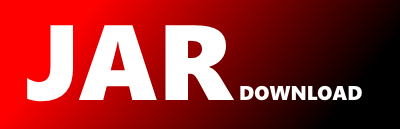
tests.test.thrift Maven / Gradle / Ivy
namespace java net.morimekta.test.providence
enum Value {
FIRST = 1,
SECOND = 2,
THIRD = 3,
FOURTH = 5,
FIFTH = 8,
SIXTH = 13,
SEVENTH = 21,
EIGHTH = 34,
NINTH = 55,
TENTH = 89,
ELEVENTH = 144,
TWELWETH = 233,
/** @Deprecated */
THIRTEENTH = 377,
FOURTEENTH = 610,
FIFTEENTH = 987,
SIXTEENTH = 1597,
SEVENTEENTH = 2584,
EIGHTEENTH = 4181,
NINTEENTH = 6765,
TWENTIETH = 10946
}
/** @compact */
struct CompactFields {
1: required string name
2: required i32 id,
3: string label;
}
/*
const list kDefaultCompactFields = [
{"name": "Tut-Ankh-Amon", "id": 1333, "label": "dead"},
{"name": "Ramses II", "id": 1279}
];
*/
struct OptionalFields {
1: optional bool booleanValue;
2: optional byte byteValue,
3: optional i16 shortValue
4: optional i32 integerValue;
5: optional i64 longValue,
6: optional double doubleValue
7: optional string stringValue;
8: optional binary binaryValue,
9: optional Value enumValue;
10: optional CompactFields compactValue;
}
struct RequiredFields {
1: required bool booleanValue;
2: required byte byteValue,
3: required i16 shortValue
4: required i32 integerValue;
5: required i64 longValue,
6: required double doubleValue
7: required string stringValue;
8: required binary binaryValue,
9: required Value enumValue;
10: required CompactFields compactValue;
}
struct DefaultFields {
1: bool booleanValue;
2: byte byteValue,
3: i16 shortValue
4: i32 integerValue;
5: i64 longValue,
6: double doubleValue
7: string stringValue;
8: binary binaryValue,
9: Value enumValue;
10: CompactFields compactValue;
}
union UnionFields {
1: bool booleanValue;
2: byte byteValue,
3: i16 shortValue
4: i32 integerValue;
5: i64 longValue,
6: double doubleValue
7: string stringValue;
8: binary binaryValue,
9: Value enumValue;
10: CompactFields compactValue;
}
exception ExceptionFields {
1: bool booleanValue;
2: byte byteValue,
3: i16 shortValue
4: i32 integerValue;
5: i64 longValue,
6: double doubleValue
7: string stringValue;
8: binary binaryValue,
9: Value enumValue;
10: CompactFields compactValue;
}
typedef double real
struct DefaultValues {
1: bool booleanValue = true;
2: byte byteValue = -125,
3: i16 shortValue = 13579
4: i32 integerValue = 1234567890;
5: i64 longValue = 1234567891,
6: real doubleValue = 2.99792458e+8
7: string stringValue = "test\\twith escapes\\nand\\u00a0ũñı©ôðé.";
8: binary binaryValue;
9: Value enumValue = Value.SECOND;
10: CompactFields compactValue
}
struct Containers {
// all types as list.
1: optional list booleanList;
2: optional list byteList;
3: optional list shortList;
4: optional list integerList;
5: optional list longList;
6: optional list doubleList;
7: optional list stringList;
8: optional list binaryList;
// all types as set.
11: optional set booleanSet;
12: optional set byteSet;
13: optional set shortSet;
14: optional set integerSet;
15: optional set longSet;
16: optional set doubleSet;
17: optional set stringSet;
18: optional set binarySet;
// all types as map.
21: optional map booleanMap;
22: optional map byteMap;
23: optional map shortMap;
24: optional map integerMap;
25: optional map longMap;
26: optional map doubleMap;
27: optional map stringMap;
28: optional map binaryMap;
// Using enum as key and value in containers.
31: optional list enumList;
32: optional set enumSet;
33: optional map enumMap;
// Using struct as key and value in containers.
41: optional list messageList;
42: optional set messageSet;
43: optional map messageMap;
51: optional RequiredFields requiredFields;
52: optional DefaultFields defaultFields;
53: optional OptionalFields optionalFields;
54: optional UnionFields unionFields;
55: optional ExceptionFields exceptionFields;
56: optional DefaultValues defaultValues;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy