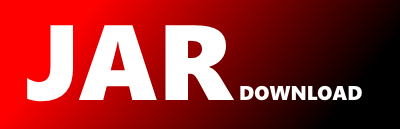
mikera.indexz.AIndex Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vectorz Show documentation
Show all versions of vectorz Show documentation
Fast double-precision vector and matrix maths library for Java, supporting N-dimensional numeric arrays.
package mikera.indexz;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import mikera.indexz.impl.IndexIterator;
import mikera.vectorz.Tools;
/**
* Abstract base class for a list of integer indexes
*
* @author Mike
*/
@SuppressWarnings("serial")
public abstract class AIndex implements Serializable, Cloneable, Comparable, Iterable {
// ===================================
// Abstract interface
/**
* Gets the index value at position i
*
* @param i
* @return
*/
public abstract int get(int i);
/**
* Returns the length of this index list
*
* @return
*/
public abstract int length();
// ===================================
// Common implementations
public abstract void set(int i, int value);
public boolean isFullyMutable() {
return false;
}
public void copyTo(int[] array, int offset) {
int len=length();
for (int i=0; i toList() {
int len=length();
ArrayList al=new ArrayList(len);
for (int i=0; imax) max=x;
}
return max;
}
/**
* Returns true if this index is sorted (in increasing order)
* @return
*/
public boolean isSorted() {
int len=length();
for (int i=1; iget(i)) return false;
}
return true;
}
/**
* Returns true if this index is distinct and sorted (in strictly increasing order)
* @return
*/
public boolean isDistinctSorted() {
int len=length();
for (int i=1; i=get(i)) return false;
}
return true;
}
/**
* Sorts the index in place. May not be supported by some AIndex implementations
* e.g. if the index is immutable.
*/
public void sort() {
throw new UnsupportedOperationException();
}
/**
* Returns true if this Index contains only distinct integer indices
* @return
*/
public boolean isDistinct() {
HashSet hs=new HashSet();
int len=length();
for (int i=0; i=length)) return false;
}
return true;
}
/**
* Returns true if the index contains a specific value.
* Performs a full scan of the index (need not be in sorted order)
* @param index
* @return
*/
public boolean contains(int index) {
int len=length();
for (int i=0; i0) {
sb.append(get(0));
for (int i = 1; i < length; i++) {
sb.append(',');
sb.append(get(i));
}
}
sb.append(']');
return sb.toString();
}
@Override
public AIndex clone() {
try {
return (AIndex) super.clone();
} catch (CloneNotSupportedException e) {
throw new Error(e);
}
}
@Override
public int compareTo(AIndex a) {
int len=length();
int alen=a.length();
if (len!=alen) return len-alen;
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy