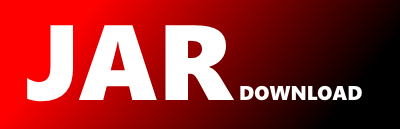
net.mingsoft.freight.action.AreaAction Maven / Gradle / Ivy
/**
The MIT License (MIT) * Copyright (c) 2016 铭飞科技(mingsoft.net)
* Permission is hereby granted, free of charge, to any person obtaining a copy of
* this software and associated documentation files (the "Software"), to deal in
* the Software without restriction, including without limitation the rights to
* use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of
* the Software, and to permit persons to whom the Software is furnished to do so,
* subject to the following conditions:
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS
* FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR
* COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER
* IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
* CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package net.mingsoft.freight.action;
import com.alibaba.fastjson.JSONArray;
import net.mingsoft.base.entity.ResultData;
import net.mingsoft.basic.action.BaseAction;
import net.mingsoft.basic.annotation.LogAnn;
import net.mingsoft.basic.bean.CityBean;
import net.mingsoft.basic.bean.EUListBean;
import net.mingsoft.basic.biz.ICityBiz;
import net.mingsoft.basic.constant.e.BusinessTypeEnum;
import net.mingsoft.basic.util.BasicUtil;
import net.mingsoft.freight.bean.AreaBean;
import net.mingsoft.freight.biz.IAreaBiz;
import net.mingsoft.freight.biz.IAreaDetailBiz;
import net.mingsoft.freight.entity.AreaDetailEntity;
import net.mingsoft.freight.entity.AreaEntity;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.util.List;
/**
* 运费模块区域的设置、添加、修改、删除
* @author 铭飞开源团队
* @date 2019年7月16日
*/
@Controller
@RequestMapping("/${ms.manager.path}/freight/area")
public class AreaAction extends BaseAction {
/**
* 注入区域基础业务层
*/
@Autowired
private IAreaBiz areaBiz;
/**
* 注入城市基础业务层
*/
@Autowired
public ICityBiz cityBiz;
/**
* 注入区域详情业务层
*/
@Autowired
private IAreaDetailBiz areaDetailBiz;
/**
* 加载页面显示所有区域信息
* @param request
* @return
*/
@GetMapping("/index")
public String index(HttpServletResponse response,HttpServletRequest request){
//通过查询城市的基础数据
List list = cityBiz.queryForTree(2,null);
String categoryJson = JSONArray.toJSONString(list);
request.setAttribute("categoryJson", categoryJson);
return "/freight/area/index";
}
/**
* 查询所有区域
* @param response
* @param request
*/
@RequestMapping("/areaList")
@ResponseBody
public ResultData areaList(HttpServletResponse response, HttpServletRequest request){
//左侧列表
List areas = areaBiz.queryAll();
return ResultData.build().success(areas);
}
/**
* 查询区域列表
* @param area
* @param response
* @param request
*/
@RequestMapping("/list")
@ResponseBody
public ResultData list(@ModelAttribute AreaBean area,HttpServletResponse response,HttpServletRequest request){
//左侧列表
BasicUtil.startPage();
List areas = areaBiz.queryByExpress(area);
EUListBean _list = new EUListBean(areas, (int) BasicUtil.endPage(areas).getTotal());
return ResultData.build().success(_list);
}
/**
* 保存区域信息
* @param area
* @param response
* @param request
*/
@LogAnn(title = "保存区域信息",businessType= BusinessTypeEnum.INSERT)
@RequestMapping("/save")
@ResponseBody
public ResultData save(@ModelAttribute AreaEntity area, HttpServletResponse response, HttpServletRequest request){
areaBiz.saveEntity(area);
return ResultData.build().success();
}
/**
* 批量保存区域信息
* @param area
* @param response
* @param request
*/
@LogAnn(title = "批量保存区域信息",businessType= BusinessTypeEnum.INSERT)
@RequestMapping("/saveBatch")
@ResponseBody
public ResultData saveBatch(@RequestBody List area, HttpServletResponse response, HttpServletRequest request){
for (int i=0;i area, HttpServletResponse response, HttpServletRequest request){
for (int i=0;i 0){
//修改areaEntity
areaEntity.setFaId(area.get(i).getFaId());
areaBiz.updateEntity(areaEntity);
//修改AreaDetailEntity
AreaDetailEntity areaDetailEntity = area.get(i).getAreaDetail();
areaDetailBiz.updateEntity(areaDetailEntity);
} else {
//保存areaEntity
areaBiz.saveEntity(areaEntity);
//修改AreaDetailEntity
AreaDetailEntity areaDetailEntity = area.get(i).getAreaDetail();
areaDetailEntity.setFadAreaId(areaEntity.getFaId());
areaDetailBiz.saveEntity(areaDetailEntity);
}
}
return ResultData.build().success();
}
/**
* 修改区域信息
* @param area
* @param response
* @param request
*/
@LogAnn(title = "修改区域信息",businessType= BusinessTypeEnum.UPDATE)
@RequestMapping("/update")
@ResponseBody
public ResultData update(@ModelAttribute AreaEntity area, HttpServletResponse response, HttpServletRequest request){
areaBiz.updateEntity(area);
return ResultData.build().success();
}
/**
* 删除区域功能
* @param area
* @param response
* @param request
*/
@LogAnn(title = "删除区域功能",businessType= BusinessTypeEnum.DELETE)
@RequestMapping("/delete")
@ResponseBody
public ResultData delete(@ModelAttribute AreaEntity area, HttpServletResponse response, HttpServletRequest request){
int[] ids = BasicUtil.getInts("ids",",");
//删除freight_area_detail表和freight_area表
areaBiz.deleteArea(ids);
return ResultData.build().success();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy