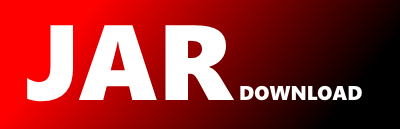
net.leanix.gsit.EventHandler Maven / Gradle / Ivy
package net.leanix.gsit;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.node.ObjectNode;
import com.google.common.base.Charsets;
import com.google.common.base.Optional;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Iterables;
import com.google.common.collect.Maps;
import com.google.common.io.Resources;
import java.io.IOException;
import java.util.AbstractMap;
import java.util.Map;
public class EventHandler {
private EventProcessor eventProcessor;
public EventHandler() throws IOException {
ObjectMapper objectMapper = new ObjectMapper();
Map eventGroupAssignment = readEventGroups(objectMapper);
Map eventSchemas = readEventSchemas(objectMapper);
this.eventProcessor = new EventProcessor(eventGroupAssignment, eventSchemas, objectMapper);
}
public ObjectNode handle(ObjectNode input) throws UnknownEventGroupException, UnknownEventSchemaException, FirehoseEncodingException, MissingAttributeException, MalformedAttributeException {
return eventProcessor.cleanData(input);
}
private static Map readEventGroups(ObjectMapper objectMapper) throws IOException {
String eventGroupsJson = Resources.toString(
Resources.getResource("event_groups.json"),
Charsets.UTF_8
);
JsonNode eventGroups = Utils.parseJson(eventGroupsJson, objectMapper);
Map eventGroupsRawMap = objectMapper.convertValue(eventGroups, Map.class);
return ImmutableMap.copyOf(
Iterables.transform(eventGroupsRawMap.entrySet(), entry -> {
EventType eventType = new EventType(entry.getKey());
EventGroup eventGroup = new EventGroup((String) entry.getValue());
return new AbstractMap.SimpleEntry<>(eventType, eventGroup);
})
);
}
private static Map readEventSchemas(ObjectMapper objectMapper) throws IOException {
String eventSchemasJson = Resources.toString(
Resources.getResource("input_schema.json"),
Charsets.UTF_8
);
JsonNode eventSchemas = Utils.parseJson(eventSchemasJson, objectMapper);
Map>> eventSchemasRawMap = objectMapper.convertValue(eventSchemas, Map.class);
Iterable> eventSchemasEntries = Iterables.transform(eventSchemasRawMap.entrySet(), entry -> {
EventGroup eventGroup = new EventGroup(entry.getKey());
Map attributes = Maps.transformValues(
entry.getValue(),
jsonMap -> {
String path = (String) jsonMap.get("path");
Optional isRequired = Optional.fromNullable((Boolean) jsonMap.get("isRequired"));
Optional isHashed = Optional.fromNullable((Boolean) jsonMap.get("isHashed"));
return new EventAttributeDefinition(path, isRequired, isHashed);
}
);
EventSchema eventSchema = new EventSchema(attributes.values());
return new AbstractMap.SimpleEntry<>(eventGroup, eventSchema);
});
return ImmutableMap.copyOf(eventSchemasEntries);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy