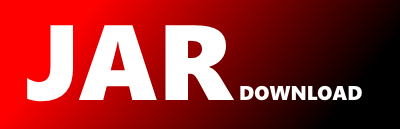
net.leanix.gsit.EventProcessor Maven / Gradle / Ivy
package net.leanix.gsit;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.node.NullNode;
import com.fasterxml.jackson.databind.node.ObjectNode;
import com.google.common.base.Optional;
import java.io.UnsupportedEncodingException;
import java.util.Map;
public class EventProcessor {
private final Map eventGroupAssignment;
private final Map eventSchemas;
private final ObjectMapper objectMapper;
public EventProcessor(
Map eventGroupAssignment,
Map eventSchemas,
ObjectMapper objectMapper
) {
this.eventGroupAssignment = eventGroupAssignment;
this.eventSchemas = eventSchemas;
this.objectMapper = objectMapper;
}
public ObjectNode cleanData(ObjectNode input) throws UnknownEventGroupException, UnknownEventSchemaException, FirehoseEncodingException, MissingAttributeException, MalformedAttributeException {
EventGroup eventGroup = assignEventGroup(input, eventGroupAssignment);
EventSchema eventSchema = lookupEventSchema(eventGroup, eventSchemas);
ObjectNode cleanedEvent = cleanEvent(input, eventSchema, objectMapper);
return encodeForFirehose(cleanedEvent, eventGroup, objectMapper);
}
private static EventGroup assignEventGroup(ObjectNode input, Map eventGroupAssignment) throws UnknownEventGroupException, MissingAttributeException, MalformedAttributeException {
EventType eventType = new EventType(
Utils.getAttributeAccordingToDefinition(
input,
new EventAttributeDefinition("type", Optional.of(true), Optional.of(false))
).get().asText()
);
return Utils.getOrThrow(
eventGroupAssignment, eventType,
() -> new UnknownEventGroupException(eventType)
);
}
private static EventSchema lookupEventSchema(EventGroup eventGroup, Map eventSchemas) throws UnknownEventSchemaException {
return Utils.getOrThrow(
eventSchemas, eventGroup,
() -> new UnknownEventSchemaException(eventGroup)
);
}
private static ObjectNode cleanEvent(ObjectNode event, EventSchema schema, ObjectMapper objectMapper) throws MissingAttributeException, MalformedAttributeException {
ObjectNode result = objectMapper.createObjectNode();
for (EventAttributeDefinition definition : schema.getAttributes()) {
Optional attribute = Utils.getAttributeAccordingToDefinition(event, definition);
Utils.deepSet(result, definition.getPath(), attribute.or(NullNode.getInstance()), objectMapper);
}
return result;
}
private static ObjectNode encodeForFirehose(JsonNode event, EventGroup eventGroup, ObjectMapper objectMapper) throws FirehoseEncodingException {
try {
String base64EncodedEvent = Utils.base64(event.toString(), "UTF-8");
String base64EncodedPayload = Utils.base64(eventGroup + "," + base64EncodedEvent + "\n", "UTF-8");
ObjectNode result = objectMapper.createObjectNode();
result.put("payload", base64EncodedPayload);
return result;
} catch (UnsupportedEncodingException e) {
// cannot really happen as encoding is hard coded to UTF-8 which should always be supported
throw new FirehoseEncodingException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy