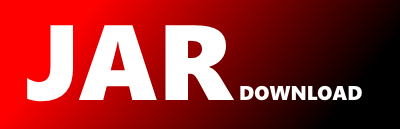
net.masterthought.cucumber.CucumberReportGeneratorMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-cucumber-reporting Show documentation
Show all versions of maven-cucumber-reporting Show documentation
This project provides a maven mojo for using cucumber-reporting to publish pretty html reports for Cucumber.
It works by generating html from the cucumber json report formatter.
So can be used anywhere a json report is generated. Current use is in the cucumber jenkins
plugin and a maven mojo to generate the same report from mvn command line when running locally.
package net.masterthought.cucumber;
import org.apache.commons.io.FileUtils;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import java.io.File;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
/**
* Goal which generates a Cucumber Report.
*
* @goal generate
* @phase verify
*/
public class CucumberReportGeneratorMojo extends AbstractMojo {
/**
* Name of the project.
*
* @parameter property="project.name"
* @required
*/
private String projectName;
/**
* Build number.
*
* @parameter property="build.number" default-value="1"
*/
private String buildNumber;
/**
* Location of the file.
*
* @parameter default-value="${project.build.directory}/cucumber-reports"
* @required
*/
private File outputDirectory;
/**
* Location of the file.
*
* @parameter default-value="${project.build.directory}/cucumber.json"
* @required
*/
private File cucumberOutput;
/**
* Skipped fails
*
* @parameter default-value="false"
* @required
*/
private Boolean skippedFails;
/**
* Undefined fails
*
* @parameter default-value="false"
* @required
*/
private Boolean undefinedFails;
/**
* Pending fails
*
* @parameter default-value="false"
* @required
*/
private Boolean pendingFails;
/**
* Missing fails
*
* @parameter default-value="false"
* @required
*/
private Boolean missingFails;
/**
* Skip check for failed build result
*
* @parameter default-value="true"
* @required
*/
private Boolean checkBuildResult;
/**
* Build reports from parallel tests
*
* @parameter property="true" default-value="false"
* @required
*/
private Boolean parallelTesting;
@Override
public void execute() throws MojoExecutionException {
if (!outputDirectory.exists()) {
outputDirectory.mkdirs();
}
List list = new ArrayList<>();
for (File jsonFile : cucumberFiles(cucumberOutput)) {
list.add(jsonFile.getAbsolutePath());
}
if (list.isEmpty()) {
getLog().warn(cucumberOutput.getAbsolutePath() + " does not exist.");
return;
}
try {
Configuration configuration = new Configuration(outputDirectory, projectName);
configuration.setBuildNumber(buildNumber);
configuration.setStatusFlags(skippedFails, pendingFails, undefinedFails, missingFails);
configuration.setParallelTesting(parallelTesting);
ReportBuilder reportBuilder = new ReportBuilder(list, configuration);
getLog().info("About to generate Cucumber report.");
reportBuilder.generateReports();
if (checkBuildResult) {
boolean buildResult = reportBuilder.hasBuildPassed();
if (!buildResult) {
throw new MojoExecutionException("BUILD FAILED - Check Report For Details");
}
}
} catch (Exception e) {
throw new MojoExecutionException("Error Found:", e);
}
}
// Normally, I'd keep this private and use mocks for testing the public contract.
// I'm not sure that the author wants to get that serious with this..
static Collection cucumberFiles(File file) throws MojoExecutionException {
if (!file.exists()) {
return Collections.emptyList();
}
if (file.isFile()) {
return Arrays.asList(file);
}
return FileUtils.listFiles(file, new String[] {"json"}, true);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy