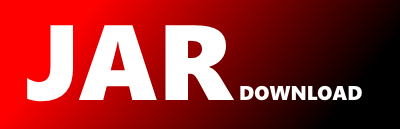
net.leanix.api.ProcessesApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leanix-sdk-java Show documentation
Show all versions of leanix-sdk-java Show documentation
SDK for Java to access leanIX REST API
package net.leanix.api;
import net.leanix.api.common.ApiException;
import net.leanix.api.common.ApiClient;
import net.leanix.api.common.Configuration;
import net.leanix.api.common.Pair;
import javax.ws.rs.core.GenericType;
import net.leanix.api.models.FactSheetHasChild;
import net.leanix.api.models.FactSheetHasDocument;
import net.leanix.api.models.FactSheetHasLifecycle;
import net.leanix.api.models.FactSheetHasParent;
import net.leanix.api.models.FactSheetHasPredecessor;
import net.leanix.api.models.FactSheetHasRequiredby;
import net.leanix.api.models.FactSheetHasRequires;
import net.leanix.api.models.FactSheetHasSuccessor;
import net.leanix.api.models.Process;
import net.leanix.api.models.ProcessHasBusinessCapability;
import net.leanix.api.models.ProjectHasProcess;
import net.leanix.api.models.ServiceHasProcess;
import net.leanix.api.models.UserSubscription;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class ProcessesApi {
private ApiClient apiClient;
public ProcessesApi() {
this(Configuration.getDefaultApiClient());
}
public ProcessesApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Create a new relation
*
* @param ID Unique ID (required)
* @param body Message-Body (optional)
* @return FactSheetHasChild
* @throws ApiException if fails to make API call
*/
public FactSheetHasChild createFactSheetHasChild(String ID, FactSheetHasChild body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling createFactSheetHasChild");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasChildren".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Create a new relation
*
* @param ID Unique ID (required)
* @param body Message-Body (optional)
* @return FactSheetHasDocument
* @throws ApiException if fails to make API call
*/
public FactSheetHasDocument createFactSheetHasDocument(String ID, FactSheetHasDocument body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling createFactSheetHasDocument");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasDocuments".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Create a new relation
*
* @param ID Unique ID (required)
* @param body Message-Body (optional)
* @return FactSheetHasLifecycle
* @throws ApiException if fails to make API call
*/
public FactSheetHasLifecycle createFactSheetHasLifecycle(String ID, FactSheetHasLifecycle body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling createFactSheetHasLifecycle");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasLifecycles".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Create a new relation
*
* @param ID Unique ID (required)
* @param body Message-Body (optional)
* @return FactSheetHasParent
* @throws ApiException if fails to make API call
*/
public FactSheetHasParent createFactSheetHasParent(String ID, FactSheetHasParent body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling createFactSheetHasParent");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasParents".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Create a new relation
*
* @param ID Unique ID (required)
* @param body Message-Body (optional)
* @return FactSheetHasPredecessor
* @throws ApiException if fails to make API call
*/
public FactSheetHasPredecessor createFactSheetHasPredecessor(String ID, FactSheetHasPredecessor body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling createFactSheetHasPredecessor");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasPredecessors".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Create a new relation
*
* @param ID Unique ID (required)
* @param body Message-Body (optional)
* @return FactSheetHasRequiredby
* @throws ApiException if fails to make API call
*/
public FactSheetHasRequiredby createFactSheetHasRequiredby(String ID, FactSheetHasRequiredby body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling createFactSheetHasRequiredby");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasRequiredby".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Create a new relation
*
* @param ID Unique ID (required)
* @param body Message-Body (optional)
* @return FactSheetHasRequires
* @throws ApiException if fails to make API call
*/
public FactSheetHasRequires createFactSheetHasRequires(String ID, FactSheetHasRequires body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling createFactSheetHasRequires");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasRequires".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Create a new relation
*
* @param ID Unique ID (required)
* @param body Message-Body (optional)
* @return FactSheetHasSuccessor
* @throws ApiException if fails to make API call
*/
public FactSheetHasSuccessor createFactSheetHasSuccessor(String ID, FactSheetHasSuccessor body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling createFactSheetHasSuccessor");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasSuccessors".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Create a new Process
*
* @param body Message-Body (optional)
* @return Process
* @throws ApiException if fails to make API call
*/
public Process createProcess(Process body) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/processes".replaceAll("\\{format\\}","json");
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Create a new relation
*
* @param ID Unique ID (required)
* @param body Message-Body (optional)
* @return ProcessHasBusinessCapability
* @throws ApiException if fails to make API call
*/
public ProcessHasBusinessCapability createProcessHasBusinessCapability(String ID, ProcessHasBusinessCapability body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling createProcessHasBusinessCapability");
}
// create path and map variables
String localVarPath = "/processes/{ID}/processHasBusinessCapabilities".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Create a new relation
*
* @param ID Unique ID (required)
* @param body Message-Body (optional)
* @return ProjectHasProcess
* @throws ApiException if fails to make API call
*/
public ProjectHasProcess createProjectHasProcess(String ID, ProjectHasProcess body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling createProjectHasProcess");
}
// create path and map variables
String localVarPath = "/processes/{ID}/projectHasProcesses".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Create a new relation
*
* @param ID Unique ID (required)
* @param body Message-Body (optional)
* @return ServiceHasProcess
* @throws ApiException if fails to make API call
*/
public ServiceHasProcess createServiceHasProcess(String ID, ServiceHasProcess body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling createServiceHasProcess");
}
// create path and map variables
String localVarPath = "/processes/{ID}/serviceHasProcesses".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Create a new relation
*
* @param ID Unique ID (required)
* @param body Message-Body (optional)
* @return UserSubscription
* @throws ApiException if fails to make API call
*/
public UserSubscription createUserSubscription(String ID, UserSubscription body) throws ApiException {
Object localVarPostBody = body;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling createUserSubscription");
}
// create path and map variables
String localVarPath = "/processes/{ID}/userSubscriptions".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Delete relation by a given relationID
*
* @param ID Unique ID (required)
* @param relationID Unique ID of the Relation (required)
* @throws ApiException if fails to make API call
*/
public void deleteFactSheetHasChild(String ID, String relationID) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling deleteFactSheetHasChild");
}
// verify the required parameter 'relationID' is set
if (relationID == null) {
throw new ApiException(400, "Missing the required parameter 'relationID' when calling deleteFactSheetHasChild");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasChildren/{relationID}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()))
.replaceAll("\\{" + "relationID" + "\\}", apiClient.escapeString(relationID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete relation by a given relationID
*
* @param ID Unique ID (required)
* @param relationID Unique ID of the Relation (required)
* @throws ApiException if fails to make API call
*/
public void deleteFactSheetHasDocument(String ID, String relationID) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling deleteFactSheetHasDocument");
}
// verify the required parameter 'relationID' is set
if (relationID == null) {
throw new ApiException(400, "Missing the required parameter 'relationID' when calling deleteFactSheetHasDocument");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasDocuments/{relationID}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()))
.replaceAll("\\{" + "relationID" + "\\}", apiClient.escapeString(relationID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete relation by a given relationID
*
* @param ID Unique ID (required)
* @param relationID Unique ID of the Relation (required)
* @throws ApiException if fails to make API call
*/
public void deleteFactSheetHasLifecycle(String ID, String relationID) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling deleteFactSheetHasLifecycle");
}
// verify the required parameter 'relationID' is set
if (relationID == null) {
throw new ApiException(400, "Missing the required parameter 'relationID' when calling deleteFactSheetHasLifecycle");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasLifecycles/{relationID}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()))
.replaceAll("\\{" + "relationID" + "\\}", apiClient.escapeString(relationID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete relation by a given relationID
*
* @param ID Unique ID (required)
* @param relationID Unique ID of the Relation (required)
* @throws ApiException if fails to make API call
*/
public void deleteFactSheetHasParent(String ID, String relationID) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling deleteFactSheetHasParent");
}
// verify the required parameter 'relationID' is set
if (relationID == null) {
throw new ApiException(400, "Missing the required parameter 'relationID' when calling deleteFactSheetHasParent");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasParents/{relationID}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()))
.replaceAll("\\{" + "relationID" + "\\}", apiClient.escapeString(relationID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete relation by a given relationID
*
* @param ID Unique ID (required)
* @param relationID Unique ID of the Relation (required)
* @throws ApiException if fails to make API call
*/
public void deleteFactSheetHasPredecessor(String ID, String relationID) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling deleteFactSheetHasPredecessor");
}
// verify the required parameter 'relationID' is set
if (relationID == null) {
throw new ApiException(400, "Missing the required parameter 'relationID' when calling deleteFactSheetHasPredecessor");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasPredecessors/{relationID}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()))
.replaceAll("\\{" + "relationID" + "\\}", apiClient.escapeString(relationID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete relation by a given relationID
*
* @param ID Unique ID (required)
* @param relationID Unique ID of the Relation (required)
* @throws ApiException if fails to make API call
*/
public void deleteFactSheetHasRequiredby(String ID, String relationID) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling deleteFactSheetHasRequiredby");
}
// verify the required parameter 'relationID' is set
if (relationID == null) {
throw new ApiException(400, "Missing the required parameter 'relationID' when calling deleteFactSheetHasRequiredby");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasRequiredby/{relationID}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()))
.replaceAll("\\{" + "relationID" + "\\}", apiClient.escapeString(relationID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete relation by a given relationID
*
* @param ID Unique ID (required)
* @param relationID Unique ID of the Relation (required)
* @throws ApiException if fails to make API call
*/
public void deleteFactSheetHasRequires(String ID, String relationID) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling deleteFactSheetHasRequires");
}
// verify the required parameter 'relationID' is set
if (relationID == null) {
throw new ApiException(400, "Missing the required parameter 'relationID' when calling deleteFactSheetHasRequires");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasRequires/{relationID}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()))
.replaceAll("\\{" + "relationID" + "\\}", apiClient.escapeString(relationID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete relation by a given relationID
*
* @param ID Unique ID (required)
* @param relationID Unique ID of the Relation (required)
* @throws ApiException if fails to make API call
*/
public void deleteFactSheetHasSuccessor(String ID, String relationID) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling deleteFactSheetHasSuccessor");
}
// verify the required parameter 'relationID' is set
if (relationID == null) {
throw new ApiException(400, "Missing the required parameter 'relationID' when calling deleteFactSheetHasSuccessor");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasSuccessors/{relationID}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()))
.replaceAll("\\{" + "relationID" + "\\}", apiClient.escapeString(relationID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete a Process by a given ID
*
* @param ID Unique ID (required)
* @throws ApiException if fails to make API call
*/
public void deleteProcess(String ID) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling deleteProcess");
}
// create path and map variables
String localVarPath = "/processes/{ID}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete relation by a given relationID
*
* @param ID Unique ID (required)
* @param relationID Unique ID of the Relation (required)
* @throws ApiException if fails to make API call
*/
public void deleteProcessHasBusinessCapability(String ID, String relationID) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling deleteProcessHasBusinessCapability");
}
// verify the required parameter 'relationID' is set
if (relationID == null) {
throw new ApiException(400, "Missing the required parameter 'relationID' when calling deleteProcessHasBusinessCapability");
}
// create path and map variables
String localVarPath = "/processes/{ID}/processHasBusinessCapabilities/{relationID}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()))
.replaceAll("\\{" + "relationID" + "\\}", apiClient.escapeString(relationID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete relation by a given relationID
*
* @param ID Unique ID (required)
* @param relationID Unique ID of the Relation (required)
* @throws ApiException if fails to make API call
*/
public void deleteProjectHasProcess(String ID, String relationID) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling deleteProjectHasProcess");
}
// verify the required parameter 'relationID' is set
if (relationID == null) {
throw new ApiException(400, "Missing the required parameter 'relationID' when calling deleteProjectHasProcess");
}
// create path and map variables
String localVarPath = "/processes/{ID}/projectHasProcesses/{relationID}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()))
.replaceAll("\\{" + "relationID" + "\\}", apiClient.escapeString(relationID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete relation by a given relationID
*
* @param ID Unique ID (required)
* @param relationID Unique ID of the Relation (required)
* @throws ApiException if fails to make API call
*/
public void deleteServiceHasProcess(String ID, String relationID) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling deleteServiceHasProcess");
}
// verify the required parameter 'relationID' is set
if (relationID == null) {
throw new ApiException(400, "Missing the required parameter 'relationID' when calling deleteServiceHasProcess");
}
// create path and map variables
String localVarPath = "/processes/{ID}/serviceHasProcesses/{relationID}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()))
.replaceAll("\\{" + "relationID" + "\\}", apiClient.escapeString(relationID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Delete relation by a given relationID
*
* @param ID Unique ID (required)
* @param relationID Unique ID of the Relation (required)
* @throws ApiException if fails to make API call
*/
public void deleteUserSubscription(String ID, String relationID) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling deleteUserSubscription");
}
// verify the required parameter 'relationID' is set
if (relationID == null) {
throw new ApiException(400, "Missing the required parameter 'relationID' when calling deleteUserSubscription");
}
// create path and map variables
String localVarPath = "/processes/{ID}/userSubscriptions/{relationID}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()))
.replaceAll("\\{" + "relationID" + "\\}", apiClient.escapeString(relationID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
apiClient.invokeAPI(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, null);
}
/**
* Read by relationID
*
* @param ID Unique ID (required)
* @param relationID Unique ID of the Relation (required)
* @return FactSheetHasChild
* @throws ApiException if fails to make API call
*/
public FactSheetHasChild getFactSheetHasChild(String ID, String relationID) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling getFactSheetHasChild");
}
// verify the required parameter 'relationID' is set
if (relationID == null) {
throw new ApiException(400, "Missing the required parameter 'relationID' when calling getFactSheetHasChild");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasChildren/{relationID}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()))
.replaceAll("\\{" + "relationID" + "\\}", apiClient.escapeString(relationID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Read all of relation
*
* @param ID Unique ID (required)
* @return List
* @throws ApiException if fails to make API call
*/
public List getFactSheetHasChildren(String ID) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling getFactSheetHasChildren");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasChildren".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType> localVarReturnType = new GenericType>() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Read by relationID
*
* @param ID Unique ID (required)
* @param relationID Unique ID of the Relation (required)
* @return FactSheetHasDocument
* @throws ApiException if fails to make API call
*/
public FactSheetHasDocument getFactSheetHasDocument(String ID, String relationID) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling getFactSheetHasDocument");
}
// verify the required parameter 'relationID' is set
if (relationID == null) {
throw new ApiException(400, "Missing the required parameter 'relationID' when calling getFactSheetHasDocument");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasDocuments/{relationID}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()))
.replaceAll("\\{" + "relationID" + "\\}", apiClient.escapeString(relationID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Read all of relation
*
* @param ID Unique ID (required)
* @return List
* @throws ApiException if fails to make API call
*/
public List getFactSheetHasDocuments(String ID) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling getFactSheetHasDocuments");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasDocuments".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType> localVarReturnType = new GenericType>() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Read by relationID
*
* @param ID Unique ID (required)
* @param relationID Unique ID of the Relation (required)
* @return FactSheetHasLifecycle
* @throws ApiException if fails to make API call
*/
public FactSheetHasLifecycle getFactSheetHasLifecycle(String ID, String relationID) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling getFactSheetHasLifecycle");
}
// verify the required parameter 'relationID' is set
if (relationID == null) {
throw new ApiException(400, "Missing the required parameter 'relationID' when calling getFactSheetHasLifecycle");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasLifecycles/{relationID}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()))
.replaceAll("\\{" + "relationID" + "\\}", apiClient.escapeString(relationID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Read all of relation
*
* @param ID Unique ID (required)
* @return List
* @throws ApiException if fails to make API call
*/
public List getFactSheetHasLifecycles(String ID) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling getFactSheetHasLifecycles");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasLifecycles".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType> localVarReturnType = new GenericType>() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Read by relationID
*
* @param ID Unique ID (required)
* @param relationID Unique ID of the Relation (required)
* @return FactSheetHasParent
* @throws ApiException if fails to make API call
*/
public FactSheetHasParent getFactSheetHasParent(String ID, String relationID) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling getFactSheetHasParent");
}
// verify the required parameter 'relationID' is set
if (relationID == null) {
throw new ApiException(400, "Missing the required parameter 'relationID' when calling getFactSheetHasParent");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasParents/{relationID}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()))
.replaceAll("\\{" + "relationID" + "\\}", apiClient.escapeString(relationID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Read all of relation
*
* @param ID Unique ID (required)
* @return List
* @throws ApiException if fails to make API call
*/
public List getFactSheetHasParents(String ID) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'ID' is set
if (ID == null) {
throw new ApiException(400, "Missing the required parameter 'ID' when calling getFactSheetHasParents");
}
// create path and map variables
String localVarPath = "/processes/{ID}/factSheetHasParents".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "ID" + "\\}", apiClient.escapeString(ID.toString()));
// query params
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map