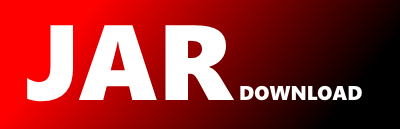
net.mindengine.galen.validation.ValidationErrorException Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of galen Show documentation
Show all versions of galen Show documentation
A library for layout testing of websites
/*******************************************************************************
* Copyright 2014 Ivan Shubin http://mindengine.net
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
******************************************************************************/
package net.mindengine.galen.validation;
import java.util.LinkedList;
import java.util.List;
import static java.util.Arrays.asList;
public class ValidationErrorException extends Exception {
private List errorAreas;
private List errorMessages;
private ImageComparison imageComparison;
public ValidationErrorException() {
super();
}
public ValidationErrorException(List errorAreas, List errorMessages) {
this.errorAreas = errorAreas;
this.errorMessages = errorMessages;
}
public ValidationErrorException(String paramString, Throwable paramThrowable) {
super(paramString, paramThrowable);
withMessage(paramString);
}
public ValidationErrorException withMessage(String message) {
if (errorMessages == null) {
errorMessages = new LinkedList();
}
errorMessages.add(message);
return this;
}
public ValidationErrorException withErrorArea(ErrorArea errorArea) {
if (errorAreas == null) {
errorAreas = new LinkedList();
}
errorAreas.add(errorArea);
return this;
}
public ValidationErrorException(String paramString) {
super(paramString);
withMessage(paramString);
}
public ValidationErrorException(Throwable paramThrowable) {
super(paramThrowable);
setErrorMessages(asList(paramThrowable.getClass().getName() + ": " + paramThrowable.getMessage()));
}
public List getErrorMessages() {
return errorMessages;
}
public void setErrorMessages(List errorMessages) {
this.errorMessages = errorMessages;
}
public List getErrorAreas() {
return errorAreas;
}
public void setErrorAreas(List errorAreas) {
this.errorAreas = errorAreas;
}
/**
*
*/
private static final long serialVersionUID = -1566513657187992205L;
public ValidationErrorException withMessages(List messages) {
setErrorMessages(messages);
return this;
}
public ValidationError asValidationError() {
return new ValidationError(this.getErrorAreas(), this.getErrorMessages(), this.getImageComparison());
}
public ImageComparison getImageComparison() {
return imageComparison;
}
public void setImageComparison(ImageComparison imageComparison) {
this.imageComparison = imageComparison;
}
public ValidationErrorException withImageComparison(ImageComparison imageComparison) {
setImageComparison(imageComparison);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy