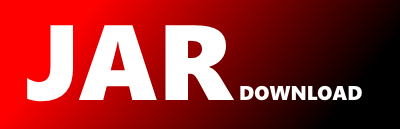
net.leanix.api.models.MetaSection Maven / Gradle / Ivy
/*
* Pathfinder
* Core application for storage and analysis of IT landscape data
*
* OpenAPI spec version: 5.0.813
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package net.leanix.api.models;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import net.leanix.api.models.MetaItemTranslation;
import net.leanix.api.models.MetaSubsection;
/**
* MetaSection
*/
public class MetaSection {
@JsonProperty("key")
private String key = null;
@JsonProperty("weight")
private Double weight = null;
@JsonProperty("subsections")
private List subsections = new ArrayList();
@JsonProperty("translations")
private Map translations = new HashMap();
@JsonProperty("visible")
private Boolean visible = false;
public MetaSection key(String key) {
this.key = key;
return this;
}
/**
* Get key
* @return key
**/
@ApiModelProperty(example = "null", value = "")
public String getKey() {
return key;
}
public void setKey(String key) {
this.key = key;
}
public MetaSection weight(Double weight) {
this.weight = weight;
return this;
}
/**
* Get weight
* @return weight
**/
@ApiModelProperty(example = "null", value = "")
public Double getWeight() {
return weight;
}
public void setWeight(Double weight) {
this.weight = weight;
}
public MetaSection subsections(List subsections) {
this.subsections = subsections;
return this;
}
public MetaSection addSubsectionsItem(MetaSubsection subsectionsItem) {
this.subsections.add(subsectionsItem);
return this;
}
/**
* Get subsections
* @return subsections
**/
@ApiModelProperty(example = "null", value = "")
public List getSubsections() {
return subsections;
}
public void setSubsections(List subsections) {
this.subsections = subsections;
}
public MetaSection translations(Map translations) {
this.translations = translations;
return this;
}
public MetaSection putTranslationsItem(String key, MetaItemTranslation translationsItem) {
this.translations.put(key, translationsItem);
return this;
}
/**
* Get translations
* @return translations
**/
@ApiModelProperty(example = "null", value = "")
public Map getTranslations() {
return translations;
}
public void setTranslations(Map translations) {
this.translations = translations;
}
public MetaSection visible(Boolean visible) {
this.visible = visible;
return this;
}
/**
* Get visible
* @return visible
**/
@ApiModelProperty(example = "null", value = "")
public Boolean getVisible() {
return visible;
}
public void setVisible(Boolean visible) {
this.visible = visible;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
MetaSection metaSection = (MetaSection) o;
return Objects.equals(this.key, metaSection.key) &&
Objects.equals(this.weight, metaSection.weight) &&
Objects.equals(this.subsections, metaSection.subsections) &&
Objects.equals(this.translations, metaSection.translations) &&
Objects.equals(this.visible, metaSection.visible);
}
@Override
public int hashCode() {
return Objects.hash(key, weight, subsections, translations, visible);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class MetaSection {\n");
sb.append(" key: ").append(toIndentedString(key)).append("\n");
sb.append(" weight: ").append(toIndentedString(weight)).append("\n");
sb.append(" subsections: ").append(toIndentedString(subsections)).append("\n");
sb.append(" translations: ").append(toIndentedString(translations)).append("\n");
sb.append(" visible: ").append(toIndentedString(visible)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy