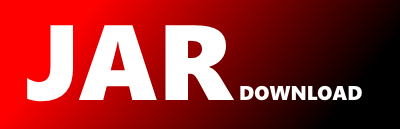
net.linksfield.cube.partnersdk.json.JacksonJsonSerializer Maven / Gradle / Ivy
package net.linksfield.cube.partnersdk.json;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
import lombok.extern.slf4j.Slf4j;
import java.io.IOException;
import java.util.Map;
/**
* @ClassName JacksonJsonSerializer
* @Description Jackson 序列化器
* @Author James.hu
* @Date 2023/3/14
**/
@Slf4j
public class JacksonJsonSerializer implements JsonSerializer{
// 用于键顺序序列化的ObjectMapper对象
private ObjectMapper linkedObjectMapper;
// 用于一般请求内容序列化
private ObjectMapper objectMapper;
public JacksonJsonSerializer() {
log.debug("[Cube] The SDK will use Jackson as the Json serialization implementation");
this.linkedObjectMapper = initLinkedObjectMapper();
this.objectMapper = initObjectMapper();
}
/** 初始化键排序的ObjectMapper
* 配置了Key排序(字典顺序)
* 配置了NON_NULL序列化策略
* @return
*/
private ObjectMapper initLinkedObjectMapper() {
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.configure(SerializationFeature.ORDER_MAP_ENTRIES_BY_KEYS,true);
objectMapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
return objectMapper;
}
private ObjectMapper initObjectMapper() {
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
objectMapper.disable(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES);
return objectMapper;
}
public String toLinkedJson(Object value) {
try {
return linkedObjectMapper.writeValueAsString(value);
} catch (JsonProcessingException e) {
throw new RuntimeException(e);
}
}
public String toJson(Object value) {
try {
return objectMapper.writeValueAsString(value);
} catch (JsonProcessingException e) {
throw new RuntimeException(e);
}
}
public Map convertMap(Object source) {
return objectMapper.convertValue(source, Map.class);
}
@Override
public T parse(byte[] source, Class clazz) {
try {
return objectMapper.readValue(source, clazz);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy