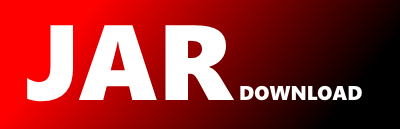
net.leanix.webhooks.api.models.Event Maven / Gradle / Ivy
/*
* LeanIX Webhooks REST API
* Sends notifications on trigger events to subscribed observers
*
* OpenAPI spec version: 2.0.35-SNAPSHOT
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package net.leanix.webhooks.api.models;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import java.util.UUID;
/**
* Event
*/
public class Event {
@JsonProperty("id")
private UUID id = null;
@JsonProperty("createdAt")
private OffsetDateTime createdAt = null;
@JsonProperty("type")
private String type = null;
@JsonProperty("sourceName")
private String sourceName = null;
@JsonProperty("sourceId")
private String sourceId = null;
@JsonProperty("sourceUrl")
private String sourceUrl = null;
@JsonProperty("workspaceId")
private UUID workspaceId = null;
@JsonProperty("userId")
private UUID userId = null;
@JsonProperty("tags")
private List tags = null;
@JsonProperty("payload")
private Object payload = null;
@JsonProperty("sequenceInfo")
private Long sequenceInfo = null;
public Event id(UUID id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@ApiModelProperty(value = "")
public UUID getId() {
return id;
}
public void setId(UUID id) {
this.id = id;
}
public Event createdAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* The date at which the event was originally created.
* @return createdAt
**/
@ApiModelProperty(value = "The date at which the event was originally created.")
public OffsetDateTime getCreatedAt() {
return createdAt;
}
public void setCreatedAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
}
public Event type(String type) {
this.type = type;
return this;
}
/**
* Get type
* @return type
**/
@ApiModelProperty(value = "")
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public Event sourceName(String sourceName) {
this.sourceName = sourceName;
return this;
}
/**
* The name of the source system, e.g. EAM or MTM.
* @return sourceName
**/
@ApiModelProperty(value = "The name of the source system, e.g. EAM or MTM.")
public String getSourceName() {
return sourceName;
}
public void setSourceName(String sourceName) {
this.sourceName = sourceName;
}
public Event sourceId(String sourceId) {
this.sourceId = sourceId;
return this;
}
/**
* The id in the source system.
* @return sourceId
**/
@ApiModelProperty(value = "The id in the source system.")
public String getSourceId() {
return sourceId;
}
public void setSourceId(String sourceId) {
this.sourceId = sourceId;
}
public Event sourceUrl(String sourceUrl) {
this.sourceUrl = sourceUrl;
return this;
}
/**
* The url of the source system, e.g. https://app.leanix.net.
* @return sourceUrl
**/
@ApiModelProperty(value = "The url of the source system, e.g. https://app.leanix.net.")
public String getSourceUrl() {
return sourceUrl;
}
public void setSourceUrl(String sourceUrl) {
this.sourceUrl = sourceUrl;
}
public Event workspaceId(UUID workspaceId) {
this.workspaceId = workspaceId;
return this;
}
/**
* An optional UUID if the event is related to a workspace.
* @return workspaceId
**/
@ApiModelProperty(value = "An optional UUID if the event is related to a workspace.")
public UUID getWorkspaceId() {
return workspaceId;
}
public void setWorkspaceId(UUID workspaceId) {
this.workspaceId = workspaceId;
}
public Event userId(UUID userId) {
this.userId = userId;
return this;
}
/**
* An optional UUID if the event is related to a user.
* @return userId
**/
@ApiModelProperty(value = "An optional UUID if the event is related to a user.")
public UUID getUserId() {
return userId;
}
public void setUserId(UUID userId) {
this.userId = userId;
}
public Event tags(List tags) {
this.tags = tags;
return this;
}
public Event addTagsItem(String tagsItem) {
if (this.tags == null) {
this.tags = new ArrayList();
}
this.tags.add(tagsItem);
return this;
}
/**
* a list of tags describing the nature of this event, used to find subscriptions for this event
* @return tags
**/
@ApiModelProperty(value = "a list of tags describing the nature of this event, used to find subscriptions for this event")
public List getTags() {
return tags;
}
public void setTags(List tags) {
this.tags = tags;
}
public Event payload(Object payload) {
this.payload = payload;
return this;
}
/**
* Contains the event the publisher wants to send wrapped inside this webhooks event here.
* @return payload
**/
@ApiModelProperty(required = true, value = "Contains the event the publisher wants to send wrapped inside this webhooks event here.")
public Object getPayload() {
return payload;
}
public void setPayload(Object payload) {
this.payload = payload;
}
public Event sequenceInfo(Long sequenceInfo) {
this.sequenceInfo = sequenceInfo;
return this;
}
/**
* Get sequenceInfo
* @return sequenceInfo
**/
@ApiModelProperty(value = "")
public Long getSequenceInfo() {
return sequenceInfo;
}
public void setSequenceInfo(Long sequenceInfo) {
this.sequenceInfo = sequenceInfo;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Event event = (Event) o;
return Objects.equals(this.id, event.id) &&
Objects.equals(this.createdAt, event.createdAt) &&
Objects.equals(this.type, event.type) &&
Objects.equals(this.sourceName, event.sourceName) &&
Objects.equals(this.sourceId, event.sourceId) &&
Objects.equals(this.sourceUrl, event.sourceUrl) &&
Objects.equals(this.workspaceId, event.workspaceId) &&
Objects.equals(this.userId, event.userId) &&
Objects.equals(this.tags, event.tags) &&
Objects.equals(this.payload, event.payload) &&
Objects.equals(this.sequenceInfo, event.sequenceInfo);
}
@Override
public int hashCode() {
return Objects.hash(id, createdAt, type, sourceName, sourceId, sourceUrl, workspaceId, userId, tags, payload, sequenceInfo);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Event {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" sourceName: ").append(toIndentedString(sourceName)).append("\n");
sb.append(" sourceId: ").append(toIndentedString(sourceId)).append("\n");
sb.append(" sourceUrl: ").append(toIndentedString(sourceUrl)).append("\n");
sb.append(" workspaceId: ").append(toIndentedString(workspaceId)).append("\n");
sb.append(" userId: ").append(toIndentedString(userId)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" payload: ").append(toIndentedString(payload)).append("\n");
sb.append(" sequenceInfo: ").append(toIndentedString(sequenceInfo)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy