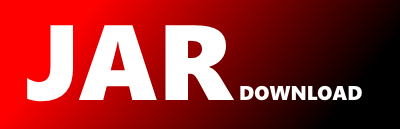
net.leanix.api.models.Report Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leanix-sdk-java Show documentation
Show all versions of leanix-sdk-java Show documentation
SDK for Java to access leanIX REST API
package net.leanix.api.models;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
import net.leanix.api.models.FactSheetHasChild;
import net.leanix.api.models.FactSheetHasDocument;
import net.leanix.api.models.FactSheetHasLifecycle;
import net.leanix.api.models.FactSheetHasParent;
import net.leanix.api.models.FactSheetHasPredecessor;
import net.leanix.api.models.FactSheetHasRequiredby;
import net.leanix.api.models.FactSheetHasRequires;
import net.leanix.api.models.FactSheetHasSuccessor;
import net.leanix.api.models.UserSubscription;
public class Report {
private String ID = null;
private String displayName = null;
private String parentID = null;
private Long level = null;
private String name = null;
private String reference = null;
private String alias = null;
private String description = null;
private String objectStatusID = null;
private List tags = new ArrayList();
private String fullName = null;
private String resourceType = null;
private String completion = null;
private String qualitySealExpiry = null;
private String modificationTime = null;
private List factSheetHasParents = new ArrayList();
private List factSheetHasChildren = new ArrayList();
private List factSheetHasDocuments = new ArrayList();
private List factSheetHasLifecycles = new ArrayList();
private List userSubscriptions = new ArrayList();
private List factSheetHasPredecessors = new ArrayList();
private List factSheetHasSuccessors = new ArrayList();
private List factSheetHasRequires = new ArrayList();
private List factSheetHasRequiredby = new ArrayList();
/**
**/
public Report ID(String ID) {
this.ID = ID;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("ID")
public String getID() {
return ID;
}
public void setID(String ID) {
this.ID = ID;
}
/**
**/
public Report displayName(String displayName) {
this.displayName = displayName;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("displayName")
public String getDisplayName() {
return displayName;
}
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
/**
**/
public Report parentID(String parentID) {
this.parentID = parentID;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("parentID")
public String getParentID() {
return parentID;
}
public void setParentID(String parentID) {
this.parentID = parentID;
}
/**
**/
public Report level(Long level) {
this.level = level;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("level")
public Long getLevel() {
return level;
}
public void setLevel(Long level) {
this.level = level;
}
/**
**/
public Report name(String name) {
this.name = name;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("name")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
/**
**/
public Report reference(String reference) {
this.reference = reference;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("reference")
public String getReference() {
return reference;
}
public void setReference(String reference) {
this.reference = reference;
}
/**
**/
public Report alias(String alias) {
this.alias = alias;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("alias")
public String getAlias() {
return alias;
}
public void setAlias(String alias) {
this.alias = alias;
}
/**
**/
public Report description(String description) {
this.description = description;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("description")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
/**
**/
public Report objectStatusID(String objectStatusID) {
this.objectStatusID = objectStatusID;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("objectStatusID")
public String getObjectStatusID() {
return objectStatusID;
}
public void setObjectStatusID(String objectStatusID) {
this.objectStatusID = objectStatusID;
}
/**
**/
public Report tags(List tags) {
this.tags = tags;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("tags")
public List getTags() {
return tags;
}
public void setTags(List tags) {
this.tags = tags;
}
/**
**/
public Report fullName(String fullName) {
this.fullName = fullName;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("fullName")
public String getFullName() {
return fullName;
}
public void setFullName(String fullName) {
this.fullName = fullName;
}
/**
**/
public Report resourceType(String resourceType) {
this.resourceType = resourceType;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("resourceType")
public String getResourceType() {
return resourceType;
}
public void setResourceType(String resourceType) {
this.resourceType = resourceType;
}
/**
**/
public Report completion(String completion) {
this.completion = completion;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("completion")
public String getCompletion() {
return completion;
}
public void setCompletion(String completion) {
this.completion = completion;
}
/**
**/
public Report qualitySealExpiry(String qualitySealExpiry) {
this.qualitySealExpiry = qualitySealExpiry;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("qualitySealExpiry")
public String getQualitySealExpiry() {
return qualitySealExpiry;
}
public void setQualitySealExpiry(String qualitySealExpiry) {
this.qualitySealExpiry = qualitySealExpiry;
}
/**
**/
public Report modificationTime(String modificationTime) {
this.modificationTime = modificationTime;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("modificationTime")
public String getModificationTime() {
return modificationTime;
}
public void setModificationTime(String modificationTime) {
this.modificationTime = modificationTime;
}
/**
**/
public Report factSheetHasParents(List factSheetHasParents) {
this.factSheetHasParents = factSheetHasParents;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasParents")
public List getFactSheetHasParents() {
return factSheetHasParents;
}
public void setFactSheetHasParents(List factSheetHasParents) {
this.factSheetHasParents = factSheetHasParents;
}
/**
**/
public Report factSheetHasChildren(List factSheetHasChildren) {
this.factSheetHasChildren = factSheetHasChildren;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasChildren")
public List getFactSheetHasChildren() {
return factSheetHasChildren;
}
public void setFactSheetHasChildren(List factSheetHasChildren) {
this.factSheetHasChildren = factSheetHasChildren;
}
/**
**/
public Report factSheetHasDocuments(List factSheetHasDocuments) {
this.factSheetHasDocuments = factSheetHasDocuments;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasDocuments")
public List getFactSheetHasDocuments() {
return factSheetHasDocuments;
}
public void setFactSheetHasDocuments(List factSheetHasDocuments) {
this.factSheetHasDocuments = factSheetHasDocuments;
}
/**
**/
public Report factSheetHasLifecycles(List factSheetHasLifecycles) {
this.factSheetHasLifecycles = factSheetHasLifecycles;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasLifecycles")
public List getFactSheetHasLifecycles() {
return factSheetHasLifecycles;
}
public void setFactSheetHasLifecycles(List factSheetHasLifecycles) {
this.factSheetHasLifecycles = factSheetHasLifecycles;
}
/**
**/
public Report userSubscriptions(List userSubscriptions) {
this.userSubscriptions = userSubscriptions;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("userSubscriptions")
public List getUserSubscriptions() {
return userSubscriptions;
}
public void setUserSubscriptions(List userSubscriptions) {
this.userSubscriptions = userSubscriptions;
}
/**
**/
public Report factSheetHasPredecessors(List factSheetHasPredecessors) {
this.factSheetHasPredecessors = factSheetHasPredecessors;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasPredecessors")
public List getFactSheetHasPredecessors() {
return factSheetHasPredecessors;
}
public void setFactSheetHasPredecessors(List factSheetHasPredecessors) {
this.factSheetHasPredecessors = factSheetHasPredecessors;
}
/**
**/
public Report factSheetHasSuccessors(List factSheetHasSuccessors) {
this.factSheetHasSuccessors = factSheetHasSuccessors;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasSuccessors")
public List getFactSheetHasSuccessors() {
return factSheetHasSuccessors;
}
public void setFactSheetHasSuccessors(List factSheetHasSuccessors) {
this.factSheetHasSuccessors = factSheetHasSuccessors;
}
/**
**/
public Report factSheetHasRequires(List factSheetHasRequires) {
this.factSheetHasRequires = factSheetHasRequires;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasRequires")
public List getFactSheetHasRequires() {
return factSheetHasRequires;
}
public void setFactSheetHasRequires(List factSheetHasRequires) {
this.factSheetHasRequires = factSheetHasRequires;
}
/**
**/
public Report factSheetHasRequiredby(List factSheetHasRequiredby) {
this.factSheetHasRequiredby = factSheetHasRequiredby;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasRequiredby")
public List getFactSheetHasRequiredby() {
return factSheetHasRequiredby;
}
public void setFactSheetHasRequiredby(List factSheetHasRequiredby) {
this.factSheetHasRequiredby = factSheetHasRequiredby;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Report report = (Report) o;
return Objects.equals(this.ID, report.ID) &&
Objects.equals(this.displayName, report.displayName) &&
Objects.equals(this.parentID, report.parentID) &&
Objects.equals(this.level, report.level) &&
Objects.equals(this.name, report.name) &&
Objects.equals(this.reference, report.reference) &&
Objects.equals(this.alias, report.alias) &&
Objects.equals(this.description, report.description) &&
Objects.equals(this.objectStatusID, report.objectStatusID) &&
Objects.equals(this.tags, report.tags) &&
Objects.equals(this.fullName, report.fullName) &&
Objects.equals(this.resourceType, report.resourceType) &&
Objects.equals(this.completion, report.completion) &&
Objects.equals(this.qualitySealExpiry, report.qualitySealExpiry) &&
Objects.equals(this.modificationTime, report.modificationTime) &&
Objects.equals(this.factSheetHasParents, report.factSheetHasParents) &&
Objects.equals(this.factSheetHasChildren, report.factSheetHasChildren) &&
Objects.equals(this.factSheetHasDocuments, report.factSheetHasDocuments) &&
Objects.equals(this.factSheetHasLifecycles, report.factSheetHasLifecycles) &&
Objects.equals(this.userSubscriptions, report.userSubscriptions) &&
Objects.equals(this.factSheetHasPredecessors, report.factSheetHasPredecessors) &&
Objects.equals(this.factSheetHasSuccessors, report.factSheetHasSuccessors) &&
Objects.equals(this.factSheetHasRequires, report.factSheetHasRequires) &&
Objects.equals(this.factSheetHasRequiredby, report.factSheetHasRequiredby);
}
@Override
public int hashCode() {
return Objects.hash(ID, displayName, parentID, level, name, reference, alias, description, objectStatusID, tags, fullName, resourceType, completion, qualitySealExpiry, modificationTime, factSheetHasParents, factSheetHasChildren, factSheetHasDocuments, factSheetHasLifecycles, userSubscriptions, factSheetHasPredecessors, factSheetHasSuccessors, factSheetHasRequires, factSheetHasRequiredby);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Report {\n");
sb.append(" ID: ").append(toIndentedString(ID)).append("\n");
sb.append(" displayName: ").append(toIndentedString(displayName)).append("\n");
sb.append(" parentID: ").append(toIndentedString(parentID)).append("\n");
sb.append(" level: ").append(toIndentedString(level)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" reference: ").append(toIndentedString(reference)).append("\n");
sb.append(" alias: ").append(toIndentedString(alias)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" objectStatusID: ").append(toIndentedString(objectStatusID)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" fullName: ").append(toIndentedString(fullName)).append("\n");
sb.append(" resourceType: ").append(toIndentedString(resourceType)).append("\n");
sb.append(" completion: ").append(toIndentedString(completion)).append("\n");
sb.append(" qualitySealExpiry: ").append(toIndentedString(qualitySealExpiry)).append("\n");
sb.append(" modificationTime: ").append(toIndentedString(modificationTime)).append("\n");
sb.append(" factSheetHasParents: ").append(toIndentedString(factSheetHasParents)).append("\n");
sb.append(" factSheetHasChildren: ").append(toIndentedString(factSheetHasChildren)).append("\n");
sb.append(" factSheetHasDocuments: ").append(toIndentedString(factSheetHasDocuments)).append("\n");
sb.append(" factSheetHasLifecycles: ").append(toIndentedString(factSheetHasLifecycles)).append("\n");
sb.append(" userSubscriptions: ").append(toIndentedString(userSubscriptions)).append("\n");
sb.append(" factSheetHasPredecessors: ").append(toIndentedString(factSheetHasPredecessors)).append("\n");
sb.append(" factSheetHasSuccessors: ").append(toIndentedString(factSheetHasSuccessors)).append("\n");
sb.append(" factSheetHasRequires: ").append(toIndentedString(factSheetHasRequires)).append("\n");
sb.append(" factSheetHasRequiredby: ").append(toIndentedString(factSheetHasRequiredby)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy