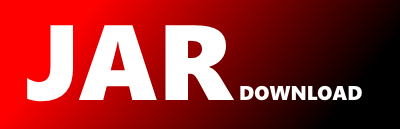
mikera.vectorz.impl.AMatrixViewVector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vectorz Show documentation
Show all versions of vectorz Show documentation
Fast double-precision vector and matrix maths library for Java, supporting N-dimensional numeric arrays.
package mikera.vectorz.impl;
import mikera.matrixx.AMatrix;
import mikera.vectorz.AVector;
/**
* Abstract Vector class representing a view over a matrix
*
* Supports arbitrary indexing into the underlying matrix
*
* @author Mike
*/
@SuppressWarnings("serial")
public abstract class AMatrixViewVector extends ASizedVector {
protected AMatrix source;
protected AMatrixViewVector(AMatrix source, int length) {
super(length);
this.source=source;
}
/**
* Calculates the row in the source matrix that corresponds to an index in this vector.
*
* This method is unsafe: caller is assumed to have checked that the index is in range
* @param i
* @return
*/
protected abstract int calcRow(int i);
/**
* Calculates the row in the source matrix that corresponds to an index in this vector
*
* This method is unsafe: caller is assumed to have checked that the index is in range
* @param i
* @return
*/
protected abstract int calcCol(int i);
@Override
public void addAt(int i, double v) {
int r=calcRow(i);
int c=calcCol(i);
source.unsafeSet(r,c,source.unsafeGet(r,c)+v);
}
@Override
public void set(int i, double value) {
checkIndex(i);
// we assume unsafe is OK, i.e. calculations are correct given correct i
source.unsafeSet(calcRow(i),calcCol(i),value);
}
@Override
public void unsafeSet(int i, double value) {
// we assume unsafe is OK, i.e. both i and calculations are correct
source.unsafeSet(calcRow(i),calcCol(i),value);
}
@Override
public double get(int i) {
checkIndex(i);
// we assume unsafe is OK, i.e. calculations are correct given correct i
return source.unsafeGet(calcRow(i),calcCol(i));
}
@Override
public double unsafeGet(int i) {
// we assume unsafe is OK, i.e. calculations are correct given correct i
return source.unsafeGet(calcRow(i),calcCol(i));
}
@Override
public void getElements(double[] data, int offset) {
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy