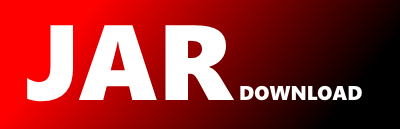
net.lenni0451.optconfig.index.ConfigDiff Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of optconfig Show documentation
Show all versions of optconfig Show documentation
A java library for parsing yaml config files with support for comments and default values
The newest version!
package net.lenni0451.optconfig.index;
import net.lenni0451.optconfig.annotations.OptConfig;
import net.lenni0451.optconfig.index.types.ConfigOption;
import net.lenni0451.optconfig.index.types.SectionIndex;
import org.jetbrains.annotations.ApiStatus;
import java.util.*;
@ApiStatus.Internal
public class ConfigDiff {
public static ConfigDiff diff(final SectionIndex sectionIndex, final Map values) {
ConfigDiff configDiff = new ConfigDiff();
Set missedKeys = new HashSet<>(values.keySet());
for (ConfigOption option : sectionIndex.getOptions()) {
if (!missedKeys.remove(option.getName())) {
//If a key is not present in the values, it was added to the config
configDiff.addedKeys.add(option.getName());
continue;
}
if (sectionIndex.getSubSections().containsKey(option)) {
Object value = values.get(option.getName());
if (!(value instanceof Map)) continue; //If a subsection is not a map, let the deserializer deal with it
Map subSectionValues = (Map) value;
ConfigDiff subSectionDiff = ConfigDiff.diff(sectionIndex.getSubSections().get(option), subSectionValues);
configDiff.subSections.put(option.getName(), subSectionDiff);
}
}
missedKeys.remove(OptConfig.CONFIG_VERSION_OPTION); //Ignore the version key
configDiff.removedKeys.addAll(missedKeys); //All keys that are left were removed from the config
return configDiff;
}
private final List addedKeys;
private final List removedKeys;
private final List invalidKeys; //Used by the deserializer if an option has an invalid value
private final Map subSections;
private ConfigDiff() {
this.addedKeys = new ArrayList<>();
this.removedKeys = new ArrayList<>();
this.invalidKeys = new ArrayList<>();
this.subSections = new HashMap<>();
}
public List getAddedKeys() {
return this.addedKeys;
}
public List getRemovedKeys() {
return this.removedKeys;
}
public List getInvalidKeys() {
return this.invalidKeys;
}
public Map getSubSections() {
return this.subSections;
}
public boolean isEmpty() {
return this.addedKeys.isEmpty() && this.removedKeys.isEmpty() && this.invalidKeys.isEmpty() && this.subSections.values().stream().allMatch(ConfigDiff::isEmpty);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy