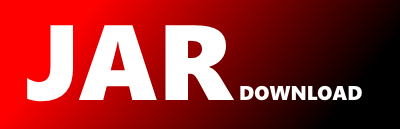
net.lightbody.bmp.proxy.jetty.jetty.servlet.Dispatcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of browsermob-proxy Show documentation
Show all versions of browsermob-proxy Show documentation
A programmatic HTTP/S designed for performance and functional testing
// ========================================================================
// $Id: Dispatcher.java,v 1.92 2005/12/12 18:03:31 gregwilkins Exp $
// Copyright 199-2004 Mort Bay Consulting Pty. Ltd.
// ------------------------------------------------------------------------
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
// http://www.apache.org/licenses/LICENSE-2.0
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
// ========================================================================
package net.lightbody.bmp.proxy.jetty.jetty.servlet;
import net.lightbody.bmp.proxy.jetty.http.HttpConnection;
import net.lightbody.bmp.proxy.jetty.http.PathMap;
import net.lightbody.bmp.proxy.jetty.log.LogFactory;
import net.lightbody.bmp.proxy.jetty.util.*;
import org.apache.commons.logging.Log;
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.*;
/* ------------------------------------------------------------ */
/** Servlet RequestDispatcher.
*
* @version $Id: Dispatcher.java,v 1.92 2005/12/12 18:03:31 gregwilkins Exp $
* @author Greg Wilkins (gregw)
*/
public class Dispatcher implements RequestDispatcher
{
static Log log = LogFactory.getLog(Dispatcher.class);
/* ------------------------------------------------------------ */
/** Dispatch types */
public static final int __DEFAULT=0;
public static final int __REQUEST=1;
public static final int __FORWARD=2;
public static final int __INCLUDE=4;
public static final int __ERROR=8;
public static final int __ALL=15;
/** Dispatch include attribute names */
public final static String __INCLUDE_REQUEST_URI= "javax.servlet.include.request_uri";
public final static String __INCLUDE_CONTEXT_PATH= "javax.servlet.include.context_path";
public final static String __INCLUDE_SERVLET_PATH= "javax.servlet.include.servlet_path";
public final static String __INCLUDE_PATH_INFO= "javax.servlet.include.path_info";
public final static String __INCLUDE_QUERY_STRING= "javax.servlet.include.query_string";
/** Dispatch include attribute names */
public final static String __FORWARD_REQUEST_URI= "javax.servlet.forward.request_uri";
public final static String __FORWARD_CONTEXT_PATH= "javax.servlet.forward.context_path";
public final static String __FORWARD_SERVLET_PATH= "javax.servlet.forward.servlet_path";
public final static String __FORWARD_PATH_INFO= "javax.servlet.forward.path_info";
public final static String __FORWARD_QUERY_STRING= "javax.servlet.forward.query_string";
public final static StringMap __managedAttributes = new StringMap();
static
{
__managedAttributes.put(__INCLUDE_REQUEST_URI,__INCLUDE_REQUEST_URI);
__managedAttributes.put(__INCLUDE_CONTEXT_PATH,__INCLUDE_CONTEXT_PATH);
__managedAttributes.put(__INCLUDE_SERVLET_PATH,__INCLUDE_SERVLET_PATH);
__managedAttributes.put(__INCLUDE_PATH_INFO,__INCLUDE_PATH_INFO);
__managedAttributes.put(__INCLUDE_QUERY_STRING,__INCLUDE_QUERY_STRING);
__managedAttributes.put(__FORWARD_REQUEST_URI,__FORWARD_REQUEST_URI);
__managedAttributes.put(__FORWARD_CONTEXT_PATH,__FORWARD_CONTEXT_PATH);
__managedAttributes.put(__FORWARD_SERVLET_PATH,__FORWARD_SERVLET_PATH);
__managedAttributes.put(__FORWARD_PATH_INFO,__FORWARD_PATH_INFO);
__managedAttributes.put(__FORWARD_QUERY_STRING,__FORWARD_QUERY_STRING);
}
ServletHandler _servletHandler;
ServletHolder _holder=null;
String _pathSpec;
String _uriInContext;
String _pathInContext;
String _query;
/* ------------------------------------------------------------ */
/** Constructor.
/** Constructor.
* @param servletHandler
* @param uriInContext Encoded uriInContext
* @param pathInContext Encoded pathInContext
* @param query
* @exception IllegalStateException
*/
Dispatcher(ServletHandler servletHandler,
String uriInContext,
String pathInContext,
String query,
Map.Entry entry)
throws IllegalStateException
{
if(log.isDebugEnabled())log.debug("Dispatcher for "+servletHandler+","+uriInContext+","+query);
_servletHandler=servletHandler;
_uriInContext=uriInContext;
_pathInContext=pathInContext;
_query=query;
_pathSpec=(String)entry.getKey();
_holder = (ServletHolder)entry.getValue();
}
/* ------------------------------------------------------------ */
/** Constructor.
* @param servletHandler
* @param name
*/
Dispatcher(ServletHandler servletHandler,String name)
throws IllegalStateException
{
_servletHandler=servletHandler;
_holder=_servletHandler.getServletHolder(name);
if (_holder==null)
throw new IllegalStateException("No named servlet handler in context");
}
/* ------------------------------------------------------------ */
public boolean isNamed()
{
return _pathInContext==null;
}
/* ------------------------------------------------------------ */
public void include(ServletRequest servletRequest,
ServletResponse servletResponse)
throws ServletException, IOException
{
dispatch(servletRequest,servletResponse,Dispatcher.__INCLUDE);
}
/* ------------------------------------------------------------ */
public void forward(ServletRequest servletRequest,
ServletResponse servletResponse)
throws ServletException,IOException
{
dispatch(servletRequest,servletResponse,Dispatcher.__FORWARD);
}
/* ------------------------------------------------------------ */
void error(ServletRequest servletRequest,
ServletResponse servletResponse)
throws ServletException,IOException
{
dispatch(servletRequest,servletResponse,Dispatcher.__ERROR);
}
/* ------------------------------------------------------------ */
void dispatch(ServletRequest servletRequest,
ServletResponse servletResponse,
int type)
throws ServletException,IOException
{
HttpServletRequest httpServletRequest=(HttpServletRequest)servletRequest;
HttpServletResponse httpServletResponse=(HttpServletResponse)servletResponse;
HttpConnection httpConnection=
_servletHandler.getHttpContext().getHttpConnection();
ServletHttpRequest servletHttpRequest=
(ServletHttpRequest)httpConnection.getRequest().getWrapper();
// wrap the request and response
DispatcherRequest request = new DispatcherRequest(httpServletRequest,
servletHttpRequest,
type);
DispatcherResponse response = new DispatcherResponse(request,
httpServletResponse);
if (type==Dispatcher.__FORWARD)
servletResponse.resetBuffer();
// Merge parameters
String query=_query;
MultiMap parameters=null;
if (query!=null)
{
Map old_params = httpServletRequest.getParameterMap();
// Add the parameters
parameters=new MultiMap();
UrlEncoded.decodeTo(query,parameters,request.getCharacterEncoding());
if (old_params!=null && old_params.size()>0)
{
// Merge old parameters.
Iterator iter = old_params.entrySet().iterator();
while (iter.hasNext())
{
Map.Entry entry = (Map.Entry)iter.next();
String name=(String)entry.getKey();
String[] values=(String[])entry.getValue();
for (int i=0;i0)
buf.append(_contextPath);
buf.append(_uriInContext);
return buf;
}
/* ------------------------------------------------------------ */
public String getPathTranslated()
{
String info=getPathInfo();
if (info==null)
return null;
return getRealPath(info);
}
/* ------------------------------------------------------------ */
StringBuffer getRootURL()
{
StringBuffer buf = super.getRequestURL();
int d=3;
for (int i=0;i1)
relTo=relTo.substring(0,slash+1);
else
relTo="/";
url=URI.addPaths(relTo,url);
}
return _servletHandler.getServletContext().getRequestDispatcher(url);
}
public String getMethod()
{
if (this._filterType==Dispatcher.__ERROR)
return net.lightbody.bmp.proxy.jetty.http.HttpRequest.__GET;
return super.getMethod();
}
}
/* ------------------------------------------------------------ */
/* ------------------------------------------------------------ */
/* ------------------------------------------------------------ */
class DispatcherResponse extends HttpServletResponseWrapper
{
DispatcherRequest _request;
private ServletOutputStream _out=null;
private PrintWriter _writer=null;
private boolean _flushNeeded=false;
private boolean _include;
/* ------------------------------------------------------------ */
DispatcherResponse(DispatcherRequest request, HttpServletResponse response)
{
super(response);
_request=request;
request._response=this;
_include=_request._filterType==Dispatcher.__INCLUDE;
}
/* ------------------------------------------------------------ */
public ServletOutputStream getOutputStream()
throws IOException
{
if (_writer!=null)
throw new IllegalStateException("getWriter called");
if (_out==null)
{
try {_out=super.getOutputStream();}
catch(IllegalStateException e)
{
LogSupport.ignore(log,e);
_flushNeeded=true;
_out=new ServletOut(new WriterOutputStream(super.getWriter()));
}
}
if (_include)
_out=new DontCloseServletOut(_out);
return _out;
}
/* ------------------------------------------------------------ */
public PrintWriter getWriter()
throws IOException
{
if (_out!=null)
throw new IllegalStateException("getOutputStream called");
if (_writer==null)
{
try{_writer=super.getWriter();}
catch(IllegalStateException e)
{
LogSupport.ignore(log, e);
_flushNeeded=true;
_writer = new ServletWriter(super.getOutputStream(),
getCharacterEncoding());
}
}
if (_include)
_writer=new DontCloseWriter(_writer);
return _writer;
}
/* ------------------------------------------------------------ */
boolean isFlushNeeded()
{
return _flushNeeded;
}
/* ------------------------------------------------------------ */
public void flushBuffer()
throws IOException
{
if (_writer!=null)
_writer.flush();
if (_out!=null)
_out.flush();
super.flushBuffer();
}
/* ------------------------------------------------------------ */
public void close()
throws IOException
{
if (_writer!=null)
_writer.close();
if (_out!=null)
_out.close();
}
/* ------------------------------------------------------------ */
public void setLocale(Locale locale)
{
if (!_include) super.setLocale(locale);
}
/* ------------------------------------------------------------ */
public void sendError(int status, String message)
throws IOException
{
if (_request._filterType!=Dispatcher.__ERROR && !_include)
super.sendError(status,message);
}
/* ------------------------------------------------------------ */
public void sendError(int status)
throws IOException
{
if (_request._filterType!=Dispatcher.__ERROR && !_include)
super.sendError(status);
}
/* ------------------------------------------------------------ */
public void sendRedirect(String url)
throws IOException
{
if (!_include)
{
if (!url.startsWith("http:/")&&!url.startsWith("https:/"))
{
StringBuffer buf = _request.getRootURL();
if (url.startsWith("/"))
buf.append(URI.canonicalPath(url));
else
buf.append(URI.canonicalPath(URI.addPaths(URI.parentPath(_request.getRequestURI()),url)));
url=buf.toString();
}
super.sendRedirect(url);
}
}
/* ------------------------------------------------------------ */
public void setDateHeader(String name, long value)
{
if (!_include) super.setDateHeader(name,value);
}
/* ------------------------------------------------------------ */
public void setHeader(String name, String value)
{
if (!_include) super.setHeader(name,value);
}
/* ------------------------------------------------------------ */
public void setIntHeader(String name, int value)
{
if (!_include) super.setIntHeader(name,value);
}
/* ------------------------------------------------------------ */
public void addHeader(String name, String value)
{
if (!_include) super.addHeader(name,value);
}
/* ------------------------------------------------------------ */
public void addDateHeader(String name, long value)
{
if (!_include) super.addDateHeader(name,value);
}
/* ------------------------------------------------------------ */
public void addIntHeader(String name, int value)
{
if (!_include) super.addIntHeader(name,value);
}
/* ------------------------------------------------------------ */
public void setStatus(int status)
{
if (_request._filterType!=Dispatcher.__ERROR && !_include)
super.setStatus(status);
}
/* ------------------------------------------------------------ */
/**
* The default behavior of this method is to call setStatus(int sc, String sm)
* on the wrapped response object.
*
* @deprecated As of version 2.1 of the Servlet spec.
* To set a status code
* use setStatus(int)
, to send an error with a description
* use sendError(int, String)
.
*
* @param status the status code
* @param message the status message
*/
public void setStatus(int status, String message)
{
if (_request._filterType!=Dispatcher.__ERROR && !_include)
super.setStatus(status,message);
}
/* ------------------------------------------------------------ */
public void setContentLength(int len)
{
if (!_include) super.setContentLength(len);
}
/* ------------------------------------------------------------ */
public void setContentType(String contentType)
{
if (!_include) super.setContentType(contentType);
}
/* ------------------------------------------------------------ */
public void addCookie(Cookie cookie)
{
if (!_include) super.addCookie(cookie);
}
}
/* ------------------------------------------------------------ */
/* ------------------------------------------------------------ */
/* ------------------------------------------------------------ */
private class DontCloseWriter extends PrintWriter
{
DontCloseWriter(PrintWriter writer)
{
super(writer);
}
public void close()
{}
}
/* ------------------------------------------------------------ */
/* ------------------------------------------------------------ */
/* ------------------------------------------------------------ */
private class DontCloseServletOut extends ServletOut
{
DontCloseServletOut(ServletOutputStream output)
{
super(output);
}
public void close()
throws IOException
{}
}
};
© 2015 - 2025 Weber Informatics LLC | Privacy Policy