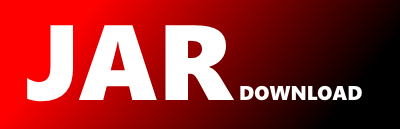
com.mingsoft.util.RegexUtil Maven / Gradle / Ivy
The newest version!
/**
The MIT License (MIT) * Copyright (c) 2016 铭飞科技(mingsoft.net)
* Permission is hereby granted, free of charge, to any person obtaining a copy of
* this software and associated documentation files (the "Software"), to deal in
* the Software without restriction, including without limitation the rights to
* use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of
* the Software, and to permit persons to whom the Software is furnished to do so,
* subject to the following conditions:
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS
* FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR
* COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER
* IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
* CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
package com.mingsoft.util;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* 解析模版中正则表达式的工具类
* @author 王天培QQ:78750478
* @version
* 版本号:100-000-000
* 创建日期:2012-03-15
* 历史修订:
*/
public class RegexUtil {
/**
* 返回所有匹配的结果,并且是第find组
*
* @param source
* 模版
* @param regex
* 标签
* @param find
* 第几组 0:返回所有
* @return 返回匹配一次的值
*/
public static String parseFirst(String source, String regex, int find) {
String content = null;
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(source);
if (matcher.find()) {
content = matcher.group(find);
}
return content;
}
/**
* 从find位置开始匹配所有返回
*
* @param source
* 模版
* @param regex
* 标签
* @param find
* 第几组 0:返回所有
* @return 返回匹配一次的值
*/
public static List parseAll(String source, String regex, int find) {
List content = new ArrayList();
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(source);
while (matcher.find()) {
content.add(matcher.group(find));
}
return content;
}
/**
* 从find位置开始匹配提供返回
*
* @param source
* 模版
* @param regex
* 标签
* @param newContent
* 新的内容
* @return 返回替换好的内容
*/
public static String replaceAll(String source, String regex, String newContent) {
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(source);
while (matcher.find()) {
source = matcher.replaceAll(Matcher.quoteReplacement(newContent.toString().replace("\\", "/")));
}
return source;
}
/**
* 替换第一个
*
* @param source
* 原始内容
* @param regex
* 正则
* @param newContent
* 新内容
* @return 返回替换后的内容
*/
public static String replaceFirst(String source, String regex, String newContent) {
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(source);
if (matcher.find()) {
source = matcher.replaceFirst(Matcher.quoteReplacement(newContent));
}
return source;
}
/**
* 查询规则匹配次数
*
* @param source
* 模版
* @param regex
* 标签
* @return 返回标签的数量
*/
public static int count(String source, String regex) {
Pattern patternList = Pattern.compile(regex);
Matcher matcherList = patternList.matcher(source);
int i = 0;
while (matcherList.find()) {
i++;
}
return i;
}
/**
* 双重匹配(采集使用)
* @param globalRegex 第一层匹配正则表达式
* @param singleRegex 内层匹配正则表达式
* @param content 需要截取的内容
* @return 返回Map数组 key:在系统中的数据编号 value:名称
*/
public static Map doubleRegex(String globalRegex,String singleRegex,String content){
Map map = new HashMap();
Pattern patternList = Pattern.compile(globalRegex);
Matcher matcherList = patternList.matcher(content);
while (matcherList.find()) {
Pattern _patternList = Pattern.compile(singleRegex);
Matcher _matcherList = _patternList.matcher(matcherList.group());
while (_matcherList.find()) {
if(!StringUtil.isBlank(_matcherList.group(1)) && !StringUtil.isBlank(_matcherList.group(2))){
map.put(_matcherList.group(1), _matcherList.group(2));
}
}
}
return map;
}
/**
* 双重匹配;自定义map数据的数量(采集使用)
* @param globalRegex 第一层匹配正则表达式
* @param singleRegex 内层匹配正则表达式
* @param content 需要截取的内容
* @param find 匹配值中的数量
* @return 返回Map数组 Map key:i value:名称
*/
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy