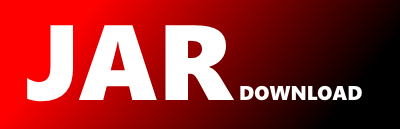
comparators.ShortComparator Maven / Gradle / Ivy
package net.mintern.primitive.comparators;
/**
* A comparison function that imposes total ordering on {@code short} values.
*
* This is a {@code @FunctionalInterface} even though it isn't declared as one,
* so that it can be used in Java 6+.
*/
public interface ShortComparator {
/**
* Compares {@code s1} and {@code s2}. Returns a negative value to indicate
* that {@code s1 < s2}, 0 to indicate that {@code s1 == s2}, and a positive
* value to indicate that {@code s1 > s2}.
*
* Implementations of this method must maintain the following invariants:
*
* - {@code s(compare(x, y)) == -s(compare(y, x))}
*
- {@code s(compare(x, y)) == s(compare(y, z))} →
* {@code s(compare(x, y)) == s(compare(x, z))} (transitivity)
*
- {@code compare(x, y) == 0} →
* {@code s(compare(x, z)) == s(compare(y, z))} ∀ {@code z}
*
*
* where {@code s(x)} is defined as follows:
*
* - {@code x < 0}: -1
*
- {@code x == 0}: 0
*
- {@code x > 0}: 1
*
*
* @param s1 the first short to compare
* @param s2 the second short to compare
* @return a negative value, 0, or a positive value to indicate that
* {@code s1} is less than, equal to, or greater than {@code s2},
* respectively
*/
int compare(short s1, short s2);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy