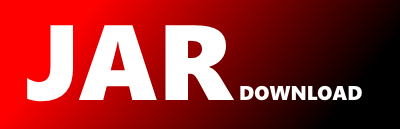
commonMain.ResultExtensions.kt Maven / Gradle / Ivy
/*
* Copyright 2019-2021 Mamoe Technologies and contributors.
*
* 此源代码的使用受 GNU AFFERO GENERAL PUBLIC LICENSE version 3 许可证的约束, 可以在以下链接找到该许可证.
* Use of this source code is governed by the GNU AGPLv3 license that can be found through the following link.
*
* https://github.com/mamoe/mirai/blob/master/LICENSE
*/
@file:JvmMultifileClass
@file:JvmName("MiraiUtils")
package net.mamoe.mirai.utils
import kotlin.reflect.KClass
@Suppress("INVISIBLE_MEMBER", "INVISIBLE_REFERENCE", "RESULT_CLASS_IN_RETURN_TYPE")
@kotlin.internal.InlineOnly
@kotlin.internal.LowPriorityInOverloadResolution
public inline fun Result.recoverCatchingSuppressed(transform: (exception: Throwable) -> R): Result {
return when (val exception = exceptionOrNull()) {
null -> this
else -> {
try {
Result.success(transform(exception))
} catch (e: Throwable) {
e.addSuppressed(exception)
Result.failure(e)
}
}
}
}
@Suppress("INVISIBLE_MEMBER", "INVISIBLE_REFERENCE", "RESULT_CLASS_IN_RETURN_TYPE")
@kotlin.internal.InlineOnly
@kotlin.internal.LowPriorityInOverloadResolution
public inline fun retryCatching(
n: Int,
except: KClass? = null,
block: (count: Int, lastException: Throwable?) -> R,
): Result {
require(n >= 0) {
"param n for retryCatching must not be negative"
}
var exception: Throwable? = null
repeat(n) {
try {
return Result.success(block(it, exception))
} catch (e: Throwable) {
if (except?.isInstance(e) == true) {
return Result.failure(e)
}
exception?.addSuppressed(e)
exception = e
}
}
return Result.failure(exception!!)
}
@Suppress("INVISIBLE_MEMBER", "INVISIBLE_REFERENCE", "RESULT_CLASS_IN_RETURN_TYPE")
@kotlin.internal.InlineOnly
@kotlin.internal.LowPriorityInOverloadResolution
public inline fun retryCatchingExceptions(
n: Int,
except: KClass? = null,
block: (count: Int, lastException: Throwable?) -> R,
): Result {
require(n >= 0) {
"param n for retryCatching must not be negative"
}
var exception: Throwable? = null
repeat(n) {
try {
return Result.success(block(it, exception))
} catch (e: Exception) {
if (except?.isInstance(e) == true) {
return Result.failure(e)
}
exception?.addSuppressed(e)
exception = e
}
}
return Result.failure(exception!!)
}
@Suppress("INVISIBLE_MEMBER", "INVISIBLE_REFERENCE", "RESULT_CLASS_IN_RETURN_TYPE")
@kotlin.internal.InlineOnly
public inline fun retryCatching(
n: Int,
except: KClass? = null,
block: () -> R,
): Result {
require(n >= 0) {
"param n for retryCatching must not be negative"
}
var exception: Throwable? = null
repeat(n) {
try {
return Result.success(block())
} catch (e: Throwable) {
if (except?.isInstance(e) == true) {
return Result.failure(e)
}
exception?.addSuppressed(e)
exception = e
}
}
return Result.failure(exception!!)
}
@Suppress("INVISIBLE_MEMBER", "INVISIBLE_REFERENCE", "RESULT_CLASS_IN_RETURN_TYPE")
@kotlin.internal.InlineOnly
public inline fun retryCatchingExceptions(
n: Int,
except: KClass? = null,
block: () -> R,
): Result {
require(n >= 0) {
"param n for retryCatching must not be negative"
}
var exception: Throwable? = null
repeat(n) {
try {
return Result.success(block())
} catch (e: Exception) {
if (except?.isInstance(e) == true) {
return Result.failure(e)
}
exception?.addSuppressed(e)
exception = e
}
}
return Result.failure(exception!!)
}
@Suppress("INVISIBLE_MEMBER", "INVISIBLE_REFERENCE", "RESULT_CLASS_IN_RETURN_TYPE")
@kotlin.internal.InlineOnly
public inline fun runCatchingExceptions(block: () -> R): Result {
return try {
Result.success(block())
} catch (e: Exception) {
Result.failure(e)
}
}
public inline fun Result.mapFailure(
block: (Throwable) -> Throwable,
): Result = onFailure {
return Result.failure(block(it))
}
public inline fun Result.onSuccessCatching(block: () -> Unit): Result {
if (isSuccess) {
runCatching(block).onFailure {
return@onSuccessCatching Result.failure(it)
}
}
return this
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy