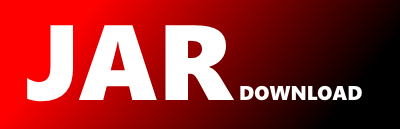
net.morher.ui.connect.html.HtmlMapper Maven / Gradle / Ivy
package net.morher.ui.connect.html;
import com.gargoylesoftware.htmlunit.html.HtmlElement;
import com.google.common.base.Function;
import com.google.common.collect.FluentIterable;
import java.util.Collection;
import net.morher.ui.connect.api.PassThroughLocator;
import net.morher.ui.connect.api.annotation.ElementName;
import net.morher.ui.connect.api.element.Screen;
import net.morher.ui.connect.api.mapping.ActionHandlerFactory;
import net.morher.ui.connect.api.mapping.ContextPassThroughActionHandlerFactory;
import net.morher.ui.connect.api.mapping.ElementLocater;
import net.morher.ui.connect.api.mapping.LocatorDescription;
import net.morher.ui.connect.api.mapping.UserInterfaceMapper;
public class HtmlMapper implements UserInterfaceMapper {
private static final ContextPassThroughActionHandlerFactory CONTEXT_PASS_THROUGH_ACTION_HANDLER_FACTORY = new ContextPassThroughActionHandlerFactory<>();
@Override
public ElementLocater buildLocator(LocatorDescription desc) {
CssSelector cssSelector = desc.findAnnotation(CssSelector.class);
if (cssSelector != null) {
return new HtmlSelectorLocator(cssSelector.value());
}
ElementName elementName = desc.findAnnotation(ElementName.class);
if (elementName != null) {
return new HtmlSelectorLocator("*[name=" + elementName.value() + "]");
}
if (Screen.class.isAssignableFrom(desc.getTargetClass())) {
return new PassThroughLocator<>();
}
return null;
}
@Override
public ActionHandlerFactory getActionHandlerFactory() {
return CONTEXT_PASS_THROUGH_ACTION_HANDLER_FACTORY;
}
public static class HtmlSelectorLocator implements ElementLocater {
private final String selectors;
public HtmlSelectorLocator(String selectors) {
this.selectors = selectors;
}
@Override
public Collection locate(HtmlElementContext parentContext) {
return FluentIterable
.from(parentContext.getElement().querySelectorAll(selectors))
.filter(HtmlElement.class)
.transform(new ElementToContextConverter(parentContext))
.toList();
}
}
public static class ElementToContextConverter implements Function {
private final HtmlElementContext parentContext;
public ElementToContextConverter(HtmlElementContext parentContext) {
this.parentContext = parentContext;
}
@Override
public HtmlElementContext apply(HtmlElement element) {
return new HtmlElementContext(parentContext, element);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy