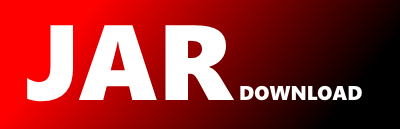
net.leanix.mtm.api.models.Permission Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leanix-mtm-sdk-java Show documentation
Show all versions of leanix-mtm-sdk-java Show documentation
SDK for Java to access leanIX MTM REST API
/*
* LeanIX MTM REST API
* Multi-tenancy-manager for LeanIX. Manages accounts, contracts, users, workspaces, permissions, and more.
*
* OpenAPI spec version: 1.6.348
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package net.leanix.mtm.api.models;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import net.leanix.mtm.api.models.Link;
import net.leanix.mtm.api.models.User;
import net.leanix.mtm.api.models.Workspace;
/**
* Permission
*/
public class Permission {
@JsonProperty("id")
private String id = null;
@JsonProperty("user")
private User user = null;
@JsonProperty("workspace")
private Workspace workspace = null;
@JsonProperty("workspaceId")
private String workspaceId = null;
/**
* Gets or Sets role
*/
public enum RoleEnum {
ADMIN("ADMIN"),
MEMBER("MEMBER"),
VIEWER("VIEWER"),
CONTACT("CONTACT"),
SYSTEM_READ("SYSTEM_READ"),
SYSTEM_WRITE("SYSTEM_WRITE"),
SYSTEM_AS_USER("SYSTEM_AS_USER"),
TRANSIENT("TRANSIENT");
private String value;
RoleEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static RoleEnum fromValue(String text) {
for (RoleEnum b : RoleEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
}
@JsonProperty("role")
private RoleEnum role = null;
/**
* Gets or Sets status
*/
public enum StatusEnum {
NOTINVITED("NOTINVITED"),
ACTIVE("ACTIVE"),
ARCHIVED("ARCHIVED"),
INVITED("INVITED"),
REQUESTED("REQUESTED"),
ANONYMIZED("ANONYMIZED");
private String value;
StatusEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static StatusEnum fromValue(String text) {
for (StatusEnum b : StatusEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
}
@JsonProperty("status")
private StatusEnum status = null;
@JsonProperty("lastLogin")
private OffsetDateTime lastLogin = null;
@JsonProperty("invitedByUser")
private User invitedByUser = null;
@JsonProperty("reviewedByUser")
private User reviewedByUser = null;
@JsonProperty("customerRoles")
private String customerRoles = null;
@JsonProperty("accessControlEntities")
private String accessControlEntities = null;
@JsonProperty("ignoreBlacklist")
private Boolean ignoreBlacklist = false;
@JsonProperty("links")
private List links = null;
@JsonProperty("count")
private Long count = null;
@JsonProperty("active")
private Boolean active = false;
public Permission id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@ApiModelProperty(value = "")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public Permission user(User user) {
this.user = user;
return this;
}
/**
* Get user
* @return user
**/
@ApiModelProperty(required = true, value = "")
public User getUser() {
return user;
}
public void setUser(User user) {
this.user = user;
}
public Permission workspace(Workspace workspace) {
this.workspace = workspace;
return this;
}
/**
* Get workspace
* @return workspace
**/
@ApiModelProperty(required = true, value = "")
public Workspace getWorkspace() {
return workspace;
}
public void setWorkspace(Workspace workspace) {
this.workspace = workspace;
}
public Permission workspaceId(String workspaceId) {
this.workspaceId = workspaceId;
return this;
}
/**
* Get workspaceId
* @return workspaceId
**/
@ApiModelProperty(value = "")
public String getWorkspaceId() {
return workspaceId;
}
public void setWorkspaceId(String workspaceId) {
this.workspaceId = workspaceId;
}
public Permission role(RoleEnum role) {
this.role = role;
return this;
}
/**
* Get role
* @return role
**/
@ApiModelProperty(required = true, value = "")
public RoleEnum getRole() {
return role;
}
public void setRole(RoleEnum role) {
this.role = role;
}
public Permission status(StatusEnum status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
**/
@ApiModelProperty(required = true, value = "")
public StatusEnum getStatus() {
return status;
}
public void setStatus(StatusEnum status) {
this.status = status;
}
public Permission lastLogin(OffsetDateTime lastLogin) {
this.lastLogin = lastLogin;
return this;
}
/**
* Get lastLogin
* @return lastLogin
**/
@ApiModelProperty(value = "")
public OffsetDateTime getLastLogin() {
return lastLogin;
}
public void setLastLogin(OffsetDateTime lastLogin) {
this.lastLogin = lastLogin;
}
public Permission invitedByUser(User invitedByUser) {
this.invitedByUser = invitedByUser;
return this;
}
/**
* Get invitedByUser
* @return invitedByUser
**/
@ApiModelProperty(value = "")
public User getInvitedByUser() {
return invitedByUser;
}
public void setInvitedByUser(User invitedByUser) {
this.invitedByUser = invitedByUser;
}
public Permission reviewedByUser(User reviewedByUser) {
this.reviewedByUser = reviewedByUser;
return this;
}
/**
* Get reviewedByUser
* @return reviewedByUser
**/
@ApiModelProperty(value = "")
public User getReviewedByUser() {
return reviewedByUser;
}
public void setReviewedByUser(User reviewedByUser) {
this.reviewedByUser = reviewedByUser;
}
public Permission customerRoles(String customerRoles) {
this.customerRoles = customerRoles;
return this;
}
/**
* Get customerRoles
* @return customerRoles
**/
@ApiModelProperty(value = "")
public String getCustomerRoles() {
return customerRoles;
}
public void setCustomerRoles(String customerRoles) {
this.customerRoles = customerRoles;
}
public Permission accessControlEntities(String accessControlEntities) {
this.accessControlEntities = accessControlEntities;
return this;
}
/**
* Get accessControlEntities
* @return accessControlEntities
**/
@ApiModelProperty(value = "")
public String getAccessControlEntities() {
return accessControlEntities;
}
public void setAccessControlEntities(String accessControlEntities) {
this.accessControlEntities = accessControlEntities;
}
public Permission ignoreBlacklist(Boolean ignoreBlacklist) {
this.ignoreBlacklist = ignoreBlacklist;
return this;
}
/**
* Get ignoreBlacklist
* @return ignoreBlacklist
**/
@ApiModelProperty(value = "")
public Boolean getIgnoreBlacklist() {
return ignoreBlacklist;
}
public void setIgnoreBlacklist(Boolean ignoreBlacklist) {
this.ignoreBlacklist = ignoreBlacklist;
}
public Permission links(List links) {
this.links = links;
return this;
}
public Permission addLinksItem(Link linksItem) {
if (this.links == null) {
this.links = new ArrayList();
}
this.links.add(linksItem);
return this;
}
/**
* Get links
* @return links
**/
@ApiModelProperty(value = "")
public List getLinks() {
return links;
}
public void setLinks(List links) {
this.links = links;
}
public Permission count(Long count) {
this.count = count;
return this;
}
/**
* Get count
* @return count
**/
@ApiModelProperty(value = "")
public Long getCount() {
return count;
}
public void setCount(Long count) {
this.count = count;
}
/**
* Get active
* @return active
**/
@ApiModelProperty(value = "")
public Boolean getActive() {
return active;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Permission permission = (Permission) o;
return Objects.equals(this.id, permission.id) &&
Objects.equals(this.user, permission.user) &&
Objects.equals(this.workspace, permission.workspace) &&
Objects.equals(this.workspaceId, permission.workspaceId) &&
Objects.equals(this.role, permission.role) &&
Objects.equals(this.status, permission.status) &&
Objects.equals(this.lastLogin, permission.lastLogin) &&
Objects.equals(this.invitedByUser, permission.invitedByUser) &&
Objects.equals(this.reviewedByUser, permission.reviewedByUser) &&
Objects.equals(this.customerRoles, permission.customerRoles) &&
Objects.equals(this.accessControlEntities, permission.accessControlEntities) &&
Objects.equals(this.ignoreBlacklist, permission.ignoreBlacklist) &&
Objects.equals(this.links, permission.links) &&
Objects.equals(this.count, permission.count) &&
Objects.equals(this.active, permission.active);
}
@Override
public int hashCode() {
return Objects.hash(id, user, workspace, workspaceId, role, status, lastLogin, invitedByUser, reviewedByUser, customerRoles, accessControlEntities, ignoreBlacklist, links, count, active);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Permission {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" user: ").append(toIndentedString(user)).append("\n");
sb.append(" workspace: ").append(toIndentedString(workspace)).append("\n");
sb.append(" workspaceId: ").append(toIndentedString(workspaceId)).append("\n");
sb.append(" role: ").append(toIndentedString(role)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" lastLogin: ").append(toIndentedString(lastLogin)).append("\n");
sb.append(" invitedByUser: ").append(toIndentedString(invitedByUser)).append("\n");
sb.append(" reviewedByUser: ").append(toIndentedString(reviewedByUser)).append("\n");
sb.append(" customerRoles: ").append(toIndentedString(customerRoles)).append("\n");
sb.append(" accessControlEntities: ").append(toIndentedString(accessControlEntities)).append("\n");
sb.append(" ignoreBlacklist: ").append(toIndentedString(ignoreBlacklist)).append("\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append(" count: ").append(toIndentedString(count)).append("\n");
sb.append(" active: ").append(toIndentedString(active)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy