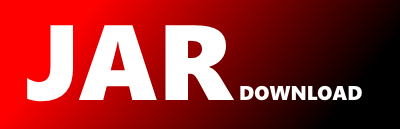
net.leanix.mtm.api.models.User Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leanix-mtm-sdk-java Show documentation
Show all versions of leanix-mtm-sdk-java Show documentation
SDK for Java to access leanIX MTM REST API
/*
* MTM
* Multi-tenancy-manager for LeanIX. Manages accounts, contracts, users, workspaces, permissions, and more.
*
* OpenAPI spec version: 1.6.348
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package net.leanix.mtm.api.models;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import net.leanix.mtm.api.models.Account;
import net.leanix.mtm.api.models.Link;
import net.leanix.mtm.api.models.Permission;
/**
* User
*/
public class User {
@JsonProperty("id")
private String id = null;
@JsonProperty("account")
private Account account = null;
@JsonProperty("userName")
private String userName = null;
@JsonProperty("technicalUserName")
private String technicalUserName = null;
@JsonProperty("email")
private String email = null;
@JsonProperty("technicalUserEmail")
private String technicalUserEmail = null;
@JsonProperty("firstName")
private String firstName = null;
@JsonProperty("lastName")
private String lastName = null;
@JsonProperty("department")
private String department = null;
@JsonProperty("lastLogin")
private OffsetDateTime lastLogin = null;
@JsonProperty("currentLogin")
private OffsetDateTime currentLogin = null;
@JsonProperty("apiKey")
private String apiKey = null;
@JsonProperty("feedKey")
private String feedKey = null;
/**
* Gets or Sets role
*/
public enum RoleEnum {
SUPERADMIN("SUPERADMIN"),
SYSTEM("SYSTEM"),
APICLIENT("APICLIENT"),
ACCOUNTADMIN("ACCOUNTADMIN"),
ACCOUNTUSER("ACCOUNTUSER");
private String value;
RoleEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static RoleEnum fromValue(String text) {
for (RoleEnum b : RoleEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
}
@JsonProperty("role")
private RoleEnum role = null;
/**
* Gets or Sets status
*/
public enum StatusEnum {
ACTIVE("ACTIVE"),
ARCHIVED("ARCHIVED");
private String value;
StatusEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static StatusEnum fromValue(String text) {
for (StatusEnum b : StatusEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
}
@JsonProperty("status")
private StatusEnum status = null;
@JsonProperty("crmTags")
private String crmTags = null;
@JsonProperty("crmLink")
private String crmLink = null;
@JsonProperty("numberOfFailedLoginAttempts")
private Integer numberOfFailedLoginAttempts = null;
@JsonProperty("technicalUser")
private Boolean technicalUser = false;
@JsonProperty("scimManaged")
private Boolean scimManaged = false;
@JsonProperty("transientUser")
private Boolean transientUser = false;
@JsonProperty("links")
private List links = null;
@JsonProperty("permissions")
private List permissions = null;
@JsonProperty("replayed")
private Boolean replayed = false;
@JsonProperty("displayName")
private String displayName = null;
@JsonProperty("active")
private Boolean active = false;
public User id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@ApiModelProperty(value = "")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public User account(Account account) {
this.account = account;
return this;
}
/**
* Get account
* @return account
**/
@ApiModelProperty(required = true, value = "")
public Account getAccount() {
return account;
}
public void setAccount(Account account) {
this.account = account;
}
public User userName(String userName) {
this.userName = userName;
return this;
}
/**
* Get userName
* @return userName
**/
@ApiModelProperty(value = "")
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public User technicalUserName(String technicalUserName) {
this.technicalUserName = technicalUserName;
return this;
}
/**
* Get technicalUserName
* @return technicalUserName
**/
@ApiModelProperty(value = "")
public String getTechnicalUserName() {
return technicalUserName;
}
public void setTechnicalUserName(String technicalUserName) {
this.technicalUserName = technicalUserName;
}
public User email(String email) {
this.email = email;
return this;
}
/**
* Get email
* @return email
**/
@ApiModelProperty(value = "")
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public User technicalUserEmail(String technicalUserEmail) {
this.technicalUserEmail = technicalUserEmail;
return this;
}
/**
* Get technicalUserEmail
* @return technicalUserEmail
**/
@ApiModelProperty(value = "")
public String getTechnicalUserEmail() {
return technicalUserEmail;
}
public void setTechnicalUserEmail(String technicalUserEmail) {
this.technicalUserEmail = technicalUserEmail;
}
public User firstName(String firstName) {
this.firstName = firstName;
return this;
}
/**
* Get firstName
* @return firstName
**/
@ApiModelProperty(value = "")
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public User lastName(String lastName) {
this.lastName = lastName;
return this;
}
/**
* Get lastName
* @return lastName
**/
@ApiModelProperty(value = "")
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public User department(String department) {
this.department = department;
return this;
}
/**
* Get department
* @return department
**/
@ApiModelProperty(value = "")
public String getDepartment() {
return department;
}
public void setDepartment(String department) {
this.department = department;
}
public User lastLogin(OffsetDateTime lastLogin) {
this.lastLogin = lastLogin;
return this;
}
/**
* Get lastLogin
* @return lastLogin
**/
@ApiModelProperty(value = "")
public OffsetDateTime getLastLogin() {
return lastLogin;
}
public void setLastLogin(OffsetDateTime lastLogin) {
this.lastLogin = lastLogin;
}
public User currentLogin(OffsetDateTime currentLogin) {
this.currentLogin = currentLogin;
return this;
}
/**
* Get currentLogin
* @return currentLogin
**/
@ApiModelProperty(value = "")
public OffsetDateTime getCurrentLogin() {
return currentLogin;
}
public void setCurrentLogin(OffsetDateTime currentLogin) {
this.currentLogin = currentLogin;
}
public User apiKey(String apiKey) {
this.apiKey = apiKey;
return this;
}
/**
* Get apiKey
* @return apiKey
**/
@ApiModelProperty(value = "")
public String getApiKey() {
return apiKey;
}
public void setApiKey(String apiKey) {
this.apiKey = apiKey;
}
public User feedKey(String feedKey) {
this.feedKey = feedKey;
return this;
}
/**
* Get feedKey
* @return feedKey
**/
@ApiModelProperty(value = "")
public String getFeedKey() {
return feedKey;
}
public void setFeedKey(String feedKey) {
this.feedKey = feedKey;
}
public User role(RoleEnum role) {
this.role = role;
return this;
}
/**
* Get role
* @return role
**/
@ApiModelProperty(required = true, value = "")
public RoleEnum getRole() {
return role;
}
public void setRole(RoleEnum role) {
this.role = role;
}
public User status(StatusEnum status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
**/
@ApiModelProperty(required = true, value = "")
public StatusEnum getStatus() {
return status;
}
public void setStatus(StatusEnum status) {
this.status = status;
}
public User crmTags(String crmTags) {
this.crmTags = crmTags;
return this;
}
/**
* Get crmTags
* @return crmTags
**/
@ApiModelProperty(value = "")
public String getCrmTags() {
return crmTags;
}
public void setCrmTags(String crmTags) {
this.crmTags = crmTags;
}
public User crmLink(String crmLink) {
this.crmLink = crmLink;
return this;
}
/**
* Get crmLink
* @return crmLink
**/
@ApiModelProperty(value = "")
public String getCrmLink() {
return crmLink;
}
public void setCrmLink(String crmLink) {
this.crmLink = crmLink;
}
public User numberOfFailedLoginAttempts(Integer numberOfFailedLoginAttempts) {
this.numberOfFailedLoginAttempts = numberOfFailedLoginAttempts;
return this;
}
/**
* Get numberOfFailedLoginAttempts
* @return numberOfFailedLoginAttempts
**/
@ApiModelProperty(value = "")
public Integer getNumberOfFailedLoginAttempts() {
return numberOfFailedLoginAttempts;
}
public void setNumberOfFailedLoginAttempts(Integer numberOfFailedLoginAttempts) {
this.numberOfFailedLoginAttempts = numberOfFailedLoginAttempts;
}
public User technicalUser(Boolean technicalUser) {
this.technicalUser = technicalUser;
return this;
}
/**
* Get technicalUser
* @return technicalUser
**/
@ApiModelProperty(value = "")
public Boolean getTechnicalUser() {
return technicalUser;
}
public void setTechnicalUser(Boolean technicalUser) {
this.technicalUser = technicalUser;
}
public User scimManaged(Boolean scimManaged) {
this.scimManaged = scimManaged;
return this;
}
/**
* Get scimManaged
* @return scimManaged
**/
@ApiModelProperty(value = "")
public Boolean getScimManaged() {
return scimManaged;
}
public void setScimManaged(Boolean scimManaged) {
this.scimManaged = scimManaged;
}
public User transientUser(Boolean transientUser) {
this.transientUser = transientUser;
return this;
}
/**
* Get transientUser
* @return transientUser
**/
@ApiModelProperty(value = "")
public Boolean getTransientUser() {
return transientUser;
}
public void setTransientUser(Boolean transientUser) {
this.transientUser = transientUser;
}
public User links(List links) {
this.links = links;
return this;
}
public User addLinksItem(Link linksItem) {
if (this.links == null) {
this.links = new ArrayList();
}
this.links.add(linksItem);
return this;
}
/**
* Get links
* @return links
**/
@ApiModelProperty(value = "")
public List getLinks() {
return links;
}
public void setLinks(List links) {
this.links = links;
}
public User permissions(List permissions) {
this.permissions = permissions;
return this;
}
public User addPermissionsItem(Permission permissionsItem) {
if (this.permissions == null) {
this.permissions = new ArrayList();
}
this.permissions.add(permissionsItem);
return this;
}
/**
* Get permissions
* @return permissions
**/
@ApiModelProperty(value = "")
public List getPermissions() {
return permissions;
}
public void setPermissions(List permissions) {
this.permissions = permissions;
}
public User replayed(Boolean replayed) {
this.replayed = replayed;
return this;
}
/**
* Get replayed
* @return replayed
**/
@ApiModelProperty(value = "")
public Boolean getReplayed() {
return replayed;
}
public void setReplayed(Boolean replayed) {
this.replayed = replayed;
}
public User displayName(String displayName) {
this.displayName = displayName;
return this;
}
/**
* Get displayName
* @return displayName
**/
@ApiModelProperty(value = "")
public String getDisplayName() {
return displayName;
}
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
public User active(Boolean active) {
this.active = active;
return this;
}
/**
* Get active
* @return active
**/
@ApiModelProperty(value = "")
public Boolean getActive() {
return active;
}
public void setActive(Boolean active) {
this.active = active;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
User user = (User) o;
return Objects.equals(this.id, user.id) &&
Objects.equals(this.account, user.account) &&
Objects.equals(this.userName, user.userName) &&
Objects.equals(this.technicalUserName, user.technicalUserName) &&
Objects.equals(this.email, user.email) &&
Objects.equals(this.technicalUserEmail, user.technicalUserEmail) &&
Objects.equals(this.firstName, user.firstName) &&
Objects.equals(this.lastName, user.lastName) &&
Objects.equals(this.department, user.department) &&
Objects.equals(this.lastLogin, user.lastLogin) &&
Objects.equals(this.currentLogin, user.currentLogin) &&
Objects.equals(this.apiKey, user.apiKey) &&
Objects.equals(this.feedKey, user.feedKey) &&
Objects.equals(this.role, user.role) &&
Objects.equals(this.status, user.status) &&
Objects.equals(this.crmTags, user.crmTags) &&
Objects.equals(this.crmLink, user.crmLink) &&
Objects.equals(this.numberOfFailedLoginAttempts, user.numberOfFailedLoginAttempts) &&
Objects.equals(this.technicalUser, user.technicalUser) &&
Objects.equals(this.scimManaged, user.scimManaged) &&
Objects.equals(this.transientUser, user.transientUser) &&
Objects.equals(this.links, user.links) &&
Objects.equals(this.permissions, user.permissions) &&
Objects.equals(this.replayed, user.replayed) &&
Objects.equals(this.displayName, user.displayName) &&
Objects.equals(this.active, user.active);
}
@Override
public int hashCode() {
return Objects.hash(id, account, userName, technicalUserName, email, technicalUserEmail, firstName, lastName, department, lastLogin, currentLogin, apiKey, feedKey, role, status, crmTags, crmLink, numberOfFailedLoginAttempts, technicalUser, scimManaged, transientUser, links, permissions, replayed, displayName, active);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class User {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" account: ").append(toIndentedString(account)).append("\n");
sb.append(" userName: ").append(toIndentedString(userName)).append("\n");
sb.append(" technicalUserName: ").append(toIndentedString(technicalUserName)).append("\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append(" technicalUserEmail: ").append(toIndentedString(technicalUserEmail)).append("\n");
sb.append(" firstName: ").append(toIndentedString(firstName)).append("\n");
sb.append(" lastName: ").append(toIndentedString(lastName)).append("\n");
sb.append(" department: ").append(toIndentedString(department)).append("\n");
sb.append(" lastLogin: ").append(toIndentedString(lastLogin)).append("\n");
sb.append(" currentLogin: ").append(toIndentedString(currentLogin)).append("\n");
sb.append(" apiKey: ").append(toIndentedString(apiKey)).append("\n");
sb.append(" feedKey: ").append(toIndentedString(feedKey)).append("\n");
sb.append(" role: ").append(toIndentedString(role)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" crmTags: ").append(toIndentedString(crmTags)).append("\n");
sb.append(" crmLink: ").append(toIndentedString(crmLink)).append("\n");
sb.append(" numberOfFailedLoginAttempts: ").append(toIndentedString(numberOfFailedLoginAttempts)).append("\n");
sb.append(" technicalUser: ").append(toIndentedString(technicalUser)).append("\n");
sb.append(" scimManaged: ").append(toIndentedString(scimManaged)).append("\n");
sb.append(" transientUser: ").append(toIndentedString(transientUser)).append("\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append(" permissions: ").append(toIndentedString(permissions)).append("\n");
sb.append(" replayed: ").append(toIndentedString(replayed)).append("\n");
sb.append(" displayName: ").append(toIndentedString(displayName)).append("\n");
sb.append(" active: ").append(toIndentedString(active)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy