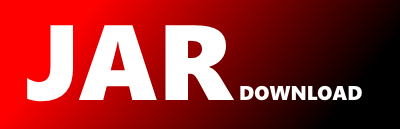
net.morher.ui.connect.http.Browser Maven / Gradle / Ivy
package net.morher.ui.connect.http;
import com.gargoylesoftware.htmlunit.BrowserVersion;
import com.gargoylesoftware.htmlunit.WebClient;
import com.gargoylesoftware.htmlunit.WebClientOptions;
import com.gargoylesoftware.htmlunit.html.HtmlElement;
import com.gargoylesoftware.htmlunit.html.HtmlPage;
import java.util.ArrayList;
import java.util.List;
import net.morher.ui.connect.api.Connector;
import net.morher.ui.connect.api.exception.ApplicationConnectionFailedException;
import net.morher.ui.connect.html.HtmlElementContext;
public class Browser implements BrowserConfigurer {
private final List configurers = new ArrayList<>();
private BrowserVersion version = BrowserVersion.getDefault();
private boolean throwExceptionOnFailingStatus = false;
private boolean followRedirect = true;
private boolean useInsecureSSL = false;
public static Connector atUrl(String url) {
return new Browser()
.openUrl(url);
}
public Browser() {
}
public Browser asChrome() {
this.version = BrowserVersion.CHROME;
return this;
}
public Browser throwExceptionOnFailingStatus() {
throwExceptionOnFailingStatus = true;
return this;
}
public Browser disableRedirects() {
followRedirect = false;
return this;
}
public Browser useInsecureSSL() {
useInsecureSSL = true;
return this;
}
public Browser addConfigurer(BrowserConfigurer configurer) {
configurers.add(configurer);
return this;
}
public Connector openUrl(String url) {
WebClient webClient = new WebClient(version);
try {
configure(webClient);
webClient.getPage(url);
return new BrowserConnector(webClient);
} catch (Exception e) {
webClient.close();
throw new ApplicationConnectionFailedException("Failed to connect to URL \"" + url + "\"", e);
}
}
@Override
public void configure(WebClient webClient) {
WebClientOptions options = webClient.getOptions();
options.setThrowExceptionOnFailingStatusCode(throwExceptionOnFailingStatus);
options.setRedirectEnabled(followRedirect);
options.setUseInsecureSSL(useInsecureSSL);
for (BrowserConfigurer configurer : configurers) {
configurer.configure(webClient);
}
}
private static class BrowserConnector implements Connector, AutoCloseable {
private final WebClient webClient;
public BrowserConnector(WebClient webClient) {
this.webClient = webClient;
}
public HtmlPage getPage() {
return (HtmlPage) webClient.getCurrentWindow().getEnclosedPage();
}
@Override
public HtmlElementContext getRootElement() {
return new HtmlRootContext(this);
}
@Override
public void close() throws Exception {
this.webClient.close();
}
}
private static class HtmlRootContext extends HtmlElementContext {
private final BrowserConnector browser;
public HtmlRootContext(BrowserConnector browser) {
super(null, null);
this.browser = browser;
}
@Override
public HtmlElement getElement() {
return browser.getPage().getDocumentElement();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy