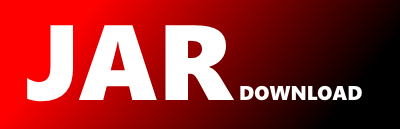
net.leanix.mtm.api.models.Impersonation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leanix-mtm-sdk-java Show documentation
Show all versions of leanix-mtm-sdk-java Show documentation
SDK for Java to access leanIX MTM REST API
/*
* LeanIX MTM REST API
* Multi-tenancy-manager for LeanIX. Manages accounts, contracts, users, workspaces, permissions, and more.
*
* OpenAPI spec version: 1.6.348
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package net.leanix.mtm.api.models;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import net.leanix.mtm.api.models.Link;
import net.leanix.mtm.api.models.User;
import net.leanix.mtm.api.models.Workspace;
/**
* Impersonation
*/
public class Impersonation {
@JsonProperty("id")
private String id = null;
@JsonProperty("impersonator")
private User impersonator = null;
@JsonProperty("workspace")
private Workspace workspace = null;
@JsonProperty("loginTime")
private OffsetDateTime loginTime = null;
@JsonProperty("logoutTime")
private OffsetDateTime logoutTime = null;
@JsonProperty("reason")
private String reason = null;
@JsonProperty("accessToken")
private String accessToken = null;
@JsonProperty("links")
private List links = null;
public Impersonation id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@ApiModelProperty(value = "")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public Impersonation impersonator(User impersonator) {
this.impersonator = impersonator;
return this;
}
/**
* Get impersonator
* @return impersonator
**/
@ApiModelProperty(required = true, value = "")
public User getImpersonator() {
return impersonator;
}
public void setImpersonator(User impersonator) {
this.impersonator = impersonator;
}
public Impersonation workspace(Workspace workspace) {
this.workspace = workspace;
return this;
}
/**
* Get workspace
* @return workspace
**/
@ApiModelProperty(required = true, value = "")
public Workspace getWorkspace() {
return workspace;
}
public void setWorkspace(Workspace workspace) {
this.workspace = workspace;
}
public Impersonation loginTime(OffsetDateTime loginTime) {
this.loginTime = loginTime;
return this;
}
/**
* Get loginTime
* @return loginTime
**/
@ApiModelProperty(required = true, value = "")
public OffsetDateTime getLoginTime() {
return loginTime;
}
public void setLoginTime(OffsetDateTime loginTime) {
this.loginTime = loginTime;
}
public Impersonation logoutTime(OffsetDateTime logoutTime) {
this.logoutTime = logoutTime;
return this;
}
/**
* Get logoutTime
* @return logoutTime
**/
@ApiModelProperty(value = "")
public OffsetDateTime getLogoutTime() {
return logoutTime;
}
public void setLogoutTime(OffsetDateTime logoutTime) {
this.logoutTime = logoutTime;
}
public Impersonation reason(String reason) {
this.reason = reason;
return this;
}
/**
* Get reason
* @return reason
**/
@ApiModelProperty(value = "")
public String getReason() {
return reason;
}
public void setReason(String reason) {
this.reason = reason;
}
public Impersonation accessToken(String accessToken) {
this.accessToken = accessToken;
return this;
}
/**
* Get accessToken
* @return accessToken
**/
@ApiModelProperty(value = "")
public String getAccessToken() {
return accessToken;
}
public void setAccessToken(String accessToken) {
this.accessToken = accessToken;
}
public Impersonation links(List links) {
this.links = links;
return this;
}
public Impersonation addLinksItem(Link linksItem) {
if (this.links == null) {
this.links = new ArrayList();
}
this.links.add(linksItem);
return this;
}
/**
* Get links
* @return links
**/
@ApiModelProperty(value = "")
public List getLinks() {
return links;
}
public void setLinks(List links) {
this.links = links;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Impersonation impersonation = (Impersonation) o;
return Objects.equals(this.id, impersonation.id) &&
Objects.equals(this.impersonator, impersonation.impersonator) &&
Objects.equals(this.workspace, impersonation.workspace) &&
Objects.equals(this.loginTime, impersonation.loginTime) &&
Objects.equals(this.logoutTime, impersonation.logoutTime) &&
Objects.equals(this.reason, impersonation.reason) &&
Objects.equals(this.accessToken, impersonation.accessToken) &&
Objects.equals(this.links, impersonation.links);
}
@Override
public int hashCode() {
return Objects.hash(id, impersonator, workspace, loginTime, logoutTime, reason, accessToken, links);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Impersonation {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" impersonator: ").append(toIndentedString(impersonator)).append("\n");
sb.append(" workspace: ").append(toIndentedString(workspace)).append("\n");
sb.append(" loginTime: ").append(toIndentedString(loginTime)).append("\n");
sb.append(" logoutTime: ").append(toIndentedString(logoutTime)).append("\n");
sb.append(" reason: ").append(toIndentedString(reason)).append("\n");
sb.append(" accessToken: ").append(toIndentedString(accessToken)).append("\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy