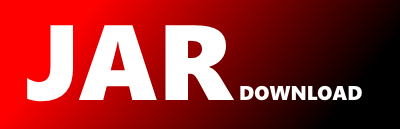
net.leanix.gsit.Utils Maven / Gradle / Ivy
package net.leanix.gsit;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.node.ObjectNode;
import com.fasterxml.jackson.databind.node.TextNode;
import com.google.common.base.Optional;
import com.google.common.hash.Hashing;
import com.google.common.io.BaseEncoding;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.util.Map;
import java.util.function.Supplier;
public class Utils {
public static String md5(String input, String encoding) throws UnsupportedEncodingException {
byte[] inputBytes = input.getBytes(encoding);
byte[] outputBytes = Hashing.md5().hashBytes(inputBytes).asBytes();
return BaseEncoding.base16().encode(outputBytes).toLowerCase();
}
public static String base64(String input, String encoding) throws UnsupportedEncodingException {
byte[] inputBytes = input.getBytes(encoding);
return BaseEncoding.base64().encode(inputBytes);
}
public static JsonNode parseJson(String jsonString, ObjectMapper objectMapper) throws IOException {
return objectMapper.readTree(jsonString);
}
public static V getOrThrow(Map map, K key, Supplier exception) throws T {
V result = map.get(key);
if (result == null) {
throw exception.get();
} else {
return result;
}
}
public static Optional getAttributeAccordingToDefinition(ObjectNode node, EventAttributeDefinition definition) throws MissingAttributeException, MalformedAttributeException {
boolean attributeRequired = definition.isRequired().or(false);
Optional maybeAttribute = deepGet(node, definition.getPath());
boolean attributeNeedsToBeHashed = definition.isHashed().or(false);
Optional result;
if (attributeRequired && !maybeAttribute.isPresent()) {
throw new MissingAttributeException(definition);
}
if (maybeAttribute.isPresent()) {
JsonNode value = maybeAttribute.get();
if (attributeNeedsToBeHashed) {
try {
return Optional.of(TextNode.valueOf(Utils.md5(value.asText(), "UTF-8")));
} catch (UnsupportedEncodingException e) {
throw new MalformedAttributeException(definition, e);
}
} else {
return Optional.of(value);
}
} else {
return Optional.absent();
}
}
public static Optional deepGet(ObjectNode node, String pathString) {
String[] pathSplit = pathString.split("\\.");
JsonNode currentNode = node;
for (int pathIndex = 0; pathIndex < pathSplit.length; pathIndex++) {
String pathSegment = pathSplit[pathIndex];
if (currentNode.has(pathSegment)) {
currentNode = currentNode.get(pathSegment);
if (pathIndex == pathSplit.length - 1) {
if (currentNode.isNull()) {
return Optional.absent();
} else {
return Optional.of(currentNode);
}
} else {
if (!currentNode.isObject()) {
return Optional.absent();
}
}
} else {
return Optional.absent();
}
}
return Optional.absent();
}
public static ObjectNode deepSet(ObjectNode object, String pathString, JsonNode value, ObjectMapper objectMapper) {
String[] pathSplit = pathString.split("\\.");
ObjectNode currentNode = object;
for (int pathIndex = 0; pathIndex < pathSplit.length; pathIndex++) {
String pathSegment = pathSplit[pathIndex];
if (pathIndex == pathSplit.length - 1) {
currentNode.set(pathSegment, value);
} else {
if (currentNode.has(pathSegment) && currentNode.get(pathSegment).isObject()) {
currentNode = (ObjectNode) currentNode.get(pathSegment);
} else {
ObjectNode newNode = objectMapper.createObjectNode();
currentNode.set(pathSegment, newNode);
currentNode = newNode;
}
}
}
return object;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy