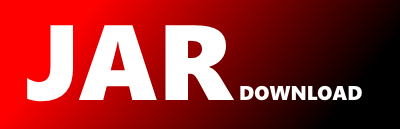
net.luminis.http3.sample.BodyHandlerWithStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of flupke Show documentation
Show all versions of flupke Show documentation
Java implementation of HTTP3 (RFC 9114): generic client / client library and HTTP3 server plugin for Kwik.
/*
* Copyright © 2019, 2020, 2021, 2022, 2023 Peter Doornbosch
*
* This file is part of Flupke, a HTTP3 client Java library
*
* Flupke is free software: you can redistribute it and/or modify it under
* the terms of the GNU Lesser General Public License as published by the
* Free Software Foundation, either version 3 of the License, or (at your option)
* any later version.
*
* Flupke is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License for
* more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*/
package net.luminis.http3.sample;
import net.luminis.http3.Http3Client;
import net.luminis.quic.log.Logger;
import net.luminis.quic.log.SysOutLogger;
import java.io.IOException;
import java.io.InputStream;
import java.net.URI;
import java.net.URISyntaxException;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.time.Duration;
public class BodyHandlerWithStream {
public static void main(String[] args) throws IOException, InterruptedException, URISyntaxException {
if (args.length != 1) {
System.err.println("Missing argument: server URL");
System.exit(1);
}
URI serverUrl = URI.create(args[0]);
HttpRequest request = HttpRequest.newBuilder()
.uri(serverUrl)
.header("User-Agent", "Flupke http3 library")
.timeout(Duration.ofSeconds(10))
.build();
Logger stdoutLogger = new SysOutLogger();
stdoutLogger.useRelativeTime(true);
stdoutLogger.logPackets(false);
HttpClient client = Http3Client.newBuilder()
.disableCertificateCheck()
.logger(stdoutLogger)
.connectTimeout(Duration.ofSeconds(3))
.build();
try {
long start = System.currentTimeMillis();
HttpResponse httpResponse = client.send(request, HttpResponse.BodyHandlers.ofInputStream());
byte[] content = httpResponse.body().readAllBytes();
int contentSize = content.length;
long end = System.currentTimeMillis();
System.out.println("Request (" + contentSize + " bytes) completed in " + (end - start) + " ms");
}
catch (IOException e) {
System.err.println("Request failed: " + e.getMessage());
e.printStackTrace();
}
catch (InterruptedException e) {
throw new RuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy