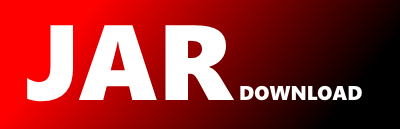
net.leanix.api.models.BusinessObject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leanix-sdk-java Show documentation
Show all versions of leanix-sdk-java Show documentation
SDK for Java to access leanIX REST API
package net.leanix.api.models;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
import net.leanix.api.models.FactSheetHasChild;
import net.leanix.api.models.FactSheetHasDocument;
import net.leanix.api.models.FactSheetHasLifecycle;
import net.leanix.api.models.FactSheetHasParent;
import net.leanix.api.models.FactSheetHasPredecessor;
import net.leanix.api.models.FactSheetHasRequiredby;
import net.leanix.api.models.FactSheetHasRequires;
import net.leanix.api.models.FactSheetHasSuccessor;
import net.leanix.api.models.IfaceHasBusinessObject;
import net.leanix.api.models.ServiceHasBusinessObject;
import net.leanix.api.models.UserSubscription;
public class BusinessObject {
private String ID = null;
private String displayName = null;
private String parentID = null;
private Long level = null;
private String name = null;
private String reference = null;
private String alias = null;
private String description = null;
private String dataClassificationID = null;
private String dataClassificationDescription = null;
private String objectStatusID = null;
private List tags = new ArrayList();
private String fullName = null;
private String resourceType = null;
private String completion = null;
private String qualitySealExpiry = null;
private String modificationTime = null;
private List factSheetHasParents = new ArrayList();
private List factSheetHasChildren = new ArrayList();
private List factSheetHasDocuments = new ArrayList();
private List factSheetHasLifecycles = new ArrayList();
private List userSubscriptions = new ArrayList();
private List factSheetHasPredecessors = new ArrayList();
private List factSheetHasSuccessors = new ArrayList();
private List factSheetHasRequires = new ArrayList();
private List factSheetHasRequiredby = new ArrayList();
private List serviceHasBusinessObjects = new ArrayList();
private List ifaceHasBusinessObjects = new ArrayList();
/**
**/
public BusinessObject ID(String ID) {
this.ID = ID;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("ID")
public String getID() {
return ID;
}
public void setID(String ID) {
this.ID = ID;
}
/**
**/
public BusinessObject displayName(String displayName) {
this.displayName = displayName;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("displayName")
public String getDisplayName() {
return displayName;
}
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
/**
**/
public BusinessObject parentID(String parentID) {
this.parentID = parentID;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("parentID")
public String getParentID() {
return parentID;
}
public void setParentID(String parentID) {
this.parentID = parentID;
}
/**
**/
public BusinessObject level(Long level) {
this.level = level;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("level")
public Long getLevel() {
return level;
}
public void setLevel(Long level) {
this.level = level;
}
/**
**/
public BusinessObject name(String name) {
this.name = name;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("name")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
/**
**/
public BusinessObject reference(String reference) {
this.reference = reference;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("reference")
public String getReference() {
return reference;
}
public void setReference(String reference) {
this.reference = reference;
}
/**
**/
public BusinessObject alias(String alias) {
this.alias = alias;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("alias")
public String getAlias() {
return alias;
}
public void setAlias(String alias) {
this.alias = alias;
}
/**
**/
public BusinessObject description(String description) {
this.description = description;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("description")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
/**
**/
public BusinessObject dataClassificationID(String dataClassificationID) {
this.dataClassificationID = dataClassificationID;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("dataClassificationID")
public String getDataClassificationID() {
return dataClassificationID;
}
public void setDataClassificationID(String dataClassificationID) {
this.dataClassificationID = dataClassificationID;
}
/**
**/
public BusinessObject dataClassificationDescription(String dataClassificationDescription) {
this.dataClassificationDescription = dataClassificationDescription;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("dataClassificationDescription")
public String getDataClassificationDescription() {
return dataClassificationDescription;
}
public void setDataClassificationDescription(String dataClassificationDescription) {
this.dataClassificationDescription = dataClassificationDescription;
}
/**
**/
public BusinessObject objectStatusID(String objectStatusID) {
this.objectStatusID = objectStatusID;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("objectStatusID")
public String getObjectStatusID() {
return objectStatusID;
}
public void setObjectStatusID(String objectStatusID) {
this.objectStatusID = objectStatusID;
}
/**
**/
public BusinessObject tags(List tags) {
this.tags = tags;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("tags")
public List getTags() {
return tags;
}
public void setTags(List tags) {
this.tags = tags;
}
/**
**/
public BusinessObject fullName(String fullName) {
this.fullName = fullName;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("fullName")
public String getFullName() {
return fullName;
}
public void setFullName(String fullName) {
this.fullName = fullName;
}
/**
**/
public BusinessObject resourceType(String resourceType) {
this.resourceType = resourceType;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("resourceType")
public String getResourceType() {
return resourceType;
}
public void setResourceType(String resourceType) {
this.resourceType = resourceType;
}
/**
**/
public BusinessObject completion(String completion) {
this.completion = completion;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("completion")
public String getCompletion() {
return completion;
}
public void setCompletion(String completion) {
this.completion = completion;
}
/**
**/
public BusinessObject qualitySealExpiry(String qualitySealExpiry) {
this.qualitySealExpiry = qualitySealExpiry;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("qualitySealExpiry")
public String getQualitySealExpiry() {
return qualitySealExpiry;
}
public void setQualitySealExpiry(String qualitySealExpiry) {
this.qualitySealExpiry = qualitySealExpiry;
}
/**
**/
public BusinessObject modificationTime(String modificationTime) {
this.modificationTime = modificationTime;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("modificationTime")
public String getModificationTime() {
return modificationTime;
}
public void setModificationTime(String modificationTime) {
this.modificationTime = modificationTime;
}
/**
**/
public BusinessObject factSheetHasParents(List factSheetHasParents) {
this.factSheetHasParents = factSheetHasParents;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasParents")
public List getFactSheetHasParents() {
return factSheetHasParents;
}
public void setFactSheetHasParents(List factSheetHasParents) {
this.factSheetHasParents = factSheetHasParents;
}
/**
**/
public BusinessObject factSheetHasChildren(List factSheetHasChildren) {
this.factSheetHasChildren = factSheetHasChildren;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasChildren")
public List getFactSheetHasChildren() {
return factSheetHasChildren;
}
public void setFactSheetHasChildren(List factSheetHasChildren) {
this.factSheetHasChildren = factSheetHasChildren;
}
/**
**/
public BusinessObject factSheetHasDocuments(List factSheetHasDocuments) {
this.factSheetHasDocuments = factSheetHasDocuments;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasDocuments")
public List getFactSheetHasDocuments() {
return factSheetHasDocuments;
}
public void setFactSheetHasDocuments(List factSheetHasDocuments) {
this.factSheetHasDocuments = factSheetHasDocuments;
}
/**
**/
public BusinessObject factSheetHasLifecycles(List factSheetHasLifecycles) {
this.factSheetHasLifecycles = factSheetHasLifecycles;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasLifecycles")
public List getFactSheetHasLifecycles() {
return factSheetHasLifecycles;
}
public void setFactSheetHasLifecycles(List factSheetHasLifecycles) {
this.factSheetHasLifecycles = factSheetHasLifecycles;
}
/**
**/
public BusinessObject userSubscriptions(List userSubscriptions) {
this.userSubscriptions = userSubscriptions;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("userSubscriptions")
public List getUserSubscriptions() {
return userSubscriptions;
}
public void setUserSubscriptions(List userSubscriptions) {
this.userSubscriptions = userSubscriptions;
}
/**
**/
public BusinessObject factSheetHasPredecessors(List factSheetHasPredecessors) {
this.factSheetHasPredecessors = factSheetHasPredecessors;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasPredecessors")
public List getFactSheetHasPredecessors() {
return factSheetHasPredecessors;
}
public void setFactSheetHasPredecessors(List factSheetHasPredecessors) {
this.factSheetHasPredecessors = factSheetHasPredecessors;
}
/**
**/
public BusinessObject factSheetHasSuccessors(List factSheetHasSuccessors) {
this.factSheetHasSuccessors = factSheetHasSuccessors;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasSuccessors")
public List getFactSheetHasSuccessors() {
return factSheetHasSuccessors;
}
public void setFactSheetHasSuccessors(List factSheetHasSuccessors) {
this.factSheetHasSuccessors = factSheetHasSuccessors;
}
/**
**/
public BusinessObject factSheetHasRequires(List factSheetHasRequires) {
this.factSheetHasRequires = factSheetHasRequires;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasRequires")
public List getFactSheetHasRequires() {
return factSheetHasRequires;
}
public void setFactSheetHasRequires(List factSheetHasRequires) {
this.factSheetHasRequires = factSheetHasRequires;
}
/**
**/
public BusinessObject factSheetHasRequiredby(List factSheetHasRequiredby) {
this.factSheetHasRequiredby = factSheetHasRequiredby;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasRequiredby")
public List getFactSheetHasRequiredby() {
return factSheetHasRequiredby;
}
public void setFactSheetHasRequiredby(List factSheetHasRequiredby) {
this.factSheetHasRequiredby = factSheetHasRequiredby;
}
/**
**/
public BusinessObject serviceHasBusinessObjects(List serviceHasBusinessObjects) {
this.serviceHasBusinessObjects = serviceHasBusinessObjects;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("serviceHasBusinessObjects")
public List getServiceHasBusinessObjects() {
return serviceHasBusinessObjects;
}
public void setServiceHasBusinessObjects(List serviceHasBusinessObjects) {
this.serviceHasBusinessObjects = serviceHasBusinessObjects;
}
/**
**/
public BusinessObject ifaceHasBusinessObjects(List ifaceHasBusinessObjects) {
this.ifaceHasBusinessObjects = ifaceHasBusinessObjects;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("ifaceHasBusinessObjects")
public List getIfaceHasBusinessObjects() {
return ifaceHasBusinessObjects;
}
public void setIfaceHasBusinessObjects(List ifaceHasBusinessObjects) {
this.ifaceHasBusinessObjects = ifaceHasBusinessObjects;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
BusinessObject businessObject = (BusinessObject) o;
return Objects.equals(this.ID, businessObject.ID) &&
Objects.equals(this.displayName, businessObject.displayName) &&
Objects.equals(this.parentID, businessObject.parentID) &&
Objects.equals(this.level, businessObject.level) &&
Objects.equals(this.name, businessObject.name) &&
Objects.equals(this.reference, businessObject.reference) &&
Objects.equals(this.alias, businessObject.alias) &&
Objects.equals(this.description, businessObject.description) &&
Objects.equals(this.dataClassificationID, businessObject.dataClassificationID) &&
Objects.equals(this.dataClassificationDescription, businessObject.dataClassificationDescription) &&
Objects.equals(this.objectStatusID, businessObject.objectStatusID) &&
Objects.equals(this.tags, businessObject.tags) &&
Objects.equals(this.fullName, businessObject.fullName) &&
Objects.equals(this.resourceType, businessObject.resourceType) &&
Objects.equals(this.completion, businessObject.completion) &&
Objects.equals(this.qualitySealExpiry, businessObject.qualitySealExpiry) &&
Objects.equals(this.modificationTime, businessObject.modificationTime) &&
Objects.equals(this.factSheetHasParents, businessObject.factSheetHasParents) &&
Objects.equals(this.factSheetHasChildren, businessObject.factSheetHasChildren) &&
Objects.equals(this.factSheetHasDocuments, businessObject.factSheetHasDocuments) &&
Objects.equals(this.factSheetHasLifecycles, businessObject.factSheetHasLifecycles) &&
Objects.equals(this.userSubscriptions, businessObject.userSubscriptions) &&
Objects.equals(this.factSheetHasPredecessors, businessObject.factSheetHasPredecessors) &&
Objects.equals(this.factSheetHasSuccessors, businessObject.factSheetHasSuccessors) &&
Objects.equals(this.factSheetHasRequires, businessObject.factSheetHasRequires) &&
Objects.equals(this.factSheetHasRequiredby, businessObject.factSheetHasRequiredby) &&
Objects.equals(this.serviceHasBusinessObjects, businessObject.serviceHasBusinessObjects) &&
Objects.equals(this.ifaceHasBusinessObjects, businessObject.ifaceHasBusinessObjects);
}
@Override
public int hashCode() {
return Objects.hash(ID, displayName, parentID, level, name, reference, alias, description, dataClassificationID, dataClassificationDescription, objectStatusID, tags, fullName, resourceType, completion, qualitySealExpiry, modificationTime, factSheetHasParents, factSheetHasChildren, factSheetHasDocuments, factSheetHasLifecycles, userSubscriptions, factSheetHasPredecessors, factSheetHasSuccessors, factSheetHasRequires, factSheetHasRequiredby, serviceHasBusinessObjects, ifaceHasBusinessObjects);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class BusinessObject {\n");
sb.append(" ID: ").append(toIndentedString(ID)).append("\n");
sb.append(" displayName: ").append(toIndentedString(displayName)).append("\n");
sb.append(" parentID: ").append(toIndentedString(parentID)).append("\n");
sb.append(" level: ").append(toIndentedString(level)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" reference: ").append(toIndentedString(reference)).append("\n");
sb.append(" alias: ").append(toIndentedString(alias)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" dataClassificationID: ").append(toIndentedString(dataClassificationID)).append("\n");
sb.append(" dataClassificationDescription: ").append(toIndentedString(dataClassificationDescription)).append("\n");
sb.append(" objectStatusID: ").append(toIndentedString(objectStatusID)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" fullName: ").append(toIndentedString(fullName)).append("\n");
sb.append(" resourceType: ").append(toIndentedString(resourceType)).append("\n");
sb.append(" completion: ").append(toIndentedString(completion)).append("\n");
sb.append(" qualitySealExpiry: ").append(toIndentedString(qualitySealExpiry)).append("\n");
sb.append(" modificationTime: ").append(toIndentedString(modificationTime)).append("\n");
sb.append(" factSheetHasParents: ").append(toIndentedString(factSheetHasParents)).append("\n");
sb.append(" factSheetHasChildren: ").append(toIndentedString(factSheetHasChildren)).append("\n");
sb.append(" factSheetHasDocuments: ").append(toIndentedString(factSheetHasDocuments)).append("\n");
sb.append(" factSheetHasLifecycles: ").append(toIndentedString(factSheetHasLifecycles)).append("\n");
sb.append(" userSubscriptions: ").append(toIndentedString(userSubscriptions)).append("\n");
sb.append(" factSheetHasPredecessors: ").append(toIndentedString(factSheetHasPredecessors)).append("\n");
sb.append(" factSheetHasSuccessors: ").append(toIndentedString(factSheetHasSuccessors)).append("\n");
sb.append(" factSheetHasRequires: ").append(toIndentedString(factSheetHasRequires)).append("\n");
sb.append(" factSheetHasRequiredby: ").append(toIndentedString(factSheetHasRequiredby)).append("\n");
sb.append(" serviceHasBusinessObjects: ").append(toIndentedString(serviceHasBusinessObjects)).append("\n");
sb.append(" ifaceHasBusinessObjects: ").append(toIndentedString(ifaceHasBusinessObjects)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy