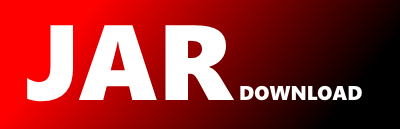
mikera.matrixx.impl.QuadtreeMatrix Maven / Gradle / Ivy
package mikera.matrixx.impl;
import mikera.arrayz.ISparse;
import mikera.matrixx.AMatrix;
import mikera.vectorz.AVector;
/**
* A matrix implemented as a quadtree of submatrices.
*
* Useful for large matrices with heirarchical structure where large regions are either fully sparse
* or of a specialised subtype (e.g. a diagonal matrix).
*
* @author Mike
*
*/
public class QuadtreeMatrix extends ABlockMatrix implements ISparse {
private static final long serialVersionUID = -7267626771473908891L;
// Quadtree subcompoents
private final AMatrix c00, c01, c10, c11;
private final int rowSplit;
private final int columnSplit;
private QuadtreeMatrix(AMatrix c00, AMatrix c01, AMatrix c10, AMatrix c11) {
super(c00.rowCount()+c10.rowCount(),c00.columnCount()+c01.columnCount());
this.c00=c00;
this.c01=c01;
this.c10=c10;
this.c11=c11;
this.rowSplit= c00.rowCount();
this.columnSplit=c00.columnCount();
}
public static QuadtreeMatrix wrap(AMatrix c00, AMatrix c01, AMatrix c10, AMatrix c11) {
if (c00.rowCount()!=c01.rowCount()) throw new IllegalArgumentException("Mismtached submatrix size");
if (c10.rowCount()!=c11.rowCount()) throw new IllegalArgumentException("Mismtached submatrix size");
if (c00.columnCount()!=c10.columnCount()) throw new IllegalArgumentException("Mismtached submatrix size");
if (c01.columnCount()!=c11.columnCount()) throw new IllegalArgumentException("Mismtached submatrix size");
return new QuadtreeMatrix(c00,c01,c10,c11);
}
public static QuadtreeMatrix create(AMatrix c00, AMatrix c01, AMatrix c10, AMatrix c11) {
return wrap(c00.copy(),c01.copy(),c10.copy(),c11.copy());
}
@Override
public boolean isFullyMutable() {
return c00.isFullyMutable()&&c01.isFullyMutable()&&c10.isFullyMutable()&&(c11.isFullyMutable());
}
@Override
public boolean isMutable() {
return (c00.isMutable())||(c01.isMutable())||(c10.isMutable())||(c11.isMutable());
}
@Override
public boolean isZero() {
return c00.isZero()&&c01.isZero()&&c10.isZero()&&(c11.isZero());
}
@Override
public boolean isDiagonal() {
if (!isSquare()) return false;
if (columnSplit==rowSplit) {
return (c01.isZero())&&(c10.isZero())&&(c00.isDiagonal())&&(c11.isDiagonal());
} else {
return super.isDiagonal();
}
}
@Override
public double get(int row, int column) {
checkIndex(row,column);
return unsafeGet(row,column);
}
@Override
public void set(int row, int column, double value) {
checkIndex(row,column);
unsafeSet(row,column,value);
}
@Override
public double unsafeGet(int row, int column) {
if (row
© 2015 - 2025 Weber Informatics LLC | Privacy Policy