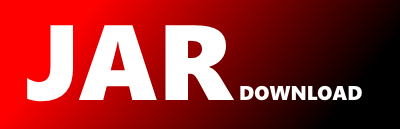
net.leanix.mtm.api.models.Workspace Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leanix-mtm-sdk-java Show documentation
Show all versions of leanix-mtm-sdk-java Show documentation
SDK for Java to access leanIX MTM REST API
/*
* MTM
* Multi-tenancy-manager for LeanIX. Manages accounts, contracts, users, workspaces, permissions, and more.
*
* OpenAPI spec version: 1.6.348
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package net.leanix.mtm.api.models;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import net.leanix.mtm.api.models.Contract;
import net.leanix.mtm.api.models.Domain;
import net.leanix.mtm.api.models.Instance;
import net.leanix.mtm.api.models.Link;
/**
* Workspace
*/
public class Workspace {
@JsonProperty("id")
private String id = null;
@JsonProperty("contract")
private Contract contract = null;
@JsonProperty("instance")
private Instance instance = null;
@JsonProperty("domain")
private Domain domain = null;
@JsonProperty("name")
private String name = null;
@JsonProperty("featureBundleId")
private String featureBundleId = null;
/**
* Gets or Sets status
*/
public enum StatusEnum {
ACTIVE("ACTIVE"),
BLOCKED("BLOCKED");
private String value;
StatusEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static StatusEnum fromValue(String text) {
for (StatusEnum b : StatusEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
}
@JsonProperty("status")
private StatusEnum status = null;
/**
* Gets or Sets type
*/
public enum TypeEnum {
LIVE("LIVE"),
DEMO("DEMO"),
SANDBOX("SANDBOX");
private String value;
TypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static TypeEnum fromValue(String text) {
for (TypeEnum b : TypeEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
}
@JsonProperty("type")
private TypeEnum type = null;
/**
* Gets or Sets defaultRole
*/
public enum DefaultRoleEnum {
ADMIN("ADMIN"),
MEMBER("MEMBER"),
VIEWER("VIEWER"),
CONTACT("CONTACT"),
SYSTEM_READ("SYSTEM_READ"),
SYSTEM_WRITE("SYSTEM_WRITE"),
SYSTEM_AS_USER("SYSTEM_AS_USER"),
TRANSIENT("TRANSIENT");
private String value;
DefaultRoleEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static DefaultRoleEnum fromValue(String text) {
for (DefaultRoleEnum b : DefaultRoleEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
}
@JsonProperty("defaultRole")
private DefaultRoleEnum defaultRole = null;
@JsonProperty("comment")
private String comment = null;
@JsonProperty("createdAt")
private OffsetDateTime createdAt = null;
@JsonProperty("invitationOnly")
private Boolean invitationOnly = false;
@JsonProperty("allowTransientUsers")
private Boolean allowTransientUsers = false;
@JsonProperty("links")
private List links = null;
@JsonProperty("url")
private String url = null;
@JsonProperty("active")
private Boolean active = false;
public Workspace id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@ApiModelProperty(value = "")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public Workspace contract(Contract contract) {
this.contract = contract;
return this;
}
/**
* Get contract
* @return contract
**/
@ApiModelProperty(required = true, value = "")
public Contract getContract() {
return contract;
}
public void setContract(Contract contract) {
this.contract = contract;
}
public Workspace instance(Instance instance) {
this.instance = instance;
return this;
}
/**
* Get instance
* @return instance
**/
@ApiModelProperty(value = "")
public Instance getInstance() {
return instance;
}
public void setInstance(Instance instance) {
this.instance = instance;
}
public Workspace domain(Domain domain) {
this.domain = domain;
return this;
}
/**
* Get domain
* @return domain
**/
@ApiModelProperty(value = "")
public Domain getDomain() {
return domain;
}
public void setDomain(Domain domain) {
this.domain = domain;
}
public Workspace name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
**/
@ApiModelProperty(value = "")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Workspace featureBundleId(String featureBundleId) {
this.featureBundleId = featureBundleId;
return this;
}
/**
* Get featureBundleId
* @return featureBundleId
**/
@ApiModelProperty(value = "")
public String getFeatureBundleId() {
return featureBundleId;
}
public void setFeatureBundleId(String featureBundleId) {
this.featureBundleId = featureBundleId;
}
public Workspace status(StatusEnum status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
**/
@ApiModelProperty(value = "")
public StatusEnum getStatus() {
return status;
}
public void setStatus(StatusEnum status) {
this.status = status;
}
public Workspace type(TypeEnum type) {
this.type = type;
return this;
}
/**
* Get type
* @return type
**/
@ApiModelProperty(value = "")
public TypeEnum getType() {
return type;
}
public void setType(TypeEnum type) {
this.type = type;
}
public Workspace defaultRole(DefaultRoleEnum defaultRole) {
this.defaultRole = defaultRole;
return this;
}
/**
* Get defaultRole
* @return defaultRole
**/
@ApiModelProperty(value = "")
public DefaultRoleEnum getDefaultRole() {
return defaultRole;
}
public void setDefaultRole(DefaultRoleEnum defaultRole) {
this.defaultRole = defaultRole;
}
public Workspace comment(String comment) {
this.comment = comment;
return this;
}
/**
* Get comment
* @return comment
**/
@ApiModelProperty(value = "")
public String getComment() {
return comment;
}
public void setComment(String comment) {
this.comment = comment;
}
public Workspace createdAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* Get createdAt
* @return createdAt
**/
@ApiModelProperty(value = "")
public OffsetDateTime getCreatedAt() {
return createdAt;
}
public void setCreatedAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
}
public Workspace invitationOnly(Boolean invitationOnly) {
this.invitationOnly = invitationOnly;
return this;
}
/**
* Get invitationOnly
* @return invitationOnly
**/
@ApiModelProperty(value = "")
public Boolean getInvitationOnly() {
return invitationOnly;
}
public void setInvitationOnly(Boolean invitationOnly) {
this.invitationOnly = invitationOnly;
}
public Workspace allowTransientUsers(Boolean allowTransientUsers) {
this.allowTransientUsers = allowTransientUsers;
return this;
}
/**
* Get allowTransientUsers
* @return allowTransientUsers
**/
@ApiModelProperty(value = "")
public Boolean getAllowTransientUsers() {
return allowTransientUsers;
}
public void setAllowTransientUsers(Boolean allowTransientUsers) {
this.allowTransientUsers = allowTransientUsers;
}
public Workspace links(List links) {
this.links = links;
return this;
}
public Workspace addLinksItem(Link linksItem) {
if (this.links == null) {
this.links = new ArrayList();
}
this.links.add(linksItem);
return this;
}
/**
* Get links
* @return links
**/
@ApiModelProperty(value = "")
public List getLinks() {
return links;
}
public void setLinks(List links) {
this.links = links;
}
/**
* Get url
* @return url
**/
@ApiModelProperty(value = "")
public String getUrl() {
return url;
}
/**
* Get active
* @return active
**/
@ApiModelProperty(value = "")
public Boolean getActive() {
return active;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Workspace workspace = (Workspace) o;
return Objects.equals(this.id, workspace.id) &&
Objects.equals(this.contract, workspace.contract) &&
Objects.equals(this.instance, workspace.instance) &&
Objects.equals(this.domain, workspace.domain) &&
Objects.equals(this.name, workspace.name) &&
Objects.equals(this.featureBundleId, workspace.featureBundleId) &&
Objects.equals(this.status, workspace.status) &&
Objects.equals(this.type, workspace.type) &&
Objects.equals(this.defaultRole, workspace.defaultRole) &&
Objects.equals(this.comment, workspace.comment) &&
Objects.equals(this.createdAt, workspace.createdAt) &&
Objects.equals(this.invitationOnly, workspace.invitationOnly) &&
Objects.equals(this.allowTransientUsers, workspace.allowTransientUsers) &&
Objects.equals(this.links, workspace.links) &&
Objects.equals(this.url, workspace.url) &&
Objects.equals(this.active, workspace.active);
}
@Override
public int hashCode() {
return Objects.hash(id, contract, instance, domain, name, featureBundleId, status, type, defaultRole, comment, createdAt, invitationOnly, allowTransientUsers, links, url, active);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Workspace {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" contract: ").append(toIndentedString(contract)).append("\n");
sb.append(" instance: ").append(toIndentedString(instance)).append("\n");
sb.append(" domain: ").append(toIndentedString(domain)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" featureBundleId: ").append(toIndentedString(featureBundleId)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" defaultRole: ").append(toIndentedString(defaultRole)).append("\n");
sb.append(" comment: ").append(toIndentedString(comment)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" invitationOnly: ").append(toIndentedString(invitationOnly)).append("\n");
sb.append(" allowTransientUsers: ").append(toIndentedString(allowTransientUsers)).append("\n");
sb.append(" links: ").append(toIndentedString(links)).append("\n");
sb.append(" url: ").append(toIndentedString(url)).append("\n");
sb.append(" active: ").append(toIndentedString(active)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy