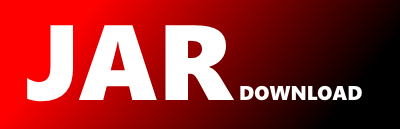
net.lenni0451.commandlib.builder.ArgumentBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of CommandLib Show documentation
Show all versions of CommandLib Show documentation
A command lib with a simple and powerful API
The newest version!
package net.lenni0451.commandlib.builder;
import net.lenni0451.commandlib.nodes.*;
import net.lenni0451.commandlib.types.ArgumentType;
import net.lenni0451.commandlib.types.DynamicType;
import net.lenni0451.commandlib.types.DynamicType.BiParser;
import net.lenni0451.commandlib.types.DynamicType.SingleParser;
import net.lenni0451.commandlib.utils.Util;
import net.lenni0451.commandlib.utils.comparator.ArgumentComparator;
import javax.annotation.Nullable;
/**
* A convenience interface to allow for the easy creation of arguments.
*
* @param The type of the executor
*/
public interface ArgumentBuilder {
/**
* Create a new string argument node.
* The argument will be matched as a single word. The case-sensitivity will be determined by the {@link ArgumentComparator} in the command executor.
*
* @param s The name of the argument
* @return The created node
*/
default StringNode string(final String s) {
return new StringNode<>(s);
}
/**
* Create a new string argument node.
* The argument will be matched as a single word. The case-sensitivity will be determined by the {@link ArgumentComparator} in the command executor.
*
* @param s The name of the argument
* @param description The description of the argument
* @return The created node
*/
default StringNode string(final String s, @Nullable final String description) {
return new StringNode<>(s, description);
}
/**
* Create a new typed argument node.
* The argument will be parsed by the given {@link ArgumentType}.
*
* @param name The name of the argument
* @param type The argument type
* @param The type of the argument
* @return The created node
*/
default TypedNode typed(final String name, final ArgumentType type) {
return new TypedNode<>(name, type);
}
/**
* Create a new typed argument node.
* The argument will be parsed by the given {@link ArgumentType}.
*
* @param name The name of the argument
* @param description The description of the argument
* @param type The argument type
* @param The type of the argument
* @return The created node
*/
default TypedNode typed(final String name, @Nullable final String description, final ArgumentType type) {
return new TypedNode<>(name, description, type);
}
/**
* Create a redirect node.
* The redirect node will redirect the execution to the given target node.
*
* @param targetNode The target node
* @return The created node
*/
default RedirectNode redirect(final ArgumentNode targetNode) {
return new RedirectNode<>(targetNode);
}
/**
* Create a new list argument node.
* The argument will be parsed by the given {@link ArgumentType}.
* The argument can be repeated multiple times.
*
* @param name The name of the argument
* @param type The argument type
* @param The type of the argument
* @return The created node
*/
default ListNode list(final String name, final ArgumentType type) {
return new ListNode<>(name, type);
}
/**
* Create a new list argument node.
* The argument will be parsed by the given {@link ArgumentType}.
* The argument can be repeated multiple times.
*
* @param name The name of the argument
* @param description The description of the argument
* @param type The argument type
* @param The type of the argument
* @return The created node
*/
default ListNode list(final String name, @Nullable final String description, final ArgumentType type) {
return new ListNode<>(name, description, type);
}
/**
* Create a new string array argument node.
* The given executor receives the whole string array as argument.
* This allows you to handle commands the traditional way.
*
* @param name The name of the argument
* @param executor The executor
* @return The created node
*/
default StringArrayNode stringArray(final String name, final StringArrayNode.Executor executor) {
StringArrayNode node = new StringArrayNode<>(name, null);
node.executes(executionContext -> {
executor.execute(Util.cast(executionContext.getExecutor()), executionContext.getArgument(name), Util.cast(executionContext));
});
return node;
}
/**
* Create a new string array argument node.
* The given executor receives the whole string array as argument.
* The given completor receives all arguments to this point as argument.
* This allows you to handle commands the traditional way.
*
* @param name The name of the argument
* @param executor The executor
* @param completor The completor
* @return The created node
*/
default StringArrayNode stringArray(final String name, final StringArrayNode.Executor executor, final StringArrayNode.Completor completor) {
StringArrayNode node = new StringArrayNode<>(name, completor);
node.executes(executionContext -> {
executor.execute(Util.cast(executionContext.getExecutor()), executionContext.getArgument(name), Util.cast(executionContext));
});
return node;
}
/**
* Create a new string array argument node.
* The given executor receives the whole string array as argument.
* This allows you to handle commands the traditional way.
*
* @param name The name of the argument
* @param description The description of the argument
* @param executor The executor
* @return The created node
*/
default StringArrayNode stringArray(final String name, @Nullable final String description, final StringArrayNode.Executor executor) {
StringArrayNode node = new StringArrayNode<>(name, description, null);
node.executes(executionContext -> {
executor.execute(Util.cast(executionContext.getExecutor()), executionContext.getArgument(name), Util.cast(executionContext));
});
return node;
}
/**
* Create a new string array argument node.
* The given executor receives the whole string array as argument.
* The given completor receives all arguments to this point as argument.
* This allows you to handle commands the traditional way.
*
* @param name The name of the argument
* @param description The description of the argument
* @param executor The executor
* @param completor The completor
* @return The created node
*/
default StringArrayNode stringArray(final String name, @Nullable final String description, final StringArrayNode.Executor executor, final StringArrayNode.Completor completor) {
StringArrayNode node = new StringArrayNode<>(name, description, completor);
node.executes(executionContext -> {
executor.execute(Util.cast(executionContext.getExecutor()), executionContext.getArgument(name), Util.cast(executionContext));
});
return node;
}
/**
* Create a dynamic parsing argument type.
* The {@link SingleParser} only receives the string reader to parse the argument.
*
* @param parser The parser
* @param The type of the parsed argument
* @return The created argument type
*/
default ArgumentType dynamicType(final SingleParser parser) {
return new DynamicType<>(parser);
}
/**
* Create a dynamic parsing argument type.
* The {@link BiParser} receives the execution context and the string reader to parse the argument.
*
* @param parser The parser
* @param The type of the parsed argument
* @return The created argument type
*/
default ArgumentType dynamicType(final BiParser parser) {
return new DynamicType<>(parser);
}
/**
* @return A new line builder
*/
default LineBuilder line() {
return LineBuilder.create();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy