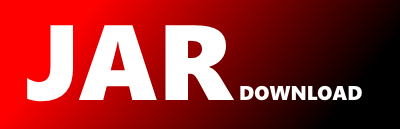
net.morimekta.providence.model.Decl Maven / Gradle / Ivy
package net.morimekta.providence.model;
/**
* Base declaration type. All declarations have these fields.
*/
@javax.annotation.Generated(
value = "net.morimekta.providence:providence-generator-java",
comments = "java:serializable")
@SuppressWarnings("unused")
public interface Decl {
/**
* Documentation for the specific declaration.
*
* @return The documentation value.
*/
String getDocumentation();
/**
* Documentation for the specific declaration.
*
* @return Optional documentation value.
*/
@javax.annotation.Nonnull
java.util.Optional optionalDocumentation();
/**
* @return If documentation is present.
*/
boolean hasDocumentation();
/**
* Name of the type, constant or service.
*
* @return The name value.
*/
@javax.annotation.Nonnull
String getName();
/**
* @return If name is present.
*/
boolean hasName();
enum _Field implements net.morimekta.providence.descriptor.PField {
DOCUMENTATION(-1, net.morimekta.providence.descriptor.PRequirement.OPTIONAL, "documentation", "documentation", net.morimekta.providence.descriptor.PPrimitive.STRING.provider(), null, null),
NAME(-2, net.morimekta.providence.descriptor.PRequirement.REQUIRED, "name", "name", net.morimekta.providence.descriptor.PPrimitive.STRING.provider(), null, null),
;
private final int mId;
private final net.morimekta.providence.descriptor.PRequirement mRequired;
private final String mName;
private final String mPojoName;
private final net.morimekta.providence.descriptor.PDescriptorProvider mTypeProvider;
private final net.morimekta.providence.descriptor.PStructDescriptorProvider mArgumentsProvider;
private final net.morimekta.providence.descriptor.PValueProvider> mDefaultValue;
_Field(int id, net.morimekta.providence.descriptor.PRequirement required, String name, String pojoName, net.morimekta.providence.descriptor.PDescriptorProvider typeProvider, net.morimekta.providence.descriptor.PStructDescriptorProvider argumentsProvider, net.morimekta.providence.descriptor.PValueProvider> defaultValue) {
mId = id;
mRequired = required;
mName = name;
mPojoName = pojoName;
mTypeProvider = typeProvider;
mArgumentsProvider = argumentsProvider;
mDefaultValue = defaultValue;
}
@Override
public int getId() { return mId; }
@javax.annotation.Nonnull
@Override
public net.morimekta.providence.descriptor.PRequirement getRequirement() { return mRequired; }
@javax.annotation.Nonnull
@Override
public net.morimekta.providence.descriptor.PDescriptor getDescriptor() { return mTypeProvider.descriptor(); }
@Override
@javax.annotation.Nullable
public net.morimekta.providence.descriptor.PStructDescriptor getArgumentsType() { return mArgumentsProvider == null ? null : mArgumentsProvider.descriptor(); }
@javax.annotation.Nonnull
@Override
public String getName() { return mName; }
@javax.annotation.Nonnull
@Override
public String getPojoName() { return mPojoName; }
@Override
public boolean hasDefaultValue() { return mDefaultValue != null; }
@Override
@javax.annotation.Nullable
public Object getDefaultValue() {
return hasDefaultValue() ? mDefaultValue.get() : null;
}
@Override
@javax.annotation.Nonnull
@SuppressWarnings("unchecked")
public net.morimekta.providence.descriptor.PMessageDescriptor onMessageType() {
return kDescriptor;
}
@Override
public String toString() {
return net.morimekta.providence.descriptor.PField.asString(this);
}
/**
* @param id Field name
* @return The identified field or null
*/
public static _Field findById(int id) {
switch (id) {
case -1: return _Field.DOCUMENTATION;
case -2: return _Field.NAME;
}
return null;
}
/**
* @param name Field name
* @return The named field or null
*/
public static _Field findByName(String name) {
if (name == null) return null;
switch (name) {
case "documentation": return _Field.DOCUMENTATION;
case "name": return _Field.NAME;
}
return null;
}
/**
* @param name Field POJO name
* @return The named field or null
*/
public static _Field findByPojoName(String name) {
if (name == null) return null;
switch (name) {
case "documentation": return _Field.DOCUMENTATION;
case "name": return _Field.NAME;
}
return null;
}
/**
* @param id Field name
* @return The identified field
* @throws IllegalArgumentException If no such field
*/
public static _Field fieldForId(int id) {
_Field field = findById(id);
if (field == null) {
throw new IllegalArgumentException("No such field id " + id + " in p_model.Decl");
}
return field;
}
/**
* @param name Field name
* @return The named field
* @throws IllegalArgumentException If no such field
*/
public static _Field fieldForName(String name) {
if (name == null) {
throw new IllegalArgumentException("Null name argument");
}
_Field field = findByName(name);
if (field == null) {
throw new IllegalArgumentException("No such field \"" + name + "\" in p_model.Decl");
}
return field;
}
/**
* @param name Field POJO name
* @return The named field
* @throws IllegalArgumentException If no such field
*/
public static _Field fieldForPojoName(String name) {
if (name == null) {
throw new IllegalArgumentException("Null name argument");
}
_Field field = findByPojoName(name);
if (field == null) {
throw new IllegalArgumentException("No such field \"" + name + "\" in p_model.Decl");
}
return field;
}
}
@SuppressWarnings("unchecked")
net.morimekta.providence.descriptor.PInterfaceDescriptor> kDescriptor =
new net.morimekta.providence.descriptor.PInterfaceDescriptor<>("p_model", "Decl", _Field.values(),
net.morimekta.providence.model.EnumValue.provider(),
net.morimekta.providence.model.EnumType.provider(),
net.morimekta.providence.model.TypedefType.provider(),
net.morimekta.providence.model.FieldType.provider(),
net.morimekta.providence.model.MessageType.provider(),
net.morimekta.providence.model.FunctionType.provider(),
net.morimekta.providence.model.ServiceType.provider(),
net.morimekta.providence.model.ConstType.provider(),
net.morimekta.providence.model.Declaration.provider());
interface _Builder extends Decl {
/**
* Set the documentation
field value.
*
* Documentation for the specific declaration.
*
* @param value The new value
* @return The builder
*/
@javax.annotation.Nonnull
public _Builder setDocumentation(String value);
/**
* Clear the documentation
field value.
*
* Documentation for the specific declaration.
*
* @return The builder
*/
@javax.annotation.Nonnull
public _Builder clearDocumentation();
/**
* Set the name
field value.
*
* Name of the type, constant or service.
*
* @param value The new value
* @return The builder
*/
@javax.annotation.Nonnull
public _Builder setName(String value);
/**
* Clear the name
field value.
*
* Name of the type, constant or service.
*
* @return The builder
*/
@javax.annotation.Nonnull
public _Builder clearName();
/**
* @return The built instance
*/
@javax.annotation.Nonnull
Decl build();
}
}