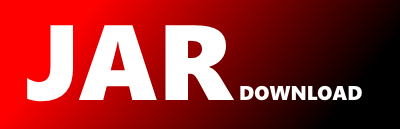
net.minidev.util.AutoCacher Maven / Gradle / Ivy
package net.minidev.util;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import net.minidev.util.CachedMapper.CacheType;
/**
* Buffered data getter
*
* automaticaly get value from cache and from external datasource
*
* @author Uriel Chemouni
*
* @param Key
* @param Value
*/
public class AutoCacher {
private CachedMapper cache;
private Loader loader;
public void clear() {
cache.clear();
}
public AutoCacher(CacheType type, long time, int size, Loader loader) {
cache = new CachedMapper(type, time, size);
this.loader = loader;
}
public V getFromCache(K key) {
return cache.get(key);
}
public void pushValuesToCache(Map map) {
for (Map.Entry ent : map.entrySet()) {
V value = ent.getValue();
cache.put(ent.getKey(), value);
}
}
public void pushValueToCache(K key, V value) {
cache.put(key, value);
}
/**
* get Data from Cache and missing data from datasource.
*/
public List getCachedList(Iterable keys, Object context) {
ArrayList toPreload = new ArrayList();
ArrayList result = new ArrayList();
for (K key : keys) {
V m = cache.get(key);
if (m != null)
result.add(m);
else
toPreload.add(key);
}
Map map = loader.load(toPreload, context);
pushValuesToCache(map);
result.addAll(map.values());
return result;
}
public Map getCachedMap(Iterable keys, Object context) {
HashMap result = new HashMap();
ArrayList toPreload = new ArrayList();
for (K key : keys) {
V m = cache.get(key);
if (m != null)
result.put(key, m);
else
toPreload.add(key);
}
Map map = loader.load(toPreload, context);
if (map.size() > 0) {
pushValuesToCache(map);
result.putAll(map);
}
return result;
}
public static interface Loader {
public Map load(Iterable keys, Object context);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy