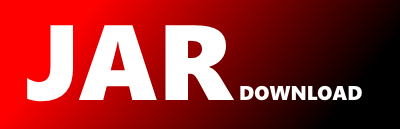
net.morimekta.tiny.server.config.ConfigSupplier Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tiny-server Show documentation
Show all versions of tiny-server Show documentation
A tiny server starter for easy and minimal microservice handling. This is based on the
assumption that the microservice does not use the admin HTTP server for anything but
kubernetes status management.
Dependencies are kept at a minimum, but should cover the basic needs of any microservice.
package net.morimekta.tiny.server.config;
import com.fasterxml.jackson.databind.ObjectMapper;
import net.morimekta.file.FileUtil;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.UncheckedIOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.Optional;
import java.util.concurrent.atomic.AtomicReference;
import java.util.function.Consumer;
import java.util.function.Supplier;
import static java.util.Objects.requireNonNull;
/**
* A wrapper around a config file to handle loading and parsing during
* application setup.
*
* {@code
* class MyApplication extends TinyApplication {
* private var config = ConfigSupplier.yamlConfig(MyConfig.class)
* {@literal@}Override
* public void initialize(ArgParser argParser, TinyApplicationContext.Builder context) {
* argParser.add(Option
* .optionLong("--config", "A config file", ValueParser.path(config::loadFromFile))
* .required())
* }
*
* public void onStart(TinyApplicationContext context) {
* var myConfig = config.get();
* // you have a config!
* }
* }
* }
*
* @param The config type.
*/
public class ConfigSupplier implements Supplier {
private final AtomicReference reference;
private final ConfigReader loader;
public ConfigSupplier(ConfigReader loader) {
this.reference = new AtomicReference<>();
this.loader = loader;
}
public void loadUnchecked(Path filePath) {
try {
load(filePath);
} catch (IOException e) {
throw new UncheckedIOException(e.getMessage(), e);
}
}
public void load(Path filePath) throws IOException {
var canonical = FileUtil.readCanonicalPath(filePath);
if (!Files.exists(canonical)) {
throw new FileNotFoundException("No such config file " + filePath);
}
if (!Files.isRegularFile(canonical)) {
throw new IOException("Config path " + filePath + " is not a regular file.");
}
reference.set(requireNonNull(loader.readConfig(filePath), "loaded config == null"));
}
@Override
public ConfigType get() {
return Optional.of(reference.get()).orElseThrow(() -> new NullPointerException("Config not loaded."));
}
public static ConfigSupplier yamlConfig(Class type) {
return yamlConfig(type, mapper -> {});
}
public static ConfigSupplier yamlConfig(Class type,
Consumer initMapper) {
return new ConfigSupplier<>(new ConfigReader.YamlConfigReader<>(type, initMapper));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy