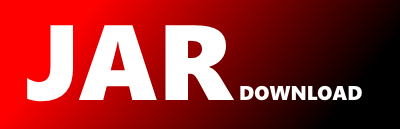
net.leanix.api.models.Provider Maven / Gradle / Ivy
package net.leanix.api.models;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
import net.leanix.api.models.FactSheetHasChild;
import net.leanix.api.models.FactSheetHasDocument;
import net.leanix.api.models.FactSheetHasLifecycle;
import net.leanix.api.models.FactSheetHasParent;
import net.leanix.api.models.FactSheetHasPredecessor;
import net.leanix.api.models.FactSheetHasRequiredby;
import net.leanix.api.models.FactSheetHasRequires;
import net.leanix.api.models.FactSheetHasSuccessor;
import net.leanix.api.models.ProjectHasProvider;
import net.leanix.api.models.ResourceHasProvider;
import net.leanix.api.models.UserSubscription;
public class Provider {
private String ID = null;
private String displayName = null;
private String parentID = null;
private Long level = null;
private String name = null;
private String reference = null;
private String alias = null;
private String description = null;
private String providerCriticalityID = null;
private String providerCriticalityDescription = null;
private String providerQualityID = null;
private String providerQualityDescription = null;
private String objectStatusID = null;
private List tags = new ArrayList();
private String fullName = null;
private String resourceType = null;
private String completion = null;
private String qualitySealExpiry = null;
private String modificationTime = null;
private List factSheetHasParents = new ArrayList();
private List factSheetHasChildren = new ArrayList();
private List factSheetHasDocuments = new ArrayList();
private List factSheetHasLifecycles = new ArrayList();
private List userSubscriptions = new ArrayList();
private List factSheetHasPredecessors = new ArrayList();
private List factSheetHasSuccessors = new ArrayList();
private List factSheetHasRequires = new ArrayList();
private List factSheetHasRequiredby = new ArrayList();
private List resourceHasProviders = new ArrayList();
private List projectHasProviders = new ArrayList();
/**
**/
public Provider ID(String ID) {
this.ID = ID;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("ID")
public String getID() {
return ID;
}
public void setID(String ID) {
this.ID = ID;
}
/**
**/
public Provider displayName(String displayName) {
this.displayName = displayName;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("displayName")
public String getDisplayName() {
return displayName;
}
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
/**
**/
public Provider parentID(String parentID) {
this.parentID = parentID;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("parentID")
public String getParentID() {
return parentID;
}
public void setParentID(String parentID) {
this.parentID = parentID;
}
/**
**/
public Provider level(Long level) {
this.level = level;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("level")
public Long getLevel() {
return level;
}
public void setLevel(Long level) {
this.level = level;
}
/**
**/
public Provider name(String name) {
this.name = name;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("name")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
/**
**/
public Provider reference(String reference) {
this.reference = reference;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("reference")
public String getReference() {
return reference;
}
public void setReference(String reference) {
this.reference = reference;
}
/**
**/
public Provider alias(String alias) {
this.alias = alias;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("alias")
public String getAlias() {
return alias;
}
public void setAlias(String alias) {
this.alias = alias;
}
/**
**/
public Provider description(String description) {
this.description = description;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("description")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
/**
**/
public Provider providerCriticalityID(String providerCriticalityID) {
this.providerCriticalityID = providerCriticalityID;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("providerCriticalityID")
public String getProviderCriticalityID() {
return providerCriticalityID;
}
public void setProviderCriticalityID(String providerCriticalityID) {
this.providerCriticalityID = providerCriticalityID;
}
/**
**/
public Provider providerCriticalityDescription(String providerCriticalityDescription) {
this.providerCriticalityDescription = providerCriticalityDescription;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("providerCriticalityDescription")
public String getProviderCriticalityDescription() {
return providerCriticalityDescription;
}
public void setProviderCriticalityDescription(String providerCriticalityDescription) {
this.providerCriticalityDescription = providerCriticalityDescription;
}
/**
**/
public Provider providerQualityID(String providerQualityID) {
this.providerQualityID = providerQualityID;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("providerQualityID")
public String getProviderQualityID() {
return providerQualityID;
}
public void setProviderQualityID(String providerQualityID) {
this.providerQualityID = providerQualityID;
}
/**
**/
public Provider providerQualityDescription(String providerQualityDescription) {
this.providerQualityDescription = providerQualityDescription;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("providerQualityDescription")
public String getProviderQualityDescription() {
return providerQualityDescription;
}
public void setProviderQualityDescription(String providerQualityDescription) {
this.providerQualityDescription = providerQualityDescription;
}
/**
**/
public Provider objectStatusID(String objectStatusID) {
this.objectStatusID = objectStatusID;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("objectStatusID")
public String getObjectStatusID() {
return objectStatusID;
}
public void setObjectStatusID(String objectStatusID) {
this.objectStatusID = objectStatusID;
}
/**
**/
public Provider tags(List tags) {
this.tags = tags;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("tags")
public List getTags() {
return tags;
}
public void setTags(List tags) {
this.tags = tags;
}
/**
**/
public Provider fullName(String fullName) {
this.fullName = fullName;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("fullName")
public String getFullName() {
return fullName;
}
public void setFullName(String fullName) {
this.fullName = fullName;
}
/**
**/
public Provider resourceType(String resourceType) {
this.resourceType = resourceType;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("resourceType")
public String getResourceType() {
return resourceType;
}
public void setResourceType(String resourceType) {
this.resourceType = resourceType;
}
/**
**/
public Provider completion(String completion) {
this.completion = completion;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("completion")
public String getCompletion() {
return completion;
}
public void setCompletion(String completion) {
this.completion = completion;
}
/**
**/
public Provider qualitySealExpiry(String qualitySealExpiry) {
this.qualitySealExpiry = qualitySealExpiry;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("qualitySealExpiry")
public String getQualitySealExpiry() {
return qualitySealExpiry;
}
public void setQualitySealExpiry(String qualitySealExpiry) {
this.qualitySealExpiry = qualitySealExpiry;
}
/**
**/
public Provider modificationTime(String modificationTime) {
this.modificationTime = modificationTime;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("modificationTime")
public String getModificationTime() {
return modificationTime;
}
public void setModificationTime(String modificationTime) {
this.modificationTime = modificationTime;
}
/**
**/
public Provider factSheetHasParents(List factSheetHasParents) {
this.factSheetHasParents = factSheetHasParents;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasParents")
public List getFactSheetHasParents() {
return factSheetHasParents;
}
public void setFactSheetHasParents(List factSheetHasParents) {
this.factSheetHasParents = factSheetHasParents;
}
/**
**/
public Provider factSheetHasChildren(List factSheetHasChildren) {
this.factSheetHasChildren = factSheetHasChildren;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasChildren")
public List getFactSheetHasChildren() {
return factSheetHasChildren;
}
public void setFactSheetHasChildren(List factSheetHasChildren) {
this.factSheetHasChildren = factSheetHasChildren;
}
/**
**/
public Provider factSheetHasDocuments(List factSheetHasDocuments) {
this.factSheetHasDocuments = factSheetHasDocuments;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasDocuments")
public List getFactSheetHasDocuments() {
return factSheetHasDocuments;
}
public void setFactSheetHasDocuments(List factSheetHasDocuments) {
this.factSheetHasDocuments = factSheetHasDocuments;
}
/**
**/
public Provider factSheetHasLifecycles(List factSheetHasLifecycles) {
this.factSheetHasLifecycles = factSheetHasLifecycles;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasLifecycles")
public List getFactSheetHasLifecycles() {
return factSheetHasLifecycles;
}
public void setFactSheetHasLifecycles(List factSheetHasLifecycles) {
this.factSheetHasLifecycles = factSheetHasLifecycles;
}
/**
**/
public Provider userSubscriptions(List userSubscriptions) {
this.userSubscriptions = userSubscriptions;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("userSubscriptions")
public List getUserSubscriptions() {
return userSubscriptions;
}
public void setUserSubscriptions(List userSubscriptions) {
this.userSubscriptions = userSubscriptions;
}
/**
**/
public Provider factSheetHasPredecessors(List factSheetHasPredecessors) {
this.factSheetHasPredecessors = factSheetHasPredecessors;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasPredecessors")
public List getFactSheetHasPredecessors() {
return factSheetHasPredecessors;
}
public void setFactSheetHasPredecessors(List factSheetHasPredecessors) {
this.factSheetHasPredecessors = factSheetHasPredecessors;
}
/**
**/
public Provider factSheetHasSuccessors(List factSheetHasSuccessors) {
this.factSheetHasSuccessors = factSheetHasSuccessors;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasSuccessors")
public List getFactSheetHasSuccessors() {
return factSheetHasSuccessors;
}
public void setFactSheetHasSuccessors(List factSheetHasSuccessors) {
this.factSheetHasSuccessors = factSheetHasSuccessors;
}
/**
**/
public Provider factSheetHasRequires(List factSheetHasRequires) {
this.factSheetHasRequires = factSheetHasRequires;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasRequires")
public List getFactSheetHasRequires() {
return factSheetHasRequires;
}
public void setFactSheetHasRequires(List factSheetHasRequires) {
this.factSheetHasRequires = factSheetHasRequires;
}
/**
**/
public Provider factSheetHasRequiredby(List factSheetHasRequiredby) {
this.factSheetHasRequiredby = factSheetHasRequiredby;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("factSheetHasRequiredby")
public List getFactSheetHasRequiredby() {
return factSheetHasRequiredby;
}
public void setFactSheetHasRequiredby(List factSheetHasRequiredby) {
this.factSheetHasRequiredby = factSheetHasRequiredby;
}
/**
**/
public Provider resourceHasProviders(List resourceHasProviders) {
this.resourceHasProviders = resourceHasProviders;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("resourceHasProviders")
public List getResourceHasProviders() {
return resourceHasProviders;
}
public void setResourceHasProviders(List resourceHasProviders) {
this.resourceHasProviders = resourceHasProviders;
}
/**
**/
public Provider projectHasProviders(List projectHasProviders) {
this.projectHasProviders = projectHasProviders;
return this;
}
@ApiModelProperty(example = "null", value = "")
@JsonProperty("projectHasProviders")
public List getProjectHasProviders() {
return projectHasProviders;
}
public void setProjectHasProviders(List projectHasProviders) {
this.projectHasProviders = projectHasProviders;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Provider provider = (Provider) o;
return Objects.equals(this.ID, provider.ID) &&
Objects.equals(this.displayName, provider.displayName) &&
Objects.equals(this.parentID, provider.parentID) &&
Objects.equals(this.level, provider.level) &&
Objects.equals(this.name, provider.name) &&
Objects.equals(this.reference, provider.reference) &&
Objects.equals(this.alias, provider.alias) &&
Objects.equals(this.description, provider.description) &&
Objects.equals(this.providerCriticalityID, provider.providerCriticalityID) &&
Objects.equals(this.providerCriticalityDescription, provider.providerCriticalityDescription) &&
Objects.equals(this.providerQualityID, provider.providerQualityID) &&
Objects.equals(this.providerQualityDescription, provider.providerQualityDescription) &&
Objects.equals(this.objectStatusID, provider.objectStatusID) &&
Objects.equals(this.tags, provider.tags) &&
Objects.equals(this.fullName, provider.fullName) &&
Objects.equals(this.resourceType, provider.resourceType) &&
Objects.equals(this.completion, provider.completion) &&
Objects.equals(this.qualitySealExpiry, provider.qualitySealExpiry) &&
Objects.equals(this.modificationTime, provider.modificationTime) &&
Objects.equals(this.factSheetHasParents, provider.factSheetHasParents) &&
Objects.equals(this.factSheetHasChildren, provider.factSheetHasChildren) &&
Objects.equals(this.factSheetHasDocuments, provider.factSheetHasDocuments) &&
Objects.equals(this.factSheetHasLifecycles, provider.factSheetHasLifecycles) &&
Objects.equals(this.userSubscriptions, provider.userSubscriptions) &&
Objects.equals(this.factSheetHasPredecessors, provider.factSheetHasPredecessors) &&
Objects.equals(this.factSheetHasSuccessors, provider.factSheetHasSuccessors) &&
Objects.equals(this.factSheetHasRequires, provider.factSheetHasRequires) &&
Objects.equals(this.factSheetHasRequiredby, provider.factSheetHasRequiredby) &&
Objects.equals(this.resourceHasProviders, provider.resourceHasProviders) &&
Objects.equals(this.projectHasProviders, provider.projectHasProviders);
}
@Override
public int hashCode() {
return Objects.hash(ID, displayName, parentID, level, name, reference, alias, description, providerCriticalityID, providerCriticalityDescription, providerQualityID, providerQualityDescription, objectStatusID, tags, fullName, resourceType, completion, qualitySealExpiry, modificationTime, factSheetHasParents, factSheetHasChildren, factSheetHasDocuments, factSheetHasLifecycles, userSubscriptions, factSheetHasPredecessors, factSheetHasSuccessors, factSheetHasRequires, factSheetHasRequiredby, resourceHasProviders, projectHasProviders);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Provider {\n");
sb.append(" ID: ").append(toIndentedString(ID)).append("\n");
sb.append(" displayName: ").append(toIndentedString(displayName)).append("\n");
sb.append(" parentID: ").append(toIndentedString(parentID)).append("\n");
sb.append(" level: ").append(toIndentedString(level)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" reference: ").append(toIndentedString(reference)).append("\n");
sb.append(" alias: ").append(toIndentedString(alias)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" providerCriticalityID: ").append(toIndentedString(providerCriticalityID)).append("\n");
sb.append(" providerCriticalityDescription: ").append(toIndentedString(providerCriticalityDescription)).append("\n");
sb.append(" providerQualityID: ").append(toIndentedString(providerQualityID)).append("\n");
sb.append(" providerQualityDescription: ").append(toIndentedString(providerQualityDescription)).append("\n");
sb.append(" objectStatusID: ").append(toIndentedString(objectStatusID)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" fullName: ").append(toIndentedString(fullName)).append("\n");
sb.append(" resourceType: ").append(toIndentedString(resourceType)).append("\n");
sb.append(" completion: ").append(toIndentedString(completion)).append("\n");
sb.append(" qualitySealExpiry: ").append(toIndentedString(qualitySealExpiry)).append("\n");
sb.append(" modificationTime: ").append(toIndentedString(modificationTime)).append("\n");
sb.append(" factSheetHasParents: ").append(toIndentedString(factSheetHasParents)).append("\n");
sb.append(" factSheetHasChildren: ").append(toIndentedString(factSheetHasChildren)).append("\n");
sb.append(" factSheetHasDocuments: ").append(toIndentedString(factSheetHasDocuments)).append("\n");
sb.append(" factSheetHasLifecycles: ").append(toIndentedString(factSheetHasLifecycles)).append("\n");
sb.append(" userSubscriptions: ").append(toIndentedString(userSubscriptions)).append("\n");
sb.append(" factSheetHasPredecessors: ").append(toIndentedString(factSheetHasPredecessors)).append("\n");
sb.append(" factSheetHasSuccessors: ").append(toIndentedString(factSheetHasSuccessors)).append("\n");
sb.append(" factSheetHasRequires: ").append(toIndentedString(factSheetHasRequires)).append("\n");
sb.append(" factSheetHasRequiredby: ").append(toIndentedString(factSheetHasRequiredby)).append("\n");
sb.append(" resourceHasProviders: ").append(toIndentedString(resourceHasProviders)).append("\n");
sb.append(" projectHasProviders: ").append(toIndentedString(projectHasProviders)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy