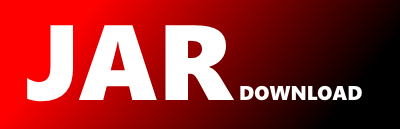
net.leanix.api.models.TodoData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leanix-sdk-java Show documentation
Show all versions of leanix-sdk-java Show documentation
SDK for Java to access leanIX REST API
/*
* LeanIX Pathfinder REST API
* Core application for storage and analysis of IT landscape data
*
* OpenAPI spec version: 4.0.217
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package net.leanix.api.models;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonSubTypes;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.time.LocalDate;
import java.time.OffsetDateTime;
import java.util.UUID;
/**
* TodoData
*/
@JsonTypeInfo(use = JsonTypeInfo.Id.NAME, include = JsonTypeInfo.As.PROPERTY, property = "type", visible = true )
@JsonSubTypes({
@JsonSubTypes.Type(value = InactiveUserTodoData.class, name = "InactiveUserTodoData"),
@JsonSubTypes.Type(value = OpenCommentTodoData.class, name = "OpenCommentTodoData"),
@JsonSubTypes.Type(value = RelationRecommendationTodoData.class, name = "RelationRecommendationTodoData"),
@JsonSubTypes.Type(value = SurveyResponseTodoData.class, name = "SurveyResponseTodoData"),
@JsonSubTypes.Type(value = FactSheetTodoData.class, name = "FactSheetTodoData"),
})
public class TodoData {
@JsonProperty("id")
private UUID id = null;
@JsonProperty("userId")
private UUID userId = null;
/**
* Gets or Sets status
*/
public enum StatusEnum {
OPEN("OPEN"),
DISMISSED("DISMISSED"),
DONE("DONE");
private String value;
StatusEnum(String value) {
this.value = value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static StatusEnum fromValue(String text) {
for (StatusEnum b : StatusEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
}
@JsonProperty("status")
private StatusEnum status = null;
@JsonProperty("dueAt")
private LocalDate dueAt = null;
@JsonProperty("createdAt")
private OffsetDateTime createdAt = null;
@JsonProperty("updatedAt")
private OffsetDateTime updatedAt = null;
@JsonProperty("priority")
private Double priority = null;
@JsonProperty("updatedBy")
private UUID updatedBy = null;
@JsonProperty("type")
private String type = null;
public TodoData id(UUID id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@ApiModelProperty(example = "null", value = "")
public UUID getId() {
return id;
}
public void setId(UUID id) {
this.id = id;
}
public TodoData userId(UUID userId) {
this.userId = userId;
return this;
}
/**
* Get userId
* @return userId
**/
@ApiModelProperty(example = "null", required = true, value = "")
public UUID getUserId() {
return userId;
}
public void setUserId(UUID userId) {
this.userId = userId;
}
public TodoData status(StatusEnum status) {
this.status = status;
return this;
}
/**
* Get status
* @return status
**/
@ApiModelProperty(example = "null", value = "")
public StatusEnum getStatus() {
return status;
}
public void setStatus(StatusEnum status) {
this.status = status;
}
public TodoData dueAt(LocalDate dueAt) {
this.dueAt = dueAt;
return this;
}
/**
* Get dueAt
* @return dueAt
**/
@ApiModelProperty(example = "null", value = "")
public LocalDate getDueAt() {
return dueAt;
}
public void setDueAt(LocalDate dueAt) {
this.dueAt = dueAt;
}
public TodoData createdAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* Get createdAt
* @return createdAt
**/
@ApiModelProperty(example = "null", value = "")
public OffsetDateTime getCreatedAt() {
return createdAt;
}
public void setCreatedAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
}
public TodoData updatedAt(OffsetDateTime updatedAt) {
this.updatedAt = updatedAt;
return this;
}
/**
* Get updatedAt
* @return updatedAt
**/
@ApiModelProperty(example = "null", value = "")
public OffsetDateTime getUpdatedAt() {
return updatedAt;
}
public void setUpdatedAt(OffsetDateTime updatedAt) {
this.updatedAt = updatedAt;
}
public TodoData priority(Double priority) {
this.priority = priority;
return this;
}
/**
* Get priority
* @return priority
**/
@ApiModelProperty(example = "null", value = "")
public Double getPriority() {
return priority;
}
public void setPriority(Double priority) {
this.priority = priority;
}
public TodoData updatedBy(UUID updatedBy) {
this.updatedBy = updatedBy;
return this;
}
/**
* Get updatedBy
* @return updatedBy
**/
@ApiModelProperty(example = "null", value = "")
public UUID getUpdatedBy() {
return updatedBy;
}
public void setUpdatedBy(UUID updatedBy) {
this.updatedBy = updatedBy;
}
/**
* Get type
* @return type
**/
@ApiModelProperty(example = "null", value = "")
public String getType() {
return type;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TodoData todoData = (TodoData) o;
return Objects.equals(this.id, todoData.id) &&
Objects.equals(this.userId, todoData.userId) &&
Objects.equals(this.status, todoData.status) &&
Objects.equals(this.dueAt, todoData.dueAt) &&
Objects.equals(this.createdAt, todoData.createdAt) &&
Objects.equals(this.updatedAt, todoData.updatedAt) &&
Objects.equals(this.priority, todoData.priority) &&
Objects.equals(this.updatedBy, todoData.updatedBy) &&
Objects.equals(this.type, todoData.type);
}
@Override
public int hashCode() {
return Objects.hash(id, userId, status, dueAt, createdAt, updatedAt, priority, updatedBy, type);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TodoData {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" userId: ").append(toIndentedString(userId)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" dueAt: ").append(toIndentedString(dueAt)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" updatedAt: ").append(toIndentedString(updatedAt)).append("\n");
sb.append(" priority: ").append(toIndentedString(priority)).append("\n");
sb.append(" updatedBy: ").append(toIndentedString(updatedBy)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy