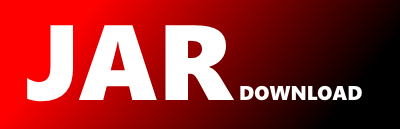
net.mingsoft.freight.action.CompanyAction Maven / Gradle / Ivy
package net.mingsoft.freight.action;
import com.alibaba.fastjson.JSON;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiImplicitParam;
import io.swagger.annotations.ApiImplicitParams;
import io.swagger.annotations.ApiOperation;
import net.mingsoft.base.entity.BaseEntity;
import net.mingsoft.base.entity.ResultData;
import net.mingsoft.base.util.JSONObject;
import net.mingsoft.basic.annotation.LogAnn;
import net.mingsoft.basic.bean.EUListBean;
import net.mingsoft.basic.constant.e.BusinessTypeEnum;
import net.mingsoft.basic.util.BasicUtil;
import net.mingsoft.freight.biz.ICompanyBiz;
import net.mingsoft.freight.entity.CompanyEntity;
import org.apache.commons.lang3.StringUtils;
import org.apache.shiro.authz.annotation.RequiresPermissions;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.validation.BindingResult;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import springfox.documentation.annotations.ApiIgnore;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.File;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.regex.Pattern;
/**
* 快递公司管理控制层
* @author 小伍io678()
* 创建日期:2019-11-6 15:23:42
* 历史修订:
*/
@Api(value = "快递公司接口")
@Controller
@RequestMapping("/${ms.manager.path}/freight/company")
public class CompanyAction extends net.mingsoft.freight.action.BaseAction{
/**
* 注入快递公司业务层
*/
@Autowired
private ICompanyBiz companyBiz;
/**
* 寻找文件正则
*/
private Pattern filePattern = Pattern.compile("(src|href)=\"(upload/.*?(png|jpg|gif))");
/**
* 返回主界面index
*/
@GetMapping("/index")
public String index(HttpServletResponse response,HttpServletRequest request){
return "/freight/company/index";
}
/**
* 查询快递公司列表
* @param company 快递公司实体
* company参数包含字段信息参考:
* companyName 快递公司名称
* companyPic 快递公司logo
* companyDesc 公司简介
* id 编号
* 返回
* [
* {
* companyName: 快递公司名称
* companyPic: 快递公司logo
* companyDesc: 公司简介
* id: 编号
* }
* ]
*/
@ApiOperation(value = "查询快递公司列表接口")
@ApiImplicitParams({
@ApiImplicitParam(name = "companyName", value = "快递公司名称", required =false,paramType="query"),
@ApiImplicitParam(name = "companyPic", value = "快递公司logo", required =false,paramType="query"),
@ApiImplicitParam(name = "companyDesc", value = "公司简介", required =false,paramType="query"),
@ApiImplicitParam(name = "createBy", value = "创建人", required =false,paramType="query"),
@ApiImplicitParam(name = "createDate", value = "创建时间", required =false,paramType="query"),
@ApiImplicitParam(name = "updateBy", value = "修改人", required =false,paramType="query"),
@ApiImplicitParam(name = "updateDate", value = "修改时间", required =false,paramType="query"),
@ApiImplicitParam(name = "del", value = "删除标记", required =false,paramType="query"),
@ApiImplicitParam(name = "id", value = "编号", required =false,paramType="query"),
})
@GetMapping("/list")
@ResponseBody
public ResultData list(@ModelAttribute @ApiIgnore CompanyEntity company,HttpServletResponse response, HttpServletRequest request,@ApiIgnore ModelMap model,BindingResult result) {
BasicUtil.startPage();
List companyList = companyBiz.query(company);
return ResultData.build().success(new EUListBean(companyList,(int)BasicUtil.endPage(companyList).getTotal()));
}
/**
* 返回编辑界面company_form
*/
@GetMapping("/form")
public String form(@ModelAttribute CompanyEntity company,HttpServletResponse response,HttpServletRequest request,ModelMap model){
if(company.getId()!=null){
BaseEntity companyEntity = companyBiz.getEntity(Integer.parseInt(company.getId()));
model.addAttribute("companyEntity",companyEntity);
}
return "/freight/company/form";
}
/**
* 获取快递公司
* @param company 快递公司实体
* company参数包含字段信息参考:
* companyName 快递公司名称
* companyPic 快递公司logo
* companyDesc 公司简介
* id 编号
* 返回
* {
* companyName: 快递公司名称
* companyPic: 快递公司logo
* companyDesc: 公司简介
* id: 编号
* }
*/
@ApiOperation(value = "获取快递公司列表接口")
@ApiImplicitParam(name = "id", value = "编号", required =true,paramType="query")
@GetMapping("/get")
@ResponseBody
public ResultData get(@ModelAttribute @ApiIgnore CompanyEntity company,HttpServletResponse response, HttpServletRequest request,@ApiIgnore ModelMap model){
if(company.getId()==null) {
return null;
}
CompanyEntity _company = (CompanyEntity)companyBiz.getEntity(Integer.parseInt(company.getId()));
return ResultData.build().success(_company);
}
/**
* 保存快递公司实体
* @param company 快递公司实体
* company参数包含字段信息参考:
* companyName 快递公司名称
* companyPic 快递公司logo
* companyDesc 公司简介
* id 编号
* 返回
* {
* companyName: 快递公司名称
* companyPic: 快递公司logo
* companyDesc: 公司简介
* id: 编号
* }
*/
@ApiOperation(value = "保存快递公司列表接口")
@ApiImplicitParams({
@ApiImplicitParam(name = "companyName", value = "快递公司名称", required =false,paramType="query"),
@ApiImplicitParam(name = "companyPic", value = "快递公司logo", required =false,paramType="query"),
@ApiImplicitParam(name = "companyDesc", value = "公司简介", required =false,paramType="query"),
@ApiImplicitParam(name = "createBy", value = "创建人", required =false,paramType="query"),
@ApiImplicitParam(name = "createDate", value = "创建时间", required =false,paramType="query"),
@ApiImplicitParam(name = "updateBy", value = "修改人", required =false,paramType="query"),
@ApiImplicitParam(name = "updateDate", value = "修改时间", required =false,paramType="query"),
@ApiImplicitParam(name = "del", value = "删除标记", required =false,paramType="query"),
@ApiImplicitParam(name = "id", value = "编号", required =false,paramType="query"),
})
@LogAnn(title = "保存快递公司列表接口",businessType= BusinessTypeEnum.INSERT)
@PostMapping("/save")
@ResponseBody
@RequiresPermissions("freight:company:save")
public ResultData save(@ModelAttribute @ApiIgnore CompanyEntity company, HttpServletResponse response, HttpServletRequest request,BindingResult result) {
companyBiz.saveEntity(company);
return ResultData.build().success(company);
}
/**
* @param company 快递公司实体
* company参数包含字段信息参考:
* id:多个id直接用逗号隔开,例如id=1,2,3,4
* 批量删除快递公司
* 返回
* {code:"错误编码",
* result:"true|false",
* resultMsg:"错误信息"
* }
*/
@ApiOperation(value = "批量删除快递公司列表接口")
@LogAnn(title = "批量删除快递公司列表接口",businessType= BusinessTypeEnum.DELETE)
@PostMapping("/delete")
@ResponseBody
@RequiresPermissions("freight:company:del")
public ResultData delete(@RequestBody List companys,HttpServletResponse response, HttpServletRequest request) {
int[] ids = new int[companys.size()];
for(int i = 0;icompany参数包含字段信息参考:
* companyName 快递公司名称
* companyPic 快递公司logo
* companyDesc 公司简介
* id 编号
* 返回
* {
* companyName: 快递公司名称
* companyPic: 快递公司logo
* companyDesc: 公司简介
* id: 编号
* }
*/
@ApiOperation(value = "更新快递公司列表接口")
@ApiImplicitParams({
@ApiImplicitParam(name = "id", value = "编号", required =true,paramType="query"),
@ApiImplicitParam(name = "companyName", value = "快递公司名称", required =false,paramType="query"),
@ApiImplicitParam(name = "companyPic", value = "快递公司logo", required =false,paramType="query"),
@ApiImplicitParam(name = "companyDesc", value = "公司简介", required =false,paramType="query"),
@ApiImplicitParam(name = "createBy", value = "创建人", required =false,paramType="query"),
@ApiImplicitParam(name = "createDate", value = "创建时间", required =false,paramType="query"),
@ApiImplicitParam(name = "updateBy", value = "修改人", required =false,paramType="query"),
@ApiImplicitParam(name = "updateDate", value = "修改时间", required =false,paramType="query"),
@ApiImplicitParam(name = "del", value = "删除标记", required =false,paramType="query"),
@ApiImplicitParam(name = "id", value = "编号", required =false,paramType="query"),
})
@LogAnn(title = "更新快递公司列表接口",businessType= BusinessTypeEnum.UPDATE)
@PostMapping("/update")
@ResponseBody
@RequiresPermissions("freight:company:update")
public ResultData update(@ModelAttribute @ApiIgnore CompanyEntity company, HttpServletResponse response,
HttpServletRequest request) {
companyBiz.updateEntity(company);
return ResultData.build().success(company);
}
@PostMapping("/deleFile")
@ResponseBody
@RequiresPermissions("freight:company:deleFile")
public void deleFile(@ModelAttribute @ApiIgnore CompanyEntity company, HttpServletResponse response,
HttpServletRequest request) {
List files=new ArrayList<>();
List entitys = companyBiz.query(null);
for (CompanyEntity entity : entitys) {
String CompanyPic = entity.getCompanyPic();
if(!StringUtils.isBlank(CompanyPic)){
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy