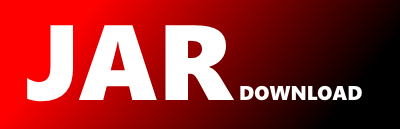
net.linksfield.cube.partnersdk.configuration.EndpointPropertiesProxy Maven / Gradle / Ivy
package net.linksfield.cube.partnersdk.configuration;
import lombok.Data;
import net.linksfield.cube.partnersdk.configuration.url.*;
import java.beans.IntrospectionException;
import java.beans.PropertyDescriptor;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
import java.util.Map;
import java.util.Properties;
/**
* @ClassName EndpointPropertiesProxy
* @Description 配置文件 代理
* 自动映射properties中的属性到对应字段中
* @Author James.hu
* @Date 2023/3/15
**/
@Data
public class EndpointPropertiesProxy extends Properties {
/** 主机地址 */
private String host;
private Service service;
private Usage usage;
private Sim sim;
private Sms sms;
private Cdr cdr;
private Other other;
private Mall mall;
private Orders orders;
/**
* 自动匹配配置文件的属性到实例的字段上
*/
public void bindingField() {
EndpointPropertiesProxy instance = this;
instance.host = this.getProperty("host");
for (Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy