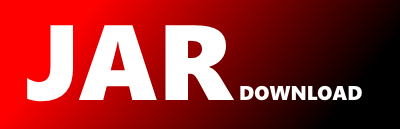
net.linksfield.cube.partnersdk.domain.BaseRequest Maven / Gradle / Ivy
package net.linksfield.cube.partnersdk.domain;
import com.google.common.collect.HashMultimap;
import com.google.common.collect.Multimap;
import lombok.Data;
import net.linksfield.cube.partnersdk.configuration.EndpointPropertiesProxy;
import net.linksfield.cube.partnersdk.rest.HttpHeaders;
import net.linksfield.cube.partnersdk.rest.HttpMethod;
import net.linksfield.cube.partnersdk.rest.MediaType;
import java.util.Map;
/**
* @ClassName BaseRequest
* @Description 基础请求参数
* @Author James.hu
* @Date 2023/3/14
**/
@Data
public abstract class BaseRequest {
/** Http Method */
protected HttpMethod httpMethod;
/** Timestamp */
protected String timestamp;
/** Nonce */
protected int nonce;
/** Client token */
private String clientToken;
protected Multimap extendHeaders;
public BaseRequest(HttpMethod httpMethod, int nonce) {
this.httpMethod = httpMethod;
this.timestamp = Long.toString(System.currentTimeMillis());
this.nonce = nonce;
this.extendHeaders = HashMultimap.create();
setContentType();
}
/**
* 由子类返回需要的Url
* @param endpointPropertiesProxy
* @return
*/
public abstract String requestUrl(EndpointPropertiesProxy endpointPropertiesProxy);
public abstract void addUrlSignatureParameters(Map mapToSign);
/** 由子类实现 添加查询参数 */
public abstract void addQueryParams(Multimap queryParams);
/** 由子类实现 添加post内容体 */
public abstract void addBody(Map body);
protected void setContentType() {
this.extendHeaders.put(HttpHeaders.ContentType, MediaType.APPLICATION_JSON);
}
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
this.extendHeaders.put("Client-token", clientToken);
}
protected void addExtendHeader(String key, String value) {
this.extendHeaders.put(key, value);
}
protected void addOptionalQueryParam(Multimap queryParams, String name, Object item) {
if (item != null) {
queryParams.put(name, item.toString());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy