndentedString(rules)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy