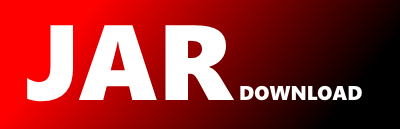
net.leanix.webhooks.api.models.Subscription Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of leanix-webhooks-sdk-java Show documentation
Show all versions of leanix-webhooks-sdk-java Show documentation
SDK for Java to access LeanIX Webhooks REST API
/*
* LeanIX Webhooks REST API
* Sends notifications on trigger events to subscribed observers
*
* OpenAPI spec version: 2.0.35-SNAPSHOT
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package net.leanix.webhooks.api.models;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import java.util.UUID;
/**
* Subscription
*/
public class Subscription {
/**
* Gets or Sets deliveryType
*/
public enum DeliveryTypeEnum {
PUSH("PUSH"),
PULL("PULL");
private String value;
DeliveryTypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static DeliveryTypeEnum fromValue(String text) {
for (DeliveryTypeEnum b : DeliveryTypeEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
}
@JsonProperty("deliveryType")
private DeliveryTypeEnum deliveryType = null;
@JsonProperty("id")
private UUID id = null;
@JsonProperty("identifier")
private String identifier = null;
@JsonProperty("tagSets")
private List> tagSets = null;
@JsonProperty("createdAt")
private OffsetDateTime createdAt = null;
@JsonProperty("workspaceId")
private UUID workspaceId = null;
@JsonProperty("userId")
private UUID userId = null;
@JsonProperty("targetUrl")
private String targetUrl = null;
@JsonProperty("targetMethod")
private String targetMethod = null;
@JsonProperty("authorizationHeader")
private String authorizationHeader = null;
@JsonProperty("callback")
private String callback = null;
@JsonProperty("lastDeliveryStatus")
private Integer lastDeliveryStatus = null;
@JsonProperty("ignoreError")
private Boolean ignoreError = null;
@JsonProperty("maxBatchSize")
private Integer maxBatchSize = null;
/**
* Specifies how the workspaceId is used on events: WORKSPACE -> get events with specified workspaceId (workspaceId must not be null), NO_WORKSPACE -> get events that have no workspaceId (workspaceId must be null), ANY -> get all events regardless if a workspaceId is assigned or not on the event
*/
public enum WorkspaceConstraintEnum {
WORKSPACE("WORKSPACE"),
NO_WORKSPACE("NO_WORKSPACE"),
ANY("ANY");
private String value;
WorkspaceConstraintEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static WorkspaceConstraintEnum fromValue(String text) {
for (WorkspaceConstraintEnum b : WorkspaceConstraintEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
}
@JsonProperty("workspaceConstraint")
private WorkspaceConstraintEnum workspaceConstraint = null;
@JsonProperty("active")
private Boolean active = null;
@JsonProperty("errorCount")
private Integer errorCount = null;
@JsonProperty("firstTimeDeliveryFailed")
private OffsetDateTime firstTimeDeliveryFailed = null;
/**
* Specifies the behavior of the payload property of a delivery for this subscription: DEFAULT -> get only the payload property of the event (default behavior), WRAPPED_EVENT -> get the whole serialized event as the payload, NO_PAYLOAD -> get always an empty {} payload
*/
public enum PayloadModeEnum {
DEFAULT("DEFAULT"),
WRAPPED_EVENT("WRAPPED_EVENT"),
NO_PAYLOAD("NO_PAYLOAD");
private String value;
PayloadModeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static PayloadModeEnum fromValue(String text) {
for (PayloadModeEnum b : PayloadModeEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
}
@JsonProperty("payloadMode")
private PayloadModeEnum payloadMode = null;
public Subscription deliveryType(DeliveryTypeEnum deliveryType) {
this.deliveryType = deliveryType;
return this;
}
/**
* Get deliveryType
* @return deliveryType
**/
@ApiModelProperty(value = "")
public DeliveryTypeEnum getDeliveryType() {
return deliveryType;
}
public void setDeliveryType(DeliveryTypeEnum deliveryType) {
this.deliveryType = deliveryType;
}
public Subscription id(UUID id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@ApiModelProperty(value = "")
public UUID getId() {
return id;
}
public void setId(UUID id) {
this.id = id;
}
public Subscription identifier(String identifier) {
this.identifier = identifier;
return this;
}
/**
* Get identifier
* @return identifier
**/
@ApiModelProperty(value = "")
public String getIdentifier() {
return identifier;
}
public void setIdentifier(String identifier) {
this.identifier = identifier;
}
public Subscription tagSets(List> tagSets) {
this.tagSets = tagSets;
return this;
}
public Subscription addTagSetsItem(List tagSetsItem) {
if (this.tagSets == null) {
this.tagSets = new ArrayList>();
}
this.tagSets.add(tagSetsItem);
return this;
}
/**
* Get tagSets
* @return tagSets
**/
@ApiModelProperty(value = "")
public List> getTagSets() {
return tagSets;
}
public void setTagSets(List> tagSets) {
this.tagSets = tagSets;
}
public Subscription createdAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* Get createdAt
* @return createdAt
**/
@ApiModelProperty(value = "")
public OffsetDateTime getCreatedAt() {
return createdAt;
}
public void setCreatedAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
}
public Subscription workspaceId(UUID workspaceId) {
this.workspaceId = workspaceId;
return this;
}
/**
* Get workspaceId
* @return workspaceId
**/
@ApiModelProperty(value = "")
public UUID getWorkspaceId() {
return workspaceId;
}
public void setWorkspaceId(UUID workspaceId) {
this.workspaceId = workspaceId;
}
public Subscription userId(UUID userId) {
this.userId = userId;
return this;
}
/**
* Get userId
* @return userId
**/
@ApiModelProperty(value = "")
public UUID getUserId() {
return userId;
}
public void setUserId(UUID userId) {
this.userId = userId;
}
public Subscription targetUrl(String targetUrl) {
this.targetUrl = targetUrl;
return this;
}
/**
* Used and required when 'deliveryType' is 'PUSH'.
* @return targetUrl
**/
@ApiModelProperty(required = true, value = "Used and required when 'deliveryType' is 'PUSH'.")
public String getTargetUrl() {
return targetUrl;
}
public void setTargetUrl(String targetUrl) {
this.targetUrl = targetUrl;
}
public Subscription targetMethod(String targetMethod) {
this.targetMethod = targetMethod;
return this;
}
/**
* Used when 'deliveryType' is 'PUSH'.
* @return targetMethod
**/
@ApiModelProperty(required = true, value = "Used when 'deliveryType' is 'PUSH'.")
public String getTargetMethod() {
return targetMethod;
}
public void setTargetMethod(String targetMethod) {
this.targetMethod = targetMethod;
}
public Subscription authorizationHeader(String authorizationHeader) {
this.authorizationHeader = authorizationHeader;
return this;
}
/**
* Used when 'deliveryType' is 'PUSH'.
* @return authorizationHeader
**/
@ApiModelProperty(value = "Used when 'deliveryType' is 'PUSH'.")
public String getAuthorizationHeader() {
return authorizationHeader;
}
public void setAuthorizationHeader(String authorizationHeader) {
this.authorizationHeader = authorizationHeader;
}
public Subscription callback(String callback) {
this.callback = callback;
return this;
}
/**
* Used when 'deliveryType' is 'PUSH'.
* @return callback
**/
@ApiModelProperty(value = "Used when 'deliveryType' is 'PUSH'.")
public String getCallback() {
return callback;
}
public void setCallback(String callback) {
this.callback = callback;
}
public Subscription lastDeliveryStatus(Integer lastDeliveryStatus) {
this.lastDeliveryStatus = lastDeliveryStatus;
return this;
}
/**
* Used when 'deliveryType' is 'PUSH'.
* @return lastDeliveryStatus
**/
@ApiModelProperty(value = "Used when 'deliveryType' is 'PUSH'.")
public Integer getLastDeliveryStatus() {
return lastDeliveryStatus;
}
public void setLastDeliveryStatus(Integer lastDeliveryStatus) {
this.lastDeliveryStatus = lastDeliveryStatus;
}
public Subscription ignoreError(Boolean ignoreError) {
this.ignoreError = ignoreError;
return this;
}
/**
* Don't re-send events that caused an error on PUSH. Shirks the at-least-once policy! No effect on PULL.
* @return ignoreError
**/
@ApiModelProperty(required = true, value = "Don't re-send events that caused an error on PUSH. Shirks the at-least-once policy! No effect on PULL.")
public Boolean getIgnoreError() {
return ignoreError;
}
public void setIgnoreError(Boolean ignoreError) {
this.ignoreError = ignoreError;
}
public Subscription maxBatchSize(Integer maxBatchSize) {
this.maxBatchSize = maxBatchSize;
return this;
}
/**
* Used when 'deliveryType' is 'PULL'. The desired maximum size (in kiB) of the returned batch of events. Default is 512. This is an approximate number: Actual responses may be smaller, or bigger when there is one single event exceeding maxBatchSize.
* minimum: 1
* maximum: 51200
* @return maxBatchSize
**/
@ApiModelProperty(value = "Used when 'deliveryType' is 'PULL'. The desired maximum size (in kiB) of the returned batch of events. Default is 512. This is an approximate number: Actual responses may be smaller, or bigger when there is one single event exceeding maxBatchSize.")
public Integer getMaxBatchSize() {
return maxBatchSize;
}
public void setMaxBatchSize(Integer maxBatchSize) {
this.maxBatchSize = maxBatchSize;
}
public Subscription workspaceConstraint(WorkspaceConstraintEnum workspaceConstraint) {
this.workspaceConstraint = workspaceConstraint;
return this;
}
/**
* Specifies how the workspaceId is used on events: WORKSPACE -> get events with specified workspaceId (workspaceId must not be null), NO_WORKSPACE -> get events that have no workspaceId (workspaceId must be null), ANY -> get all events regardless if a workspaceId is assigned or not on the event
* @return workspaceConstraint
**/
@ApiModelProperty(value = "Specifies how the workspaceId is used on events: WORKSPACE -> get events with specified workspaceId (workspaceId must not be null), NO_WORKSPACE -> get events that have no workspaceId (workspaceId must be null), ANY -> get all events regardless if a workspaceId is assigned or not on the event")
public WorkspaceConstraintEnum getWorkspaceConstraint() {
return workspaceConstraint;
}
public void setWorkspaceConstraint(WorkspaceConstraintEnum workspaceConstraint) {
this.workspaceConstraint = workspaceConstraint;
}
public Subscription active(Boolean active) {
this.active = active;
return this;
}
/**
* Get active
* @return active
**/
@ApiModelProperty(value = "")
public Boolean getActive() {
return active;
}
public void setActive(Boolean active) {
this.active = active;
}
public Subscription errorCount(Integer errorCount) {
this.errorCount = errorCount;
return this;
}
/**
* Get errorCount
* @return errorCount
**/
@ApiModelProperty(value = "")
public Integer getErrorCount() {
return errorCount;
}
public void setErrorCount(Integer errorCount) {
this.errorCount = errorCount;
}
public Subscription firstTimeDeliveryFailed(OffsetDateTime firstTimeDeliveryFailed) {
this.firstTimeDeliveryFailed = firstTimeDeliveryFailed;
return this;
}
/**
* Get firstTimeDeliveryFailed
* @return firstTimeDeliveryFailed
**/
@ApiModelProperty(value = "")
public OffsetDateTime getFirstTimeDeliveryFailed() {
return firstTimeDeliveryFailed;
}
public void setFirstTimeDeliveryFailed(OffsetDateTime firstTimeDeliveryFailed) {
this.firstTimeDeliveryFailed = firstTimeDeliveryFailed;
}
public Subscription payloadMode(PayloadModeEnum payloadMode) {
this.payloadMode = payloadMode;
return this;
}
/**
* Specifies the behavior of the payload property of a delivery for this subscription: DEFAULT -> get only the payload property of the event (default behavior), WRAPPED_EVENT -> get the whole serialized event as the payload, NO_PAYLOAD -> get always an empty {} payload
* @return payloadMode
**/
@ApiModelProperty(value = "Specifies the behavior of the payload property of a delivery for this subscription: DEFAULT -> get only the payload property of the event (default behavior), WRAPPED_EVENT -> get the whole serialized event as the payload, NO_PAYLOAD -> get always an empty {} payload")
public PayloadModeEnum getPayloadMode() {
return payloadMode;
}
public void setPayloadMode(PayloadModeEnum payloadMode) {
this.payloadMode = payloadMode;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Subscription subscription = (Subscription) o;
return Objects.equals(this.deliveryType, subscription.deliveryType) &&
Objects.equals(this.id, subscription.id) &&
Objects.equals(this.identifier, subscription.identifier) &&
Objects.equals(this.tagSets, subscription.tagSets) &&
Objects.equals(this.createdAt, subscription.createdAt) &&
Objects.equals(this.workspaceId, subscription.workspaceId) &&
Objects.equals(this.userId, subscription.userId) &&
Objects.equals(this.targetUrl, subscription.targetUrl) &&
Objects.equals(this.targetMethod, subscription.targetMethod) &&
Objects.equals(this.authorizationHeader, subscription.authorizationHeader) &&
Objects.equals(this.callback, subscription.callback) &&
Objects.equals(this.lastDeliveryStatus, subscription.lastDeliveryStatus) &&
Objects.equals(this.ignoreError, subscription.ignoreError) &&
Objects.equals(this.maxBatchSize, subscription.maxBatchSize) &&
Objects.equals(this.workspaceConstraint, subscription.workspaceConstraint) &&
Objects.equals(this.active, subscription.active) &&
Objects.equals(this.errorCount, subscription.errorCount) &&
Objects.equals(this.firstTimeDeliveryFailed, subscription.firstTimeDeliveryFailed) &&
Objects.equals(this.payloadMode, subscription.payloadMode);
}
@Override
public int hashCode() {
return Objects.hash(deliveryType, id, identifier, tagSets, createdAt, workspaceId, userId, targetUrl, targetMethod, authorizationHeader, callback, lastDeliveryStatus, ignoreError, maxBatchSize, workspaceConstraint, active, errorCount, firstTimeDeliveryFailed, payloadMode);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Subscription {\n");
sb.append(" deliveryType: ").append(toIndentedString(deliveryType)).append("\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" identifier: ").append(toIndentedString(identifier)).append("\n");
sb.append(" tagSets: ").append(toIndentedString(tagSets)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" workspaceId: ").append(toIndentedString(workspaceId)).append("\n");
sb.append(" userId: ").append(toIndentedString(userId)).append("\n");
sb.append(" targetUrl: ").append(toIndentedString(targetUrl)).append("\n");
sb.append(" targetMethod: ").append(toIndentedString(targetMethod)).append("\n");
sb.append(" authorizationHeader: ").append(toIndentedString(authorizationHeader)).append("\n");
sb.append(" callback: ").append(toIndentedString(callback)).append("\n");
sb.append(" lastDeliveryStatus: ").append(toIndentedString(lastDeliveryStatus)).append("\n");
sb.append(" ignoreError: ").append(toIndentedString(ignoreError)).append("\n");
sb.append(" maxBatchSize: ").append(toIndentedString(maxBatchSize)).append("\n");
sb.append(" workspaceConstraint: ").append(toIndentedString(workspaceConstraint)).append("\n");
sb.append(" active: ").append(toIndentedString(active)).append("\n");
sb.append(" errorCount: ").append(toIndentedString(errorCount)).append("\n");
sb.append(" firstTimeDeliveryFailed: ").append(toIndentedString(firstTimeDeliveryFailed)).append("\n");
sb.append(" payloadMode: ").append(toIndentedString(payloadMode)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy