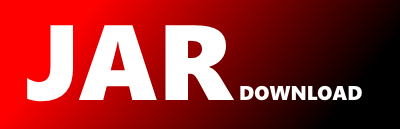
net.leanix.api.models.UpdateViewActionPayload Maven / Gradle / Ivy
/*
* Pathfinder
* Core application for storage and analysis of IT landscape data
*
* OpenAPI spec version: 5.0.813
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package net.leanix.api.models;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
import net.leanix.api.models.FacetViewState;
import net.leanix.api.models.MetaData;
/**
* UpdateViewActionPayload
*/
public class UpdateViewActionPayload {
@JsonProperty("renderType")
private String renderType = null;
@JsonProperty("readOnly")
private Boolean readOnly = false;
@JsonProperty("visible")
private Boolean visible = false;
@JsonProperty("weight")
private Double weight = null;
@JsonProperty("size")
private Integer size = null;
@JsonProperty("onTheFlyCreation")
private Boolean onTheFlyCreation = false;
@JsonProperty("bgColor")
private String bgColor = null;
@JsonProperty("metadata")
private MetaData metadata = null;
@JsonProperty("facetViewStates")
private List facetViewStates = new ArrayList();
@JsonProperty("hiddenForRoles")
private List hiddenForRoles = new ArrayList();
public UpdateViewActionPayload renderType(String renderType) {
this.renderType = renderType;
return this;
}
/**
* Get renderType
* @return renderType
**/
@ApiModelProperty(example = "null", value = "")
public String getRenderType() {
return renderType;
}
public void setRenderType(String renderType) {
this.renderType = renderType;
}
public UpdateViewActionPayload readOnly(Boolean readOnly) {
this.readOnly = readOnly;
return this;
}
/**
* Get readOnly
* @return readOnly
**/
@ApiModelProperty(example = "null", value = "")
public Boolean getReadOnly() {
return readOnly;
}
public void setReadOnly(Boolean readOnly) {
this.readOnly = readOnly;
}
public UpdateViewActionPayload visible(Boolean visible) {
this.visible = visible;
return this;
}
/**
* Get visible
* @return visible
**/
@ApiModelProperty(example = "null", value = "")
public Boolean getVisible() {
return visible;
}
public void setVisible(Boolean visible) {
this.visible = visible;
}
public UpdateViewActionPayload weight(Double weight) {
this.weight = weight;
return this;
}
/**
* Get weight
* @return weight
**/
@ApiModelProperty(example = "null", value = "")
public Double getWeight() {
return weight;
}
public void setWeight(Double weight) {
this.weight = weight;
}
public UpdateViewActionPayload size(Integer size) {
this.size = size;
return this;
}
/**
* Get size
* minimum: 1
* maximum: 12
* @return size
**/
@ApiModelProperty(example = "null", value = "")
public Integer getSize() {
return size;
}
public void setSize(Integer size) {
this.size = size;
}
public UpdateViewActionPayload onTheFlyCreation(Boolean onTheFlyCreation) {
this.onTheFlyCreation = onTheFlyCreation;
return this;
}
/**
* Get onTheFlyCreation
* @return onTheFlyCreation
**/
@ApiModelProperty(example = "null", value = "")
public Boolean getOnTheFlyCreation() {
return onTheFlyCreation;
}
public void setOnTheFlyCreation(Boolean onTheFlyCreation) {
this.onTheFlyCreation = onTheFlyCreation;
}
public UpdateViewActionPayload bgColor(String bgColor) {
this.bgColor = bgColor;
return this;
}
/**
* Get bgColor
* @return bgColor
**/
@ApiModelProperty(example = "null", value = "")
public String getBgColor() {
return bgColor;
}
public void setBgColor(String bgColor) {
this.bgColor = bgColor;
}
public UpdateViewActionPayload metadata(MetaData metadata) {
this.metadata = metadata;
return this;
}
/**
* Get metadata
* @return metadata
**/
@ApiModelProperty(example = "null", value = "")
public MetaData getMetadata() {
return metadata;
}
public void setMetadata(MetaData metadata) {
this.metadata = metadata;
}
public UpdateViewActionPayload facetViewStates(List facetViewStates) {
this.facetViewStates = facetViewStates;
return this;
}
public UpdateViewActionPayload addFacetViewStatesItem(FacetViewState facetViewStatesItem) {
this.facetViewStates.add(facetViewStatesItem);
return this;
}
/**
* Get facetViewStates
* @return facetViewStates
**/
@ApiModelProperty(example = "null", value = "")
public List getFacetViewStates() {
return facetViewStates;
}
public void setFacetViewStates(List facetViewStates) {
this.facetViewStates = facetViewStates;
}
public UpdateViewActionPayload hiddenForRoles(List hiddenForRoles) {
this.hiddenForRoles = hiddenForRoles;
return this;
}
public UpdateViewActionPayload addHiddenForRolesItem(String hiddenForRolesItem) {
this.hiddenForRoles.add(hiddenForRolesItem);
return this;
}
/**
* Get hiddenForRoles
* @return hiddenForRoles
**/
@ApiModelProperty(example = "null", value = "")
public List getHiddenForRoles() {
return hiddenForRoles;
}
public void setHiddenForRoles(List hiddenForRoles) {
this.hiddenForRoles = hiddenForRoles;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
UpdateViewActionPayload updateViewActionPayload = (UpdateViewActionPayload) o;
return Objects.equals(this.renderType, updateViewActionPayload.renderType) &&
Objects.equals(this.readOnly, updateViewActionPayload.readOnly) &&
Objects.equals(this.visible, updateViewActionPayload.visible) &&
Objects.equals(this.weight, updateViewActionPayload.weight) &&
Objects.equals(this.size, updateViewActionPayload.size) &&
Objects.equals(this.onTheFlyCreation, updateViewActionPayload.onTheFlyCreation) &&
Objects.equals(this.bgColor, updateViewActionPayload.bgColor) &&
Objects.equals(this.metadata, updateViewActionPayload.metadata) &&
Objects.equals(this.facetViewStates, updateViewActionPayload.facetViewStates) &&
Objects.equals(this.hiddenForRoles, updateViewActionPayload.hiddenForRoles);
}
@Override
public int hashCode() {
return Objects.hash(renderType, readOnly, visible, weight, size, onTheFlyCreation, bgColor, metadata, facetViewStates, hiddenForRoles);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class UpdateViewActionPayload {\n");
sb.append(" renderType: ").append(toIndentedString(renderType)).append("\n");
sb.append(" readOnly: ").append(toIndentedString(readOnly)).append("\n");
sb.append(" visible: ").append(toIndentedString(visible)).append("\n");
sb.append(" weight: ").append(toIndentedString(weight)).append("\n");
sb.append(" size: ").append(toIndentedString(size)).append("\n");
sb.append(" onTheFlyCreation: ").append(toIndentedString(onTheFlyCreation)).append("\n");
sb.append(" bgColor: ").append(toIndentedString(bgColor)).append("\n");
sb.append(" metadata: ").append(toIndentedString(metadata)).append("\n");
sb.append(" facetViewStates: ").append(toIndentedString(facetViewStates)).append("\n");
sb.append(" hiddenForRoles: ").append(toIndentedString(hiddenForRoles)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy