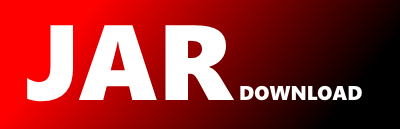
net.mintern.primitive.comparators.DoubleComparator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of primitive Show documentation
Show all versions of primitive Show documentation
Utility methods for primitive types
package net.mintern.primitive.comparators;
/**
* A comparison function that imposes total ordering on {@code double} values.
*
* This is a {@code @FunctionalInterface} even though it isn't declared as one,
* so that it can be used in Java 6+.
*/
public interface DoubleComparator {
/**
* Compares {@code d1} and {@code d2}. Returns a negative value to indicate
* that {@code d1 < d2}, 0 to indicate that {@code d1 == d2}, and a positive
* value to indicate that {@code d1 > d2}.
*
* Implementations of this method must maintain the following invariants:
*
* - {@code s(compare(x, y)) == -s(compare(y, x))}
*
- {@code s(compare(x, y)) == s(compare(y, z))} →
* {@code s(compare(x, y)) == s(compare(x, z))} (transitivity)
*
- {@code compare(x, y) == 0} →
* {@code s(compare(x, z)) == s(compare(y, z))} ∀ {@code z}
*
*
* where {@code s(x)} is defined as follows:
*
* - {@code x < 0}: -1
*
- {@code x == 0}: 0
*
- {@code x > 0}: 1
*
* Important Note
* A floating-point compare implementation must be careful to maintain
* total ordering, in particular when comparing -0.0, 0.0, @{code NaN},
* {@link Double#NEGATIVE_INFINITY}, and {@link Double#POSITIVE_INFINITY}
* values. See {@link Double#compareTo(Double)} for a valid example.
*
* @param d1 the first double to compare
* @param d2 the second double to compare
* @return a negative value, 0, or a positive value to indicate that
* {@code d1} is less than, equal to, or greater than {@code d2},
* respectively
*/
int compare(double d1, double d2);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy