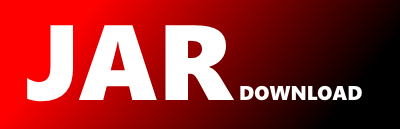
net.masterthought.cucumber.ReportBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cucumber-reporting Show documentation
Show all versions of cucumber-reporting Show documentation
Provides pretty html reports for Cucumber (Behaviour-Driven Development). It works by generating html from the
cucumber json report formatter. So can be used anywhere a json report is generated. Current use is in the
cucumber jenkins plugin and a maven mojo to generate the same report from mvn command line when running locally.
package net.masterthought.cucumber;
import java.io.File;
import java.io.IOException;
import java.util.List;
import org.apache.commons.io.FileUtils;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import net.masterthought.cucumber.generators.ErrorPage;
import net.masterthought.cucumber.generators.FeatureReportPage;
import net.masterthought.cucumber.generators.FeaturesOverviewPage;
import net.masterthought.cucumber.generators.StepsOverviewPage;
import net.masterthought.cucumber.generators.TagReportPage;
import net.masterthought.cucumber.generators.TagsOverviewPage;
import net.masterthought.cucumber.json.Feature;
import net.masterthought.cucumber.json.support.TagObject;
public class ReportBuilder {
private static final Logger LOG = LogManager.getLogger(ReportBuilder.class);
/**
* Page that should be displayed when the reports is generated. Shared between {@link FeaturesOverviewPage} and
* {@link ErrorPage}.
*/
public static final String HOME_PAGE = "feature-overview.html";
private ReportResult reportResult;
private final ReportParser reportParser;
private Configuration configuration;
private List jsonFiles;
public ReportBuilder(List jsonFiles, Configuration configuration) {
this.jsonFiles = jsonFiles;
this.configuration = configuration;
reportParser = new ReportParser(configuration);
}
/**
* Checks if all features pass.
*
* @return true if all feature pass otherwise false
*/
public boolean hasBuildPassed() {
return reportResult != null && reportResult.getAllFailedFeatures() == 0;
}
public void generateReports() {
try {
// first copy static resources so ErrorPage is displayed properly
copyStaticResources();
List features = reportParser.parseJsonResults(jsonFiles);
reportResult = new ReportResult(features);
generateAllPages();
// whatever happens we want to provide at least error page instead of empty report
} catch (Exception e) {
generateErrorPage(e);
}
}
private void copyStaticResources() {
copyResources("css", "reporting.css", "bootstrap.min.css");
copyResources("js", "jquery.min.js", "bootstrap.min.js", "jquery.tablesorter.min.js", "highcharts.js",
"highcharts-3d.js");
}
private void generateAllPages() {
new FeaturesOverviewPage(reportResult, configuration).generatePage();
for (Feature feature : reportResult.getAllFeatures()) {
new FeatureReportPage(reportResult, configuration, feature).generatePage();
}
new TagsOverviewPage(reportResult, configuration).generatePage();
for (TagObject tagObject : reportResult.getAllTags()) {
new TagReportPage(reportResult, configuration, tagObject).generatePage();
}
new StepsOverviewPage(reportResult, configuration).generatePage();
}
private void copyResources(String resourceLocation, String... resources) {
for (String resource : resources) {
File tempFile = new File(configuration.getReportDirectory().getAbsoluteFile(), resource);
// don't change this implementation unless you verified it works on Jenkins
try {
FileUtils.copyInputStreamToFile(
this.getClass().getResourceAsStream("/" + resourceLocation + "/" + resource), tempFile);
} catch (IOException e) {
throw new ValidationException(e);
}
}
}
private void generateErrorPage(Exception exception) {
LOG.info(exception);
ErrorPage errorPage = new ErrorPage(reportResult, configuration, exception, jsonFiles);
errorPage.generatePage();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy