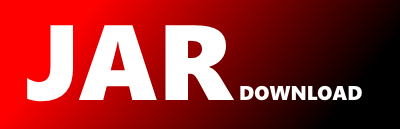
net.minestom.server.entity.EntityView Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of minestom-snapshots Show documentation
Show all versions of minestom-snapshots Show documentation
1.20.4 Lightweight Minecraft server
package net.minestom.server.entity;
import it.unimi.dsi.fastutil.ints.Int2ObjectOpenHashMap;
import it.unimi.dsi.fastutil.ints.IntIterator;
import it.unimi.dsi.fastutil.ints.IntOpenHashSet;
import it.unimi.dsi.fastutil.ints.IntSet;
import net.minestom.server.ServerFlag;
import net.minestom.server.coordinate.Point;
import net.minestom.server.instance.EntityTracker;
import net.minestom.server.instance.Instance;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.util.*;
import java.util.concurrent.atomic.AtomicIntegerFieldUpdater;
import java.util.function.Consumer;
import java.util.function.Predicate;
final class EntityView {
private static final int RANGE = ServerFlag.ENTITY_VIEW_DISTANCE;
private final Entity entity;
private final Set manualViewers = new HashSet<>();
// Decide if this entity should be viewable to X players
public final Option viewableOption;
// Decide if this entity should view X entities
public final Option viewerOption;
final Set set = new SetImpl();
private final Object mutex = this;
private volatile TrackedLocation trackedLocation;
public EntityView(Entity entity) {
this.entity = entity;
this.viewableOption = new Option<>(EntityTracker.Target.PLAYERS, Entity::autoViewEntities,
player -> {
// Add viewable
var lock1 = player.getEntityId() < entity.getEntityId() ? player : entity;
var lock2 = lock1 == entity ? player : entity;
synchronized (lock1.viewEngine.mutex) {
synchronized (lock2.viewEngine.mutex) {
if (!entity.viewEngine.viewableOption.predicate(player) ||
!player.viewEngine.viewerOption.predicate(entity)) return;
entity.viewEngine.viewableOption.register(player);
player.viewEngine.viewerOption.register(entity);
}
}
// Entity#updateNewViewer handles calling itself for passengers
if (entity.getVehicle() != null) return;
entity.updateNewViewer(player);
},
player -> {
// Remove viewable
var lock1 = player.getEntityId() < entity.getEntityId() ? player : entity;
var lock2 = lock1 == entity ? player : entity;
synchronized (lock1.viewEngine.mutex) {
synchronized (lock2.viewEngine.mutex) {
entity.viewEngine.viewableOption.unregister(player);
player.viewEngine.viewerOption.unregister(entity);
}
}
entity.updateOldViewer(player);
});
this.viewerOption = new Option<>(EntityTracker.Target.ENTITIES, Entity::isAutoViewable,
entity instanceof Player player ? e -> e.viewEngine.viewableOption.addition.accept(player) : null,
entity instanceof Player player ? e -> e.viewEngine.viewableOption.removal.accept(player) : null);
}
public void updateTracker(@Nullable Instance instance, @NotNull Point point) {
this.trackedLocation = instance != null ? new TrackedLocation(instance, point) : null;
}
record TrackedLocation(Instance instance, Point point) {
}
public boolean manualAdd(@NotNull Player player) {
if (player == this.entity) return false;
synchronized (mutex) {
if (manualViewers.add(player)) {
viewableOption.bitSet.add(player.getEntityId());
return true;
}
return false;
}
}
public boolean manualRemove(@NotNull Player player) {
if (player == this.entity) return false;
synchronized (mutex) {
if (manualViewers.remove(player)) {
viewableOption.bitSet.remove(player.getEntityId());
return true;
}
return false;
}
}
public void forManuals(@NotNull Consumer consumer) {
synchronized (mutex) {
Set manualViewersCopy = Set.copyOf(this.manualViewers);
manualViewersCopy.forEach(consumer);
}
}
public boolean hasPredictableViewers() {
// Verify if this entity's viewers can be predicted from surrounding entities
synchronized (mutex) {
return viewableOption.isAuto() && viewableOption.predicate == null && manualViewers.isEmpty();
}
}
public void handleAutoViewAddition(Entity entity) {
handleAutoView(entity, viewerOption.addition, viewableOption.addition);
}
public void handleAutoViewRemoval(Entity entity) {
handleAutoView(entity, viewerOption.removal, viewableOption.removal);
}
private void handleAutoView(Entity entity, Consumer viewer, Consumer viewable) {
if (this.entity instanceof Player && viewerOption.isAuto() && entity.isAutoViewable()) {
if (viewer != null) viewer.accept(entity); // Send packet to this player
}
if (entity instanceof Player player && player.autoViewEntities() && viewableOption.isAuto()) {
if (viewable != null) viewable.accept(player); // Send packet to the range-visible player
}
}
public final class Option {
@SuppressWarnings("rawtypes")
private static final AtomicIntegerFieldUpdater UPDATER = AtomicIntegerFieldUpdater.newUpdater(EntityView.Option.class, "auto");
// Entities that should be tracked from this option
private final EntityTracker.Target target;
// The condition that must be met for this option to be considered auto.
private final Predicate loopPredicate;
// The consumers to be called when an entity is added/removed.
public final Consumer addition, removal;
// Contains all the auto-entity ids that are viewable by this option.
public final IntSet bitSet = new IntOpenHashSet();
// 1 if auto, 0 if manual
private volatile int auto = 1;
// The custom rule used to determine if an entity is viewable.
// null if auto-viewable
private Predicate predicate = null;
public Option(EntityTracker.Target target, Predicate loopPredicate,
Consumer addition, Consumer removal) {
this.target = target;
this.loopPredicate = loopPredicate;
this.addition = addition;
this.removal = removal;
}
public boolean isAuto() {
return auto == 1;
}
public boolean predicate(T entity) {
final Predicate predicate = this.predicate;
return predicate == null || predicate.test(entity);
}
public boolean isRegistered(T entity) {
return bitSet.contains(entity.getEntityId());
}
public void register(T entity) {
assert entity.getInstance() != null : "Instance-less entity shouldn't be registered as viewer";
this.bitSet.add(entity.getEntityId());
}
public void unregister(T entity) {
this.bitSet.remove(entity.getEntityId());
}
public void updateAuto(boolean autoViewable) {
final boolean previous = UPDATER.getAndSet(this, autoViewable ? 1 : 0) == 1;
if (previous != autoViewable) {
synchronized (mutex) {
if (autoViewable) update(loopPredicate, addition);
else update(this::isRegistered, removal);
}
}
}
public void updateRule(Predicate predicate) {
synchronized (mutex) {
this.predicate = predicate;
updateRule0(predicate);
}
}
public void updateRule() {
synchronized (mutex) {
updateRule0(predicate);
}
}
void updateRule0(Predicate predicate) {
if (predicate == null) {
update(loopPredicate, entity -> {
if (!isRegistered(entity)) addition.accept(entity);
});
} else {
update(loopPredicate, entity -> {
final boolean result = predicate.test(entity);
if (result != isRegistered(entity)) {
if (result) addition.accept(entity);
else removal.accept(entity);
}
});
}
}
private void update(Predicate visibilityPredicate,
Consumer action) {
references().forEach(entity -> {
if (entity == EntityView.this.entity || !visibilityPredicate.test(entity)) return;
if (entity instanceof Player player && manualViewers.contains(player)) return;
if (entity.getVehicle() != null) return;
action.accept(entity);
});
}
private int lastSize;
private Collection references() {
final TrackedLocation trackedLocation = EntityView.this.trackedLocation;
if (trackedLocation == null) return List.of();
final Instance instance = trackedLocation.instance();
final Point point = trackedLocation.point();
Int2ObjectOpenHashMap entityMap = new Int2ObjectOpenHashMap<>(lastSize);
instance.getEntityTracker().nearbyEntitiesByChunkRange(point, RANGE, target,
(entity) -> entityMap.putIfAbsent(entity.getEntityId(), entity));
this.lastSize = entityMap.size();
return entityMap.values();
}
}
final class SetImpl extends AbstractSet {
@Override
public @NotNull Iterator iterator() {
List players;
synchronized (mutex) {
var bitSet = viewableOption.bitSet;
if (bitSet.isEmpty()) return Collections.emptyIterator();
Instance instance = entity.getInstance();
if (instance == null) return Collections.emptyIterator();
players = new ArrayList<>(bitSet.size());
for (IntIterator it = bitSet.intIterator(); it.hasNext(); ) {
final int id = it.nextInt();
final Player player = (Player) instance.getEntityById(id);
if (player != null) players.add(player);
}
}
return players.iterator();
}
@Override
public int size() {
synchronized (mutex) {
return viewableOption.bitSet.size();
}
}
@Override
public boolean isEmpty() {
synchronized (mutex) {
return viewableOption.bitSet.isEmpty();
}
}
@Override
public boolean contains(Object o) {
if (!(o instanceof Player player)) return false;
synchronized (mutex) {
return viewableOption.isRegistered(player);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy