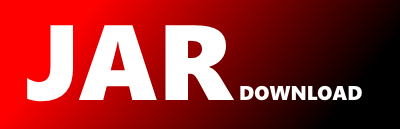
net.maizegenetics.plugindef.DataSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tassel Show documentation
Show all versions of tassel Show documentation
TASSEL is a software package to evaluate traits associations, evolutionary patterns, and linkage
disequilibrium.
/*
* DataSet.java
*
*/
package net.maizegenetics.plugindef;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Iterator;
import java.util.List;
/**
* This is a set of Datum.
*/
public class DataSet implements Serializable {
private static final long serialVersionUID = -5197800047652332969L;
private final List myList;
private final Plugin myCreator;
/**
* Creates a new instance of Datum
*
* @param list list of data elements.
* @param creator creating plugin.
*
*/
public DataSet(List list, Plugin creator) {
if (list == null) {
myList = new ArrayList();
} else {
myList = new ArrayList(list);
}
myCreator = creator;
}
/**
* Convenience constructor for a single Datum
*
* @param theDatum a single Datum
* @param creator creating plugin.
*
*/
public DataSet(Datum theDatum, Plugin creator) {
if (theDatum == null) {
myList = new ArrayList();
} else {
myList = new ArrayList();
myList.add(theDatum);
}
myCreator = creator;
}
/**
* Creates a new instance of Datum
*
* @param list list of data elements.
* @param creator creating plugin.
*
*/
public DataSet(Datum[] list, Plugin creator) {
if (list == null) {
myList = new ArrayList();
} else {
myList = new ArrayList(Arrays.asList(list));
}
myCreator = creator;
}
/**
* Combines multiple data sets.
*
* @param list list of data sets.
* @param creator creating plugin.
*
*/
public static DataSet getDataSet(List list, Plugin creator) {
List temp = new ArrayList();
if (list != null) {
Iterator itr = list.iterator();
while (itr.hasNext()) {
DataSet current = (DataSet) itr.next();
if (current != null) {
temp.addAll(current.getDataSet());
}
}
}
return new DataSet(temp, creator);
}
public static DataSet getDataSet(Object data) {
return new DataSet(new Datum("Datum", data, null), null);
}
public List getDataSet() {
return new ArrayList(myList);
}
public String toString() {
StringBuilder buffer = new StringBuilder();
buffer.append("DataSet...\n");
if (getCreator() != null) {
buffer.append("Creator: ");
buffer.append(getCreator().getClass().getName());
buffer.append("\n");
}
Iterator itr = myList.iterator();
while (itr.hasNext()) {
Datum current = (Datum) itr.next();
buffer.append("name: ");
buffer.append(current.getName());
buffer.append(" type: ");
buffer.append(current.getDataType());
buffer.append("\n");
}
return buffer.toString();
}
public Datum getData(int i) {
if (i < myList.size()) {
return myList.get(i);
} else {
return null;
}
}
public int getSize() {
return myList.size();
}
public Plugin getCreator() {
return myCreator;
}
public List getDataOfType(Class theClass) {
if (theClass == null) {
return null;
}
List list = new ArrayList();
Iterator itr = myList.iterator();
while (itr.hasNext()) {
Datum current = (Datum) itr.next();
if (theClass.isInstance(current.getData())) {
list.add(current);
}
}
return list;
}
public List getDataOfType(Class[] classes) {
if ((classes == null) || (classes.length == 0)) {
return new ArrayList(myList);
}
List result = null;
for (int i = 0, n = classes.length; i < n; i++) {
List current = getDataOfType(classes[i]);
if (result == null) {
result = current;
} else {
result.addAll(current);
}
}
return result;
}
public List getDataWithName(String name) {
if (name == null) {
return null;
}
List list = new ArrayList();
Iterator itr = myList.iterator();
while (itr.hasNext()) {
Datum current = (Datum) itr.next();
if (name.equals(current.getName())) {
list.add(current);
}
}
return list;
}
public List getDataWithName(String[] names) {
if ((names == null) || (names.length == 0)) {
return new ArrayList(myList);
}
List result = null;
for (int i = 0, n = names.length; i < n; i++) {
List current = getDataWithName(names[i]);
if (result == null) {
result = current;
} else {
result.addAll(current);
}
}
return result;
}
public List getDataOfTypeWithName(Class[] classes, String[] names) {
return (new DataSet(getDataOfType(classes), null)).getDataWithName(names);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy