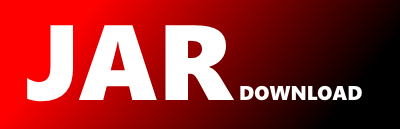
net.leanix.mtm.api.WorkspacesApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leanix-mtm-sdk-java Show documentation
Show all versions of leanix-mtm-sdk-java Show documentation
SDK for Java to access leanIX MTM REST API
package net.leanix.mtm.api;
import net.leanix.dropkit.apiclient.ApiException;
import net.leanix.dropkit.apiclient.ApiClient;
import net.leanix.dropkit.apiclient.Configuration;
import net.leanix.dropkit.apiclient.Pair;
import javax.ws.rs.core.GenericType;
import net.leanix.mtm.api.models.CustomFeatureListResponse;
import net.leanix.mtm.api.models.DownloadKeyResponse;
import net.leanix.mtm.api.models.EventListResponse;
import net.leanix.mtm.api.models.FeatureBundleResponse;
import net.leanix.mtm.api.models.ImpersonationListResponse;
import net.leanix.mtm.api.models.PermissionListResponse;
import net.leanix.mtm.api.models.PermissionResponse;
import net.leanix.mtm.api.models.SettingListResponse;
import net.leanix.mtm.api.models.StatsListResponse;
import java.util.UUID;
import net.leanix.mtm.api.models.UserListResponse;
import net.leanix.mtm.api.models.Workspace;
import net.leanix.mtm.api.models.WorkspaceListResponse;
import net.leanix.mtm.api.models.WorkspaceResponse;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class WorkspacesApi {
private ApiClient apiClient;
public WorkspacesApi() {
this(Configuration.getDefaultApiClient());
}
public WorkspacesApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* createWorkspace
* Creates a workspace
* @param body (required)
* @return WorkspaceResponse
* @throws ApiException if fails to make API call
*/
public WorkspaceResponse createWorkspace(Workspace body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling createWorkspace");
}
// create path and map variables
String localVarPath = "/workspaces";
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "token" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* deleteWorkspace
* Deletes a workspace
* @param id Workspace UUID (required)
* @return WorkspaceResponse
* @throws ApiException if fails to make API call
*/
public WorkspaceResponse deleteWorkspace(String id) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling deleteWorkspace");
}
// create path and map variables
String localVarPath = "/workspaces/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "token" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* getCustomFeatures
* Lists the workspace specific custom features
* @param id Workspace UUID (required)
* @return CustomFeatureListResponse
* @throws ApiException if fails to make API call
*/
public CustomFeatureListResponse getCustomFeatures(String id) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getCustomFeatures");
}
// create path and map variables
String localVarPath = "/workspaces/{id}/customFeatures"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "token" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* getEvents
* Retrieves all events for a workspace (date must be ISO 8601 formatted)
* @param id Workspace UUID (required)
* @param since (optional)
* @param page The page number to access (1 indexed, defaults to 1) (optional, default to 1)
* @param size The page size requested (defaults to 100, max 100) (optional, default to 100)
* @param sort Comma-separated list of sorting (optional) (optional)
* @param eventType Event type filter (optional) (optional)
* @return EventListResponse
* @throws ApiException if fails to make API call
*/
public EventListResponse getEvents(String id, String since, Integer page, Integer size, String sort, String eventType) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getEvents");
}
// create path and map variables
String localVarPath = "/workspaces/{id}/events"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "since", since));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "page", page));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "size", size));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "sort", sort));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "eventType", eventType));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "token" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* getFeatureBundle
* Retrieves a workspace feature bundle (setup settings)
* @param id Workspace UUID (required)
* @return FeatureBundleResponse
* @throws ApiException if fails to make API call
*/
public FeatureBundleResponse getFeatureBundle(String id) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getFeatureBundle");
}
// create path and map variables
String localVarPath = "/workspaces/{id}/featureBundle"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "token" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* getImpersonations
* Read support user impersonations of the workspace
* @param id The workspace to create a support user impersonation for. (required)
* @param page The page number to access (1 indexed, defaults to 1) (optional, default to 1)
* @param size The page size requested (defaults to 30, max 100) (optional, default to 30)
* @param sort Comma-separated list of sorting (optional) (optional)
* @return ImpersonationListResponse
* @throws ApiException if fails to make API call
*/
public ImpersonationListResponse getImpersonations(UUID id, Integer page, Integer size, String sort) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getImpersonations");
}
// create path and map variables
String localVarPath = "/workspaces/{id}/impersonations"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "page", page));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "size", size));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "sort", sort));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "token" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* getPermission
* Retrieves the permission with the given ID of the workspace
* @param id Workspace UUID (required)
* @param permissionId ID of the permission to get (required)
* @return PermissionResponse
* @throws ApiException if fails to make API call
*/
public PermissionResponse getPermission(String id, String permissionId) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getPermission");
}
// verify the required parameter 'permissionId' is set
if (permissionId == null) {
throw new ApiException(400, "Missing the required parameter 'permissionId' when calling getPermission");
}
// create path and map variables
String localVarPath = "/workspaces/{id}/permissions/{permissionId}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()))
.replaceAll("\\{" + "permissionId" + "\\}", apiClient.escapeString(permissionId.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "token" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* getPermissionStats
* Returns the total number of users within a workspace for each Permission Status (ACTIVE, ARCHIVED, INVITED...). Additionally, for each Permission Status a list of all Permission Roles (ADMIN, MEMBER...) and user count is provided. In other words, the result is a matrix of user counts with axis PermissionStatus and PermissionRole.
* @param id Workspace UUID (required)
* @return StatsListResponse
* @throws ApiException if fails to make API call
*/
public StatsListResponse getPermissionStats(String id) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getPermissionStats");
}
// create path and map variables
String localVarPath = "/workspaces/{id}/permissions/stats"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "token" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* getPermissions
* Retrieves all permission (users) for the workspace
* @param id Workspace UUID (required)
* @param q Query string to search in user (first name, last name, role) (optional)
* @param email Email to search for (optional)
* @param status Optional status to search for (optional)
* @param includeTechnicalUsers Include technical users in listing (optional)
* @param page The page number to access (1 indexed, defaults to 1) (optional, default to 1)
* @param size The page size requested (defaults to 30, max 100) (optional, default to 30)
* @param sort Comma-separated list of sorting (optional) (optional)
* @return PermissionListResponse
* @throws ApiException if fails to make API call
*/
public PermissionListResponse getPermissions(String id, String q, String email, String status, Boolean includeTechnicalUsers, Integer page, Integer size, String sort) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getPermissions");
}
// create path and map variables
String localVarPath = "/workspaces/{id}/permissions"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "q", q));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "email", email));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "status", status));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "includeTechnicalUsers", includeTechnicalUsers));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "page", page));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "size", size));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "sort", sort));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "token" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* getSettings
* Lists the workspace specific settings
* @param id Workspace UUID (required)
* @param type Setting type (optional)
* @return SettingListResponse
* @throws ApiException if fails to make API call
*/
public SettingListResponse getSettings(String id, String type) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getSettings");
}
// create path and map variables
String localVarPath = "/workspaces/{id}/settings"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "type", type));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "token" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* getUserListExport
* Creates an EXCEL export of the user list
* @param id Workspace UUID (required)
* @return DownloadKeyResponse
* @throws ApiException if fails to make API call
*/
public DownloadKeyResponse getUserListExport(String id) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getUserListExport");
}
// create path and map variables
String localVarPath = "/workspaces/{id}/permissions/export"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "token" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* getUsers
* Retrieves all users for the workspace
* @param id Workspace UUID (required)
* @param page The page number to access (1 indexed, defaults to 1) (optional, default to 1)
* @param size The page size requested (defaults to 30, max 100) (optional, default to 30)
* @param sort Comma-separated list of sorting (optional) (optional)
* @return UserListResponse
* @throws ApiException if fails to make API call
*/
public UserListResponse getUsers(String id, Integer page, Integer size, String sort) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getUsers");
}
// create path and map variables
String localVarPath = "/workspaces/{id}/users"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "page", page));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "size", size));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "sort", sort));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "token" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* getWorkspace
* Retrieves a workspace
* @param id Workspace UUID (required)
* @return WorkspaceResponse
* @throws ApiException if fails to make API call
*/
public WorkspaceResponse getWorkspace(String id) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling getWorkspace");
}
// create path and map variables
String localVarPath = "/workspaces/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "token" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* getWorkspaces
* List all workspaces for the requesting user
* @param q Search query (optional)
* @param page The page number to access (1 indexed, defaults to 1) (optional, default to 1)
* @param size The page size requested (defaults to 30, max 100) (optional, default to 30)
* @param sort Comma-separated list of sorting (optional) (optional, default to id-asc)
* @return WorkspaceListResponse
* @throws ApiException if fails to make API call
*/
public WorkspaceListResponse getWorkspaces(String q, Integer page, Integer size, String sort) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/workspaces";
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
localVarQueryParams.addAll(apiClient.parameterToPairs("", "q", q));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "page", page));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "size", size));
localVarQueryParams.addAll(apiClient.parameterToPairs("", "sort", sort));
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "token" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* updateWorkspace
* Updates a workspace
* @param id Workspace UUID (required)
* @param body (required)
* @return WorkspaceResponse
* @throws ApiException if fails to make API call
*/
public WorkspaceResponse updateWorkspace(String id, Workspace body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'id' is set
if (id == null) {
throw new ApiException(400, "Missing the required parameter 'id' when calling updateWorkspace");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException(400, "Missing the required parameter 'body' when calling updateWorkspace");
}
// create path and map variables
String localVarPath = "/workspaces/{id}"
.replaceAll("\\{" + "id" + "\\}", apiClient.escapeString(id.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { "token" };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "PUT", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy